Question
In this programming assignment you will practice using ArrayLists, Inheritance and Polymorphism. You are to mention game called Magical Creatures War. make a class called
In this programming assignment you will practice using ArrayLists, Inheritance and Polymorphism. You are to mention game called Magical Creatures War. make a class called MagicalCreature that can be used to mention magical creature that is defined by a name, type, color and age. All of these characteristics are of String type except age which is an integer. There is one attribute that keeps track of whether the creature is alive or dead. Use a Boolean variable called alive to store this information. There are four types of magical creatures: Dragon, Elf, Genie and Goblin. Write four derived classes for each of these creatures using the tables given below: A main program for the game using the rules given. MagicalCreature class should have the following public methods. It should be saved in a file called MagicalCreatures.java Method Description MagicalCreature(String name, String type, String color, int age) Constructor that sets the attribute values as received in the parameters String getName() String getType() String getColor() int getAge() boolean getAlive() Getters, one for each of the attributes void setName(String name) void setType(String type) void setColor(String color) void setAge(int age) void setAlive(boolean alive) Setters, one for each of the attributes If alive is true, then the creature is alive otherwise it is dead. You can cause a live creature to die by setting alive to false. void kill() Prints the string "I do not have the license to kill "); void die() Sets the attribute alive to false. This causes the creature to "die" String toString() Returns a string "My name is " + name +" I am a "+color+" " +type+" I am "+age +" years old ";
Create the classes for the four derived types using the tables given below. Note that all attributes will have private access and all instance methods will be public. Keep the following game rules in mind: Rules of the game: Dragons cannot be killed. A young dragon who is under 40 years old cannot kill Elves and Goblins cannot kill Elves can have a shield. Those with a shield cannot be killed Genies can have wands. Only Genies that have a wand can kill Creatures cannot kill themselves Class Dragon This class has no attributes. It is a sub class of MagicalCreatures. Save in Dragon.java Method Description Dragon(String name, String type, String color, int age) Constructor. Calls the constructor of the parent class. String toString() Returns the MagicalCreatures toString information plus " I breathe fire ". void kill(MagicalCreature other) Overrides the MagicalCreature method. If it's a younger dragon, prints "I am too young to kill !". Otherwise kills the other dragon by causing it to die void die() Overrides the MagicalCreature method. Prints "I am a dragon - nobody gets to kill me !" Class Elf Method Description This class is a sub class of MagicalCreature and has an attribute of boolean type called shield. Save class in file Elf.java Elf(String name, String type, String color, int age) Constructor. Calls the parent class' constructor. Initializes shield to false
String toString() Returns the MagicalCreatures toString information plus " I eat leaves, I have "+ a +"shield"; or " I eat leaves, I have "+ no +"shield"; depending on whether it has a shield or not. void setShield(boolean shield) Sets the shield attribute accordingly Class Goblin Method Description This class is a sub class of MagicalCreature. Save class in file Goblin.java Goblin(String name, String type, String color, int age) Constructor. Calls the parent class' constructor. String toString() Returns the MagicalCreatures toString information plus " I kill elves if they do not have shields "; . Class Genie Method Description This class has an attribute of boolean type called wand. Save class in file Genie.java Genie(String name, String type, String color, int age) Constructor. Calls the parent class' constructor. Initializes wand to false String toString() Returns the MagicalCreatures toString information plus " I eat leaves, I have "+ a +"wand"; or " I eat leaves, I have "+ no +"wand"; depending on whether it has a wandor not. void setWand(boolean wand) boolean getWand() Sets the wand attribute accordingly
void kill(MagicalCreature other) If it has a wand, it causes the other creature to die (if the other is not a Genie). If it does not have a wand, it prints "I cannot kill without my wand !" In addition to the above, make client or driver program called MagicalCreaturesGame.java that allows the user to play a war game as follows: Read the creatures information from a text file called creatures.txt. Each creature occupies one line in the data file and contains name, type, color and age. make and populate an ArrayList of MagicalCreatures by processing one line at a time of the data file. The line of text is to be split using the split method into an array of String containing four String tokens representing the four attributes. The war game consists of moves. The number of moves is decided by the user. Each move consists of an attack by one magical creature on another. The attacker and victim both need to adhere to the rules of the game which will decide if the attacker is eligible to kill or the victim is a candidate that can die. In each move, the selection of the attacker and victim is done randomly using the Random class. After each move, dead creatures are removed from the game, (from the Arraylist) and the list of creatures that are still alive is printed. Review of Inheritance and Polymorphism and other java classes Make sure to review the Random Class, Character class, Integer class (parseInt method for converting String to integer) ArrayList methods. Read the chapter on Inheritance and Polymorphism to understand how overriding works. Make note of declaring objects of super class make and actually constructing or creating them of the sub class type. You will also need to use the instanceof method to determine the class of an object while dealing with functions that use polymorphism. Casting of objects is another feature that may be necessary Documentation and Testing In terms of correctness, your class must provide all of the functionality described above and must satisfy all of the constraints mentioned in this writeup. In terms of style, we will be grading on your use of comments, good variable names, consistent indentation, minimal data fields and good coding style to implement these operations. You must use good documentation including Javadoc comments and tags as discussed in class. You welcome to research sources like https://www.geeksforgeeks.org/what-is-javadoc-tool-and-how-to-use-it/.You must include exactly what type of exception is thrown if a precondition is violated. Remember to mention all important behavior that a client would want to know about. Test your program by running your driver multiple times with different number of moves and giving or refusing shields to elves and wands to genies. Since a random number is used your output may not exactly match the
output given in this document. Run your program initially with number of moves =1, and gradually increase the number and the options for shield and wand. Test for number of moves =20. Take a screen shot of your output for number of moves =20, 10, 5, 1. Vary the shield and wand options. Submission 1. all your class files (MagicalCreature.java, Elf.java, Dragon.java, Goblin.java and Genie.java) 2. your client programs (MagicalCreatureGame.java and MagicalCreatureHelper.java) 3. a document with the output of testing. Call this document Assignment2Test.doc. Development Strategy One of the most important techniques for software professionals is to develop code in stages rather than trying to write it all at once (the technical term is iterative enhancement or stepwise refinement). It is also important to be able to test the correctness of your solution at each different stage. Use this execution chart for reference Execution Chart of the Driver. Main MagicalCreaturesHelper.fillData(c); // is the arraylist of creatures processLine(line); // this method reads a line from the data file, creates and returns a magical creature MagicalCreaturesHelper.playGame(c); // asks the user for number of moves and calls war method that many times war(c,in); // inputs the creature arraylist and scanner and randomly // selects a killer and victim. If the killer is a genie asks // wand option, if victim is elf asks shield option. Then // makes the killing move by invoking the kill method displayCreatures(c); // removes dead creatures from the arraylist and prints // the remaining creatures by using toString method We are suggesting that you develop the program in multiple stages: Put all methods in helper file called MagicalCreaturesHelper.java. 1. In this stage we want to test constructing a MagicalCreature class and examining it's contents. So the methods we will implement are the constructor, the getters and setters and the toString method. mention simple test program to make magical creature and test its methods.
2. Now in your test program, practice creating an arraylist of magical creatures by hard coding in your program, declaration and construction of 3 or 4 magical creatures. Print the contents of this database and add and remove creatures from it. 3. Create one creature class, like Goblin. In your main program, practice creating goblins. You can use the data file given to you. Save a copy and make changes as needed to it for testing your program in a step wise manner. Change all types to Goblins in the data file and use it to make an arraylist of goblins. Implement the filldatabase method and the processLine method that it calls 4. Add another class like Dragon. Change your testing file as needed. Start working on the game and write the war method. 5. Add the rest of the classes and complete the rest of the implementation. 6. Test your program by using the original creatures.txt file given to you. Expected Output When you run your program, test it for moves =1, 5,10, 20. Sample interactions are provided in the file Assigment2_SampleOutput.txt given to you. Your output may not exactly match the given one, due to
Step by Step Solution
There are 3 Steps involved in it
Step: 1
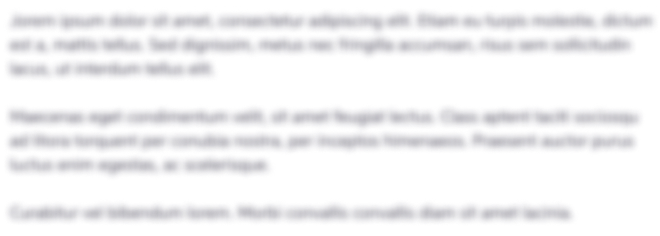
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started