Question
In this project we're are going to create an application that calculates the surface area and volume of 1 cylinder using two classes: a base
In this project we're are going to create an application that calculates the surface area and volume of 1 cylinder using two classes: a base class and a derived class. The classes are:
Circle (Base class)
Cylinder (Derived class)
Classes
Create the following classes.
Circle |
-radius: float |
+setRadius(float r): void +getRadius() const: float +getArea() const: float +Circle(float r = 0) |
Business rules
radius value must be zero or greater.
getArea function calculates and returns the area of the circle.
Cylinder |
-height: float |
+setHeight(float h): void +getHeight() const: float +getArea() const: float +getVolume() const: float +Cylinder(float r = 0, float h = 0) |
Cylinder is a derived class of Circle. Use the public access specifier for the inheritance i.e. Cylinder : public Circle
getArea function will override the Circle's getArea function. This function will return the surface area of the cylinder.
getVolume function calculates and returns the volume of the circle.
Business rules
radius value must be zero or greater. height must be zero or greater.
In the main
Create a Cylinder object.
Ask for radius and height for the Cylinder object. Then, calculate and display the cylinder's surface area and volume.
Files to submit
Solution/project related files.
Circle class (.h and .cpp)
Cylinder class (.h and .cpp)
Main program (.cpp)
Submit your project in a zip folder.
Example of expected output
Cylinder App! -------------------- Enter radius: 10 Enter height: 5 Cylinder area: 942.477 Cylinder volume: 1570.79
Step by Step Solution
There are 3 Steps involved in it
Step: 1
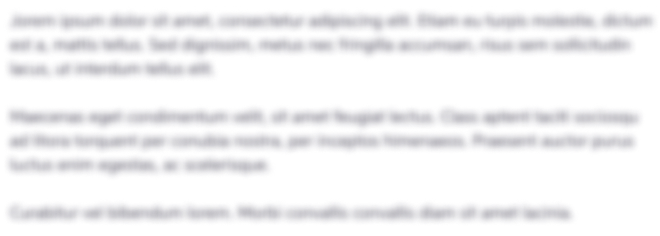
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started