Question
In which data structure element is inserted at one end called Rear? a. Stack b. Queue c. Both d. None Q2. Which method is called
In which data structure element is inserted at one end called Rear?
a. Stack b. Queue c. Both d. None
Q2. Which method is called in a push() method?
a. IsFull() b. IsEmpty() c. Both d. None
Q3. What method is used to add an element to a Stack?
a. dequeue() b. enqueue() c. push() d. pop()
Q4. Queue is called
a. LIFO b. FIFO c. Both d. None
Q5. Index values of an array range from ______________.
-
1 to length
-
0 to length + 1
-
0 to length 1
-
0 to length
Q6. Which of the following creates an array of int named alpha?
-
int alpha = new alpha[10];;
-
int [] alpha = int[10];
-
int alpha = new int alpha[10];
-
int [] alpha = new int[10];
(10 points)
Q7. What are the legal indexes for the array xy, given the following declaration: int [] xy = {5, 2, 3, 7};
a. 0,1,2,3 b. 0,2,4.6 c. 1,2,3,4 d. 5,2,3,7
Q8. What happens when you compile and run this program? int[] a = {7, 9, 13, 21, 35, 48}; System.out.println(a[6]);
-
48 will be printed
-
6 will be printed
-
ArrayIndexOutOfBoundsException occurs
-
None of the above
Q9. String[][] name = new String[9][7]; What is the value of name.length?
a. 0 b. 7 c. 9 d. 16
Q10. How many columns does abc have if it is created as follows int [][] abc = {{2, 6, 8}, {1, 2},{5}};?
a. 0 b. 1 c. 2 d. 3
2/6
Section 2
(10 points)
Q11. Fill in the four blanks of the following code fragment so that the elements of the array are printed in reverse order. (3 points)
int[] array = { 2, 4, 6, 8, 10 }; for ( __________________ ;__________________ ; ___________ )
System.out.print(_________________+ " " );
public static int mysteryArray (int list[], int x)
Q13. Consider changeArray method. An array is created that contains {1, 3, 5, 2} and is passed to changeArray. What are the contents of the array after the changeArray method executes? Show all steps. (4 points)
public static void changeArray(int[] data){ for (int i = 1; i < data.length -2 ; i++)
data[i] = data[i - 1] + data[i + 1]; }
Q12. Given the following method heading, write a complete code that calls the method mysteryArray. (3 points)
i values | Array new values |
3/6
Section 3 (10 points) Find the output for the below code fragments. Show your work.
Q14. (3 points) int[] arrX = {3, 5, 0, 1, 6, 4, 2}; int[] arrY = {2, 6, 5, 4, 3, 1, 0}; int[] arrZ = {6, 9, 4, 0, 5, 3, 2};
System.out.print(arrZ[arrX[arrY[arrZ[2]]]]);
Q15. (3 points) int[] list = {2, 4, 6, 8 }; int count = 0, item = 5; for (int j = 0; j < list.length; j++)
if (list[j] == item) count++;
System.out.print(count);
Q16. (2 points) double[][] values = { {1.2, 9.0, 3.2},
{9.2, 0.5, 1.5, 1.3},
{7.3, 7.9, 4.8} } ; System.out.print(values[1][3]);
Q17. (2 points)
int[] xy = { 2, 4, 6, 8, 10, 1, 3, 5, 7, 9 }; for ( int index = 0 ; index < xy.length ; index += 3)
System.out.print( xy[ index ] + " " );
4/6
Section 4
(10 points)
public class Stack {
private int maxSize; private int[] stackArray; private int top;
public Stack(int s) { maxSize = s;
stackArray = new int[maxSize]; top = -1;
} Based on the above class declaration, write the following methods. Q18. Write isEmpty (boolean method) that checks if the stack is empty or not. (3 points)
Q19. Write isFull (boolean method) that checks if the stack is full or not. (3 points)
Q20. Write push (void method) that add a new element on top of stack. (4 points)
5/6
Section 5
(10 points)
class Queue { private int front, rear, maxSize;
private int queue[];
Queue(int c) { front = 0; rear = -1;
maxSize = c;
queue = new int[maxSize]; }
Based on the above class declaration, write the following methods.
Q21. Write dequeue (void method) that checks if the queue is not empty then removes the element on front.
(4 points)
Q22. Write splitQ method that splits the elements of a queue (q1) in two by assigning first half values to a new queue (q2) and keeping only last half values in (q1). (6 points)
Example:
q1 holds 20 <-- 30 <-- 40 <-- 50 <-- 60 <-- 70 After calling split method q2 will hold 20 <-- 30 <-- 40 and q1 will hold 50 <-- 60 <-- 70
void splitQueue(Queue q1){ Queue q2 = new Queue(q1.maxSize);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
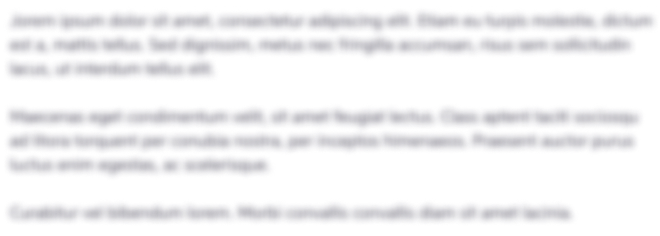
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started