Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this project, you will: - Complete the code to solve a maze - Discuss related data structures topics Programming - - - - -
In this project, you will:
Complete the code to solve a maze
Discuss related data structures topics
Programming
from arraystack import ArrayStack
from grid import Grid
BARRIER # barrier
FINISH F # finish goal
OPEN O # open step
START S # start step
VISITED # # visited step
def main:
maze getMaze
print
The maze:"
printMazemaze
startRow startCol findStartPositionmaze
if startRow startCol:
printThis maze does not have a start symbol.
return
success solveMazestartRow startCol, maze
if success:
printMaze solution:"
printMazemaze
else:
printThere is no solution for this maze.
def getMaze:
Reads the maze from a text file and returns a grid that represents it
name inputEnter a file name for the maze:
fileObj opennamer
firstLine listmapint fileObj.readlinestripsplit
rows firstLine
columns firstLine
maze Gridrows columns
for row in rangerows:
line fileObj.readlinestrip
column
for character in line:
mazerowcolumn character
column
return maze
# Returns a tuple containing the row and column position of the start symbol.
# If there is no start symbol, returns the tuple
def findStartPositionmaze:
# Part :
#
# Prints the maze with no spaces between cells.
def printMazemaze:
# Part :
#
# rowcolumn is the position of the start symbol in the maze.
# Returns True if the maze can be solved or False otherwise.
def solveMazestartRow startColumn, maze:
# The exploration stack contains tuples of row column coordinates of
# grid cells on the frontier of the maze solution exploration.
exploreStack ArrayStack
exploreStack.pushstartRow startColumn
while not exploreStack.isEmpty:
row column exploreStack.pop
if mazerowcolumn FINISH:
# Restore start cell to its original label:
mazestartRowstartColumn START
removeDeadEndsmaze
return True
if mazerowcolumn VISITED:
continue
# Cell has not been visited.
# Mark it as visited.
mazerowcolumn VISITED
# Push adjacent unvisited positions onto the stack:
# Part :
#
return False
# Changes visited, dead end cells to be open.
def removeDeadEndsmaze:
while True:
deadEndCount
for row in rangemazegetHeight:
for column in rangemazegetWidth:
if isDeadEndrow column, maze:
mazerowcolumn OPEN
deadEndCount
if deadEndCount :
return
# A dead end cell is a visited cell that:
# is not the start cell
# is not the finish cell
# has less than visitedstartfinish compass direction NSEW neighbor cells
def isDeadEndrow column, maze:
if mazerowcolumn VISITED:
return False
if mazerowcolumn START:
return False
if mazerowcolumn FINISH:
return False
numberOfVisitedNeighbors
# Check north:
if row and mazerow column in VISITED START, FINISH:
numberOfVisitedNeighbors
# Check south:
if row maze.getHeight and mazerow column in VISITED START, FINISH:
numberOfVisitedNeighbors
# Check east:
if column maze.getWidth and mazerowcolumn in VISITED START, FINISH:
numberOfVisitedNeighbors
# Check west:
if column and mazerowcolumn in VISITED START, FINISH:
numberOfVisitedNeighbors
if numberOfVisitedNeighbors :
return True
return False
main
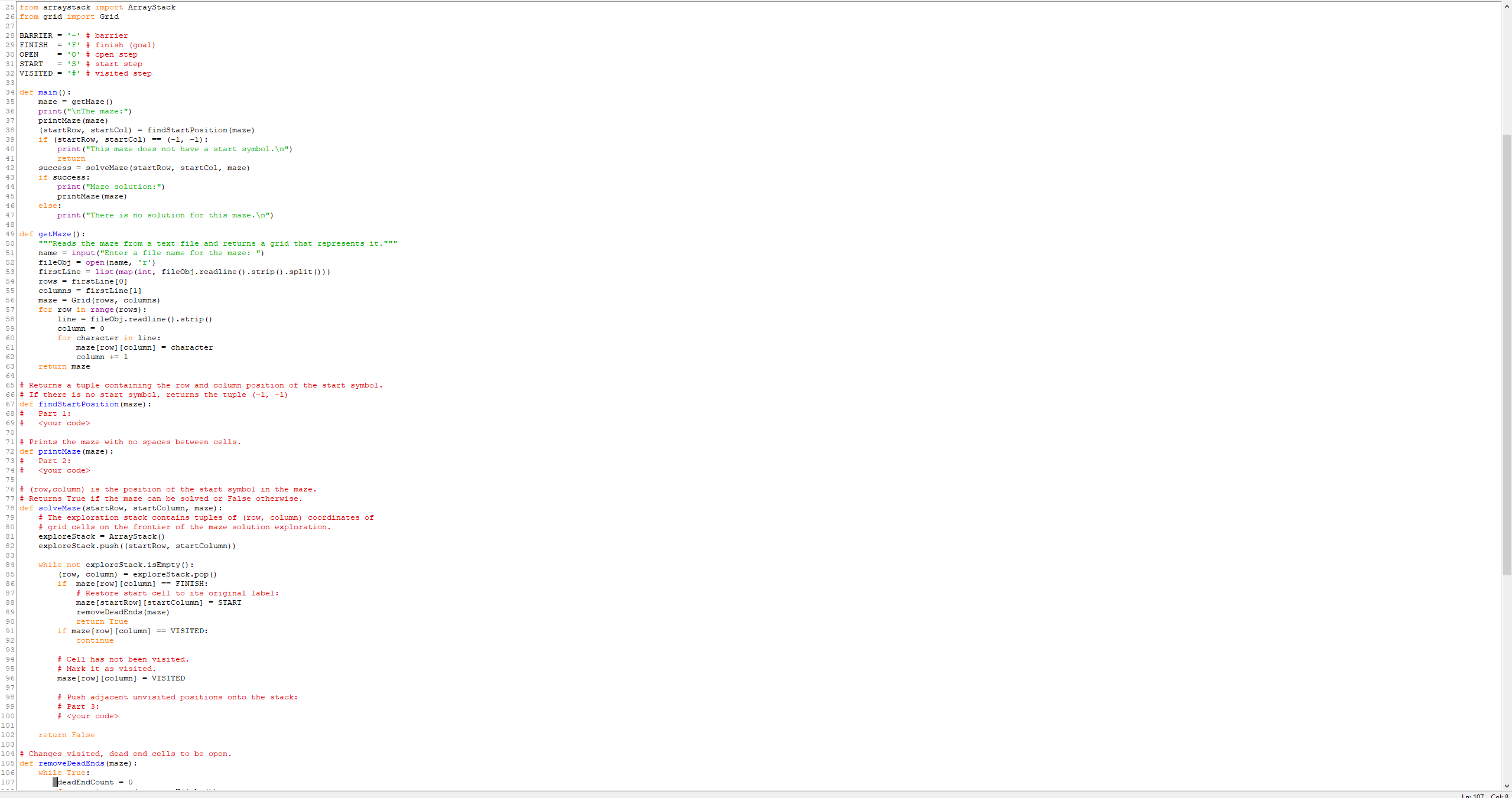
Step by Step Solution
There are 3 Steps involved in it
Step: 1
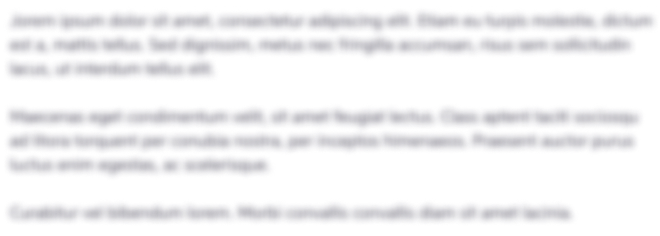
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started