Question
In your final project, you will create a program that will help you manage a collection of recipes. The Recipe class you build for this
In your final project, you will create a program that will help you manage a collection of recipes. The Recipe class you build for this milestone will hold all the details of the recipe, the methods to create a new recipe, and a method to print a recipe. In your final project submission, this class will also contain a custom method to add a new feature. In your submission for Milestone Two, you will include commented out pseudocode for this method.
In this milestone, you submit the final project version of your Recipe class. Your submission should include the Recipe.java file and a Recipe_Test.java file.
Your Recipe class should include the following items:
Instance variables: recipeName, servings, recipeIngredients, and totalRecipeCalories
Accessors and mutators for the instance variables
Constructors
A printRecipe() method
A createNewRecipe() method to build a recipe from user input
Pseudocode for the custom method selected from the list in Stepping Stone Lab Five
Your Recipe_Test.java file containing a main() method that:
Uses a constructor to create a new recipe
Accesses the printRecipe() method to print the formatted recipe
Invokes the createNewRecipe() method to accept user input.
Specifics
Here is the code so far that I've done for the Recipe Class
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipe.collection;
import java.util.ArrayList; import java.util.Scanner;
/** * * */ public class Recipe { private String recipeName; private int serving; private ArrayListrecipeIngredients; private double totalRecipeCalories; public Recipe() { this.recipeName = ""; this.serving = 0; //
Loops Class
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipe.collection;
import java.util.ArrayList; import java.util.Scanner;
/** * * */ public class Loops { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String recipeName = ""; ArrayList ingredientList = new ArrayList(); String newIngredient = ""; boolean addMoreIngredients = true; System.out.println("Please enter the recipe name: "); recipeName = scnr.nextLine(); do { System.out.println("Would you like to enter an ingredient: (y or n)"); String reply = scnr.next().toLowerCase(); //checking whether user entered y or n if y is entered then if(reply.equalsIgnoreCase("y")) { //asking user for ingredient name System.out.println("Enter ingredient name"); //storing into ingredient newIngredient=scnr.next(); //adding ingrdient to ist ingredientList.add(newIngredient); } else { //if n is entered then the do loop is exited addMoreIngredients=false; } } while (addMoreIngredients); for (int i = 0; i
The is the Recipe Main
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipe.collection;
/** * * */ public class RecipeCollection {
/** * @param args the command line arguments */ public static void main(String[] args) { // TODO code application logic here } }
Ingredient Class
package recipe.collection; import java.util.Scanner; /** * * For your Final Project, adapt your Ingredient java file to include * data type validation steps for each of the variables in the class: * * ingredientName (String) * ingredientAmount (float) * unitMeasurment (String) * number of Calories (double) * */ public class Ingredient { private String nameOfIngredient = ""; private float ingredientAmount = 0; private String unitOfMeasurement = ""; private int numberCaloriesPerCup = 0; private double totalCalories = 0.0; private float numberCups; public String getNameOfIngredient() { return nameOfIngredient; }
/** * @param nameOfIngredient the nameOfIngredient to set */ public void setNameOfIngredient(String nameOfIngredient) { this.nameOfIngredient = nameOfIngredient; } public String getUnitOfMeasurement() { return unitOfMeasurement; }
/** * @param UnitOfMeasurement the UnitMeasurement to set */ public void setUnitOfMeasurement(String unitOfMeasurement) { this.unitOfMeasurement = unitOfMeasurement; }
/** * @return the numberCups */ public float getNumberCups() { return numberCups; }
/** * @param numberCups the numberCups to set */ public void setNumberCups(float numberCups) { this.numberCups = numberCups; }
/** * @return the numberCaloriesPerCup */ public int getNumberCaloriesPerCup() { return numberCaloriesPerCup; }
/** * @param numberCaloriesPerCup the numberCaloriesPerCup to set */ public void setNumberCaloriesPerCup(int numberCaloriesPerCup) { this.numberCaloriesPerCup = numberCaloriesPerCup; }
/** * @return the totalCalories */ public double getTotalCalories() { return totalCalories; }
/** * @param totalCalories the totalCalories to set */ public void setTotalCalories(double totalCalories) { this.totalCalories = totalCalories; } // Sets the variables in the in the ingredient method to a default value public Ingredient() { this.nameOfIngredient = ""; this.numberCups = 0; this.numberCaloriesPerCup = 0; this.totalCalories = 0.0; } //Sets the parameters for the ingredient method public Ingredient(String nameOfIngredient, String tempUnitOfMeasurment, float numberCups, int numberCaloriesPerCup, double totalCalories) { this.nameOfIngredient = nameOfIngredient; this.numberCups = numberCups; this.numberCaloriesPerCup = numberCaloriesPerCup; this.totalCalories = totalCalories; } //allows the user to add ingredients to a recipe public Ingredient addIngredient() { int tempNumberCaloriesPerCup = 0; Scanner scnr = new Scanner(System.in); System.out.println("Please enter the name of the ingredient: "); //Validates that the user is entering a string while(!scnr.hasNext()) { System.out.println("Invalid input Type the name as a string:"); scnr.next(); } String tempNameOfIngredient = scnr.next();
System.out.println("Please enter the measurment type: "); // Validtes that the user has aentered a string while(!scnr.hasNext()) { System.out.println("Invalid input Type the name as a string:"); scnr.next(); } String tempUnitOfMeasurment = scnr.next(); System.out.println("Please enter the number of cups of " + nameOfIngredient + " we'll need: "); //Validates that the user has entered a float while(!scnr.hasNextFloat()) { System.out.println("Invalid input Type the number as a float:"); scnr.next(); } float tempNumberCups = scnr.nextFloat(); System.out.println("Please enter the name of calories per cup: "); //Validates that the user has entered a double while(!scnr.hasNextDouble()) { System.out.println("Invalid input Type the number as a double:"); scnr.next(); } double tempTotalCalories = scnr.nextDouble(); tempTotalCalories = numberCups * numberCaloriesPerCup; Ingredient tempNewIngredient = new Ingredient(tempNameOfIngredient, tempUnitOfMeasurment, tempNumberCups, tempNumberCaloriesPerCup, tempTotalCalories); return tempNewIngredient; } }
Ingredient Calculator Class
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipe.collection;
import java.util.Scanner; public class IngredientCalculator { //Calculates the calories that are in a recipe based on the ingredieints and measurment size of the ingredients /** * @param args the command line arguments */ public static void main(String[] args) { String nameOfIngredient = ""; float numberCups = 0; int numberCaloriesPerCup = 0; double totalCalories = 0.0; Scanner scnr = new Scanner(System.in); System.out.println("Please enter the name of the ingredient: "); nameOfIngredient = scnr.next(); System.out.println("Please enter the number of cups of " + nameOfIngredient + " we'll need: "); numberCups = (int) scnr.nextFloat(); System.out.println("Please enter the name of calories per cup: "); numberCaloriesPerCup = scnr.nextInt(); /** * Write an expression that multiplies the number of cups * by the Calories per cup. * Assign this value to totalCalories */ totalCalories = numberCups * numberCaloriesPerCup; System.out.println(nameOfIngredient + " uses " + numberCups + " cups and has " + totalCalories + " calories."); } }
Branches Class
package recipe.collection;
import java.util.Scanner;
public class Branches { public static void main(String[] args) { int numberCups = -1; final int MAX_CUPS = 100; /** * Add a CONSTANT variable MAX_CUPS assigned to the value 100 */ Scanner scnr = new Scanner(System.in); /**NESTED BRANCH: * Insert a nested branch that follows the following pattern: * * if numberCups is greater 1 AND less than or equal to MAX_CUPS: * print numberCups + " is a valid number of cups!" * * else: * print numberCups + " is a not valid number of cups!" * print "Please enter another number of cups between 1 and 100: " * numberCups = scnr.nextInt(); * * if numberCups is greater 1 AND less than or equal to MAX_CUPS: * print numberCups + " is a valid number of cups!" * * else if numberCups =1) && (numberCups=1) && (numberCups
Specifically, the following critical elements of the final project are addressed: Data Types: Your Recipe class should properly employ each of the following data types that meet the scenario's requirements where necessary: A. Utilize appropriate numerical and string data types to represent values for variables and attributes in your program. B. Populate a list or array that allows the management of a set of values as a single unit in your program I Algorithms and Control Structure: Your Recipe class should properly employ each of the following control structures as required or defined by the scenario where necessary: Utilize expressions or statements that carry out appropriate actions or that make appropriate changes to your program's state as represented in your program's variables. Employ the appropriate conditional control structures that enable choosing between options in your program. Utilize iterative control structures that repeat actions as needed to achieve the program's goal. A. B. C. Specifically, the following critical elements of the final project are addressed: Data Types: Your Recipe class should properly employ each of the following data types that meet the scenario's requirements where necessary: A. Utilize appropriate numerical and string data types to represent values for variables and attributes in your program. B. Populate a list or array that allows the management of a set of values as a single unit in your program I Algorithms and Control Structure: Your Recipe class should properly employ each of the following control structures as required or defined by the scenario where necessary: Utilize expressions or statements that carry out appropriate actions or that make appropriate changes to your program's state as represented in your program's variables. Employ the appropriate conditional control structures that enable choosing between options in your program. Utilize iterative control structures that repeat actions as needed to achieve the program's goal. A. B. C
Step by Step Solution
There are 3 Steps involved in it
Step: 1
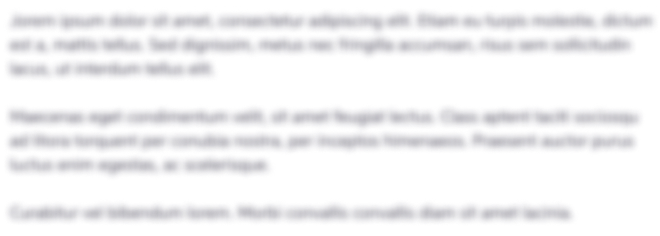
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started