Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this problem, you will draw a series of memory diagrams that illustrate the execution of a program that operates on objects of the










In this problem, you will draw a series of memory diagrams that illustrate the execution of a program that operates on objects of the ArrayBag class from lecture. Consider the following Java program: public class ArrayBagTest { public static void main(String[] args) { ArrayBag b1 = new ArrayBag(3); ArrayBag b2 = new ArrayBag(3); ArrayBag b3 = b2; = // part 1: what do things look like when we get here? // part 2: what do things look like at the start of // this method call? bl.add("hello"); b2.add("world"); b3.add("hello"); // part 3: what do things look like when we get here? System.out.println(b1); System.out.println(b2); System.out.println(b3); 1. In section 3-1 of ps4_partI, we have given you the beginnings of a memory diagram. On the stack (the region of memory where local variables are stored), we have included a frame for the main method. On the heap (the region of memory where objects are stored), we have included the ArrayBag object to which the variable b1 refers and its items array. As usual, we have used arrows to represent the necessary references. Complete the provided memory diagram so that it shows what things look like in memory just before the call b1.add("hello"). To do so, you should: Click on the diagram and then click the Edit link that appears below the diagram. Make whatever changes are needed to the diagram. Below the thick horizontal line, we have given you a set of extra components that you can use as needed by dragging them above the thick line and putting them in the correct position. These components include String objects representing the strings "hello" and "world". You may not need all of the provided components. You can also edit any of the values in a field or array by clicking on the corresponding cell and editing the text that is inside the cell. Once you have made all of the necessary changes, click the Save & Close button. 2. In section 3-2 of ps4_partI, we have given you the beginnings of a memory diagram that you should complete to show what things look like in memory at the start of the execution of b1.add("hello")-just before the first statement of that method is executed. Note: To save time, it should be possible to select and copy some of the components of your diagram for 3-1 and paste them into your diagram for 3-2. 3. In section 3-3 of ps4_partI, we have given you the beginnings of a memory diagram that you should complete to show what things look like in memory after all of the calls to add have returned-just before the print statements execute in main(). Note: We have included a method frame for add, but it may or not be needed in this diagram; you should delete it if you determine that it would no longer be present in memory. 4. What would be printed by this program? You may find it helpful to consult the toString method of the ArrayBag class. import java.util.*; public class ArrayBag { /** * The array used to store the items in the bag. */ private Object[] items; /** * The number of items in the bag. */ private int numItems; public static final int DEFAULT_MAX_SIZE 50; /** * Constructor with no parameters creates a new, empty ArrayBag with *the default maximum size. */ public ArrayBag() { this.items = new Object [DEFAULT_MAX_SIZE]; this.numItems = 0; } /** * A constructor that creates a new, empty ArrayBag with the specified * maximum size. */ public ArrayBag(int maxSize) { if (maxSize } } else if (this.numItems == this.items.length) { return false; // no more room! } else { /** * remove removes one occurrence of the specified item (if any) * from this ArrayBag. Returns true on success and false if the * specified item (i.e., an object equal to item) is not in this ArrayBag. */ } public boolean remove (Object item) { for (int i = 0; i < this.numItems; i++) { if (this.items[i].equals(item)) { /** } this.items [this.numItems] this.numItems++; return true; } // Shift the remaining items left by one. for (int j i; j < this.numItems 1; j++) { this.items[j] this.items[j + 1]; this.items [this.numItems 1] = null; } this.numItems --; return false; // item not found /** * contains returns true if the specified item is in the Bag, and * false otherwise. */ return true; public boolean contains (Object item) { } } item; for (int i = 0; i < this.numItems; i++) { if (this.items[i].equals(item)) { = return true; return false; * grab */ public Object grab() { returns a reference to a randomly chosen item in this ArrayBag. if (this.numItems == = 0) { throw new IllegalStateException ("the bag is empty"); } /** * toArray return an array containing the current contents of the bag */ int whichOne = (int) (Math.random() * this.numItems); return this.items [whichOne]; public Object[] toArray() { Object[] copy = new Object[this.numItems ] ; } for (int i = 0; i < this.numItems; i++) { copy[i] = this.items [i]; } } return copy; /** * toString converts this ArrayBag into a string that can be printed. * Overrides the version of this method inherited from the Object class. */ public String toString() { String str = "{"; for (int i = 0; i < this.numItems; i++) { str = str + this.items[i]; if (i != this.numItems 1) { } 11 str + " " ; } str = str + "}"; return str; /* Test the ArrayBag implementation. */ public static void main(String[] args) { // Create a Scanner object for user input. Scanner scan = new Scanner(System.in); // Create an ArrayBag named bagl. System.out.print("size of bag 1: "); int size= scan.nextInt (); ArrayBag bagl scan.nextLine(); new ArrayBag (size); = // consume the rest of the line add them to bagl, and print out bagl. // Read in strings, String itemStr; for (int i = 0; i < size; i++) { System.out.print("item +i+ ': "); itemStr = scan.nextLine(); bagl.add(itemStr); } } = System.out.println("bag 1 System.out.println(); // Select a random item and print it. Object item = bagl.grab(); System.out.println("grabbed System.out.println(); + item); // Iterate over the objects in bagl, printing them one per // line. Object[] items = bagl.toArray(); for (int i = 0; i < items.length; i++) { System.out.println(items[i]); } + bagl); System.out.println(); // Get an item to remove from bagl, remove it, and reprint the bag. System.out.print("item to remove: "); itemStr = scan.nextLine(); if (bagl.contains (itemStr)) { } bagl.remove(itemStr); System.out.println("bag 1 = System.out.println(); + bagl); Problem 3: Memory management and the ArrayBag class 3-1) Stack main b1 b2 b3 items numltems 0 items numltems items numltems 0 0 items numltems Heap 0 null null null null null null null null null null null null null null null "hello" "hello" "world" "world" 3-2) Stack add this item main b1 b2 b3 items numltems 0 items numltems items numltems 0 0 items Heap numltems 0 null null null null null null null null null null null null null null null "hello" "hello" "world" "world" 3-3) Stack add this item 3-4) main b1 b2 b3 items numltems 0 items numltems items numltems 0 0 items Heap numltems 0 null null null null null null null null null null null null null null null "hello" "hello" "world" "world"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
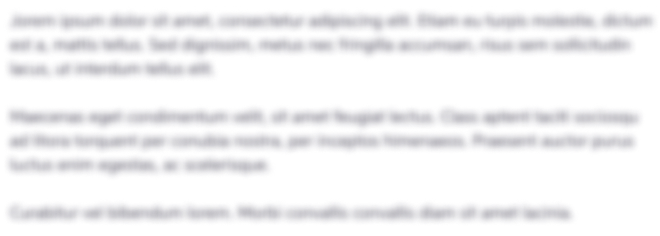
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started