Question
In this project, you need to implement two classes: Player, in the file player.py, which encapsulates a (soccer) player. One of the public instance variables
In this project, you need to implement two classes:
Player, in the file player.py, which encapsulates a (soccer) player. One of the public instance variables in Player is goals which stores the number of goals that the player has scored.
Team, in the file team.py, which encapsulates a soccer (team). By inspecting the main program below and the corresponding output, you should be able to figure out which variables and methods the two classes need
/ Main program:
import random
from player import Player
from team import Team
def main():
random.seed(10)
player1 = Player("Mohamed", "Salah")
player2 = Player("Roberto", "Firmino")
player3 = Player("Luis", "Daz")
player4 = Player("Marcus", "Rashford")
player5 = Player("Harry", "Maguire")
player6 = Player("Christiano", "Ronaldo")
players = [player1, player2, player3, player4, player5, player6]
for player in players:
goals = random.randint(1,5)
player.add_goals(goals)
print(player)
team1 = Team("Liverpool")
team1.add_player(player1)
team1.add_player(player2)
team1.add_player(player3)
print(team1)
team2 = Team("Manchester United")
team2.add_player(player4)
team2.add_player(player5)
team2.add_player(player6)
print(team2)
most_goals_player = team1.most_goals_player()
print(most_goals_player)
most_goals_player = team2.most_goals_player()
print(most_goals_player)
team3 = team1 + team2
print(team3)
most_goals_player = team3.most_goals_player()
print(most_goals_player)
if __name__ == "__main__":
main()
InfoWarningTip
Note that when a team is printed out its players are shown in descending order on the number of goals they have scored. If two or more players have scored equal number of goals, the one that was added first to the team is shown first. One tab-character is printed in each line before the information for each player is shown
InfoWarningTip
Let us assume that players is some kind of a collection of instances of the Player class. In order to sort players in descending order on goals scored, you can use: sorted(players, key=lambda p: p.goals, reverse=True) Here, "lambda p: p.goals" is an anonymous function which returns the value of the goals variable in the instance
Step by Step Solution
There are 3 Steps involved in it
Step: 1
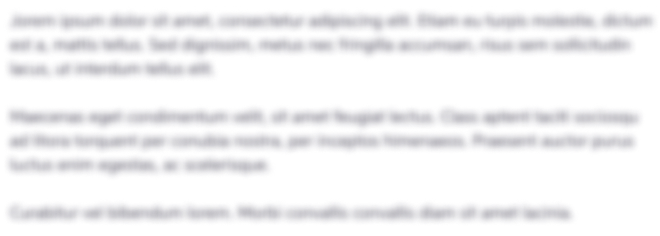
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started