Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include #include class Packet { private: int sourceId; int destId; std::string message; size _ t hash; public: Packet ( ) ; Packet ( int sourceId,
#include #include class Packet private: int sourceId; int destId; std::string message; sizet hash; public: Packet; Packetint sourceId, int destId, std::string message; int getSourceId; int getDestId; std::string getMessage; bool checkIntegrity; void corrupt; ; enum class MacPacketType Empty, Message, Success, Failure ; class MacPacket private: MacPacketType type; int macSourceId; int macDestId; std::vector path; Packet packet; public: MacPacket; MacPacketMacPacketType type, int macSourceId, int macDestId, std::vector& path, Packet& packet; static MacPacket createMessagePacketint macSourceId, int macDestId, std::vector& path, Packet& packet; static MacPacket createSuccessPacketint macSourceId, int macDestId, std::vector& path; static MacPacket createFailurePacketint macSourceId, int macDestId, std::vector& path; MacPacketType getType; int getMacSourceId; int getMacDestId; std::vector& getPath; Packet& getPacket; ; class Node private: int id; std::vector neighbors; MacPacket buffer; public: Node; Nodeint id std::vector& neighbors; int getId; std::vector& getNeighbors; MacPacket receiveMacPacket packet; ; class Network private: std::vector nodes; float corruptionRate; Node getNodeint id; std::vector calculatePathint source, int destination; public: Networkfloat corruptionRate; void addNodeint id std::vector& neighbors; void removeNodeint id; void simulatePacket packet; ; this is teh adhoc code and you can find the question below: AdHoc Network Simulation In this homework, you are going to simulate an AdHoc Network. Your simulation will have two layers with acknowledgment and resending. A header file is provided for your use. DO NOT CHANGE ANYTHING IN THE HEADER FILE INCLUDING ITS NAME OTHERWISE YOUR HOMEWORK WILL NOT BE GRADED. Packet Class Write a hash function for the packet class. Its calculation should include sourceId, dest Id and message. Implement the constructors for the packet class. Constructors should calculate the hash value and assign it to the member parameter. Implement the functions getSourceId, getDestId, getMessage. Implement the function checkIntegrity. It should recalculate the hash value and compare it to the member parameter. It should return false if the values do not match. Implement the function corrupt. It should change a random character in the message to a random ASCII character. MacPacket Class Implement the constructors. The default constructor should assign MacPacketType: : Empty as type. Implement the static functions which are shorthands for creating certain types of mac packets. Implement the functions getType, getMacSourceId, getMacDestId, getPath, getPacket. Node Class Implement the constructors. The default constructor should assign the id value as zero. Throughout this project, node id zero will be considered as the invalid idempty value. Implement the functions getId and getNeighbors. Implement the function receive. It should receive a packet and process it accordingly. It should log the events according to the sample output given. a If the received packet is a message packet and it is not corrupted, it should save the packet to the buffer and send it to the next receiver. If this node is the final destination, it should send an acknowledgmentsuccess packet backwards through the path. b If the received packet is a message packet and it is corrupted, it should request a resending. c If the received packet is a success packet, it should save the packet to the buffer and send it to the next receiver. If this node is the source, it should return an empty mac packet to end the simulation. d If the received packet is an error packet, it should resend the previous packet in the buffer. Network Class Implement the constructor and functions getNode, addNode, removeNode. All connections are twoway, hence addNode should add the new node as a neighbor to the already existing nodes. removeNode should remove the reference from the other nodes. Implement the function calculatePath. It should calculate the shortest path from source to destination and return a vector of nodes along the path. Implement the simulate function. a Calculate the path for the message to take. b In an infinite loop, send the packet to the destination node. If the response is an empty packet, end the simulation. If the response is a message packet, corrupt the message with the given probability Hint: float std: : rand RANDMAX corruptionRate Main Function
#include
#include
class Packet
private:
int sourceId;
int destId;
std::string message;
sizet hash;
public:
Packet;
Packetint sourceId, int destId, std::string message;
int getSourceId;
int getDestId;
std::string getMessage;
bool checkIntegrity;
void corrupt;
;
enum class MacPacketType
Empty,
Message,
Success,
Failure
;
class MacPacket
private:
MacPacketType type;
int macSourceId;
int macDestId;
std::vector path;
Packet packet;
public:
MacPacket;
MacPacketMacPacketType type, int macSourceId, int macDestId, std::vector& path, Packet& packet;
static MacPacket createMessagePacketint macSourceId, int macDestId, std::vector& path, Packet& packet;
static MacPacket createSuccessPacketint macSourceId, int macDestId, std::vector& path;
static MacPacket createFailurePacketint macSourceId, int macDestId, std::vector& path;
MacPacketType getType;
int getMacSourceId;
int getMacDestId;
std::vector& getPath;
Packet& getPacket;
;
class Node
private:
int id;
std::vector neighbors;
MacPacket buffer;
public:
Node;
Nodeint id std::vector& neighbors;
int getId;
std::vector& getNeighbors;
MacPacket receiveMacPacket packet;
;
class Network
private:
std::vector nodes;
float corruptionRate;
Node getNodeint id;
std::vector calculatePathint source, int destination;
public:
Networkfloat corruptionRate;
void addNodeint id std::vector& neighbors;
void removeNodeint id;
void simulatePacket packet;
; this is teh adhoc code and you can find the question below:
AdHoc Network Simulation
In this homework, you are going to simulate an AdHoc Network. Your simulation will have two layers with
acknowledgment and resending. A header file is provided for your use. DO NOT CHANGE ANYTHING IN
THE HEADER FILE INCLUDING ITS NAME OTHERWISE YOUR HOMEWORK WILL NOT BE GRADED.
Packet Class
Write a hash function for the packet class. Its calculation should include sourceId, dest Id
and message.
Implement the constructors for the packet class. Constructors should calculate the hash value
and assign it to the member parameter.
Implement the functions getSourceId, getDestId, getMessage.
Implement the function checkIntegrity. It should recalculate the hash value and compare
it to the member parameter. It should return false if the values do not match.
Implement the function corrupt. It should change a random character in the message to a
random ASCII character.
MacPacket Class
Implement the constructors. The default constructor should assign
MacPacketType: : Empty as type.
Implement the static functions which are shorthands for creating certain types of mac packets.
Implement the functions getType, getMacSourceId, getMacDestId, getPath,
getPacket.
Node Class
Implement the constructors. The default constructor should assign the id value as zero.
Throughout this project, node id zero will be considered as the invalid idempty value.
Implement the functions getId and getNeighbors.
Implement the function receive. It should receive a packet and process it accordingly. It
should log the events according to the sample output given.
a If the received packet is a message packet and it is not corrupted, it should save the
packet to the buffer and send it to the next receiver. If this node is the final destination,
it should send an acknowledgmentsuccess packet backwards through the path.
b If the received packet is a message packet and it is corrupted, it should request a
resending.
c If the received packet is a success packet, it should save the packet to the buffer and
send it to the next receiver. If this node is the source, it should return an empty mac
packet to end the simulation.
d If the received packet is an error packet, it should resend the previous packet in the
buffer.
Network Class
Implement the constructor and functions getNode, addNode, removeNode. All connections
are twoway, hence addNode should add the new node as a neighbor to the already existing
nodes. removeNode should remove the reference from the other nodes.
Implement the function calculatePath. It should calculate the shortest path from source to
destination and return a vector of nodes along the path.
Implement the simulate function.
a Calculate the path for the message to take.
b In an infinite loop, send the packet to the destination node. If the response is an empty
packet, end the simulation. If the response is a message packet, corrupt the message
with the given probability Hint: float std: : rand RANDMAX
corruptionRate
Main Function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
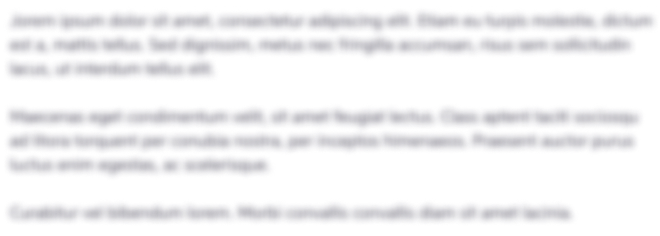
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started