Question
#include #include #include // Given a csv file, return the minimum of the specified column, excluding blanks double getMin(char csvfile[], char column[]); // Given a
#include
// Given a csv file, return the minimum of the specified column, excluding blanks double getMin(char csvfile[], char column[]); // Given a csv file, return the maximum of the specified column, excluding blanks double getMax(char csvfile[], char column[]); // Given a csv file, return the average of the specified column, excluding blanks double getAvg(char csvfile[], char column[]); // Given a csv file, return the number of students with their column value >= threshold, excluding blanks int getCount(char csvfile[], char column[], double threshold); // Given a csv file, return the weighted average of the specified student // or -1.0 if there is no such student. // A blank cell is viewed as 0 double getGrade(char csvfile[], char first[], char last[]);
int isValidColumn(char column[]) { char type; int num; sscanf(column, "%c%d", &type, &num); switch (type) { case 'L': case 'B': if (num10) return 0; break; case 'E': if (num8) return 0; break; case 'Q': if (num11) return 0; break; case 'P': if (num6) return 0; break; default: return 0; }
char column2[strlen(column)+1]; sprintf(column2, "%c%d", type, num); if (strcmp(column, column2)!=0) return 0;
return 1; }
void printHelp() { printf(" The valid commands: "); printf("\tmin column "); printf("\t*** find the minimum of the specified column, excluding blanks "); printf("\t*** for example: min P4 "); printf("\tmax column "); printf("\t*** find the maximum of the specified column, excluding blanks "); printf("\t*** for example: max E1 "); printf("\tavg column "); printf("\t*** find the average of the specified column, excluding blanks "); printf("\t*** for example: avg Q10 "); printf("\tcount column threshold "); printf("\t*** find the number of rows with its column value >= threshold, excluding blanks "); printf("\t*** for example: count L2 60 "); printf("\tgrade firstname lastname "); printf("\t*** find the weighted average of the specified student. A blank cell is viewed as 0 "); printf("\t*** for example: grade John Smith "); printf("\tquit "); printf("\t*** quit this program "); printf("\thelp "); printf("\t*** print this list "); }
int main(int argc, char *argv[]) { if (argc!=2) { printf("Usage: %s csvfile ", argv[0]); return 1; } FILE *fp=fopen(argv[1], "r"); if (fp==NULL) { printf("Unable to open %s for reading ", argv[1]); return 2; } fclose(fp);
while (1) { char cmd[30]; char column[30]; char line[300]; printf(" Enter a command: "); scanf("%s", cmd); if (strcmp(cmd, "quit")==0) break; if (strcmp(cmd, "grade")==0) { char first[30], last[30]; scanf("%s%s", first, last); double grade=getGrade(argv[1], first, last); if (grade>=0) printf("grade(%s %s)=%g ", first, last, grade); else printf("No student named %s %s ", first, last); } else if (strcmp(cmd, "min")==0) { scanf("%s", column); if (isValidColumn(column)) { double min=getMin(argv[1], column); printf("min(%s)=%g ",column, min); } else { printf("%s: invalid column name. ", column); fgets(line, 300, stdin); // skip the rest of line } } else if (strcmp(cmd, "max")==0) { scanf("%s", column); if (isValidColumn(column)) { double max=getMax(argv[1], column); printf("max(%s)=%g ", column, max); } else { printf("%s: invalid column name. ", column); fgets(line, 300, stdin); // skip the rest of line } } else if (strcmp(cmd, "avg")==0) { scanf("%s", column); if (isValidColumn(column)) { double avg=getAvg(argv[1], column); printf("avg(%s)=%g ", column, avg); } else { printf("%s: invalid column name. ", column); fgets(line, 300, stdin); // skip the rest of line } } else if (strcmp(cmd, "count")==0) { scanf("%s", column); if (isValidColumn(column)) { double threshold; scanf("%lf", &threshold); int count=getCount(argv[1], column, threshold); printf("count(%s>=%g)=%d ", column, threshold, count); } else { printf("%s: invalid column name. ", column); fgets(line, 300, stdin); // skip the rest of line } } else if (strcmp(cmd, "help")==0) { printHelp(); } else { printf("%s: invalid commmand. Type help for help. ", cmd); fgets(line, 300, stdin); // skip the rest of line } } return 0; }
Project Overview: In this project, yo w implement a set of funcions tat can be used to retrieve information from a CS100 grade book stored in a CSV (comma-separated values) file. The CSV file consists of 47 columns as shown below. The first two columns are always the first name and the last name. The order of the remaining 45 columns are unknown, and they can be in any order First Name and Last Name. L1 through L10 for 10 labs. B1 through B10 for 10 textbook exercises. 1 through Q11 for 11 quizzes. P1 through P6 for 6 projects. El through E8 for 8 exams (tracing and coding are considered as two different exams) The first row (or line) of the CSV file is the header, i.e. column names. Each following row represents a student. The first name and the last name in each row will not be blank or contain a space. Each score under the 45 headings will be either blank (wit no score) or a real number. A blank score is considered as O in some calculations and is ignored in the others You are asked to implement the following five functions in the functions.c file. double getMin (char csvfile, char column[])Given a CSV file, return the minimum score of the specified column. The blank cells are excluded from the calculation double getMax (char csvfile[,char column [])Given a CSV file, return the maximum score of the specified column. The blank cells are excluded from the calculation double getAvg (char csvfile, char column [1)Given a CSV file, return the average score of the specified column. The blank cells are excluded from the calculation int getCount (char csvfilet,char column [i,double threshold) Given a CSV file, return the number of students with their specified scorethreshold. The blank cells are excluded from the calculation double getGrade (char csvfile[, char first,cha last[ Given a CSV file, return the weighted average of the specified student. A blank cel is viewed as 0 in the calculation. The weight percentage for cach column is specified below. The lowest quiz score w be dropped from the We 1% for each lab t Percenta Score L1 thro (21 throu h Q1 1 B1 through B10 P1 through P6 LIO 0-100 0-10 0-100 0-100 | 1 % for each 1% for each exercise 2% for PI 4% each for P2, P3 5% each for P4, P5, P6 5% for each exam 7.5% for each exam El through E6 E7, E8 0-50 0-75 Project Overview: In this project, yo w implement a set of funcions tat can be used to retrieve information from a CS100 grade book stored in a CSV (comma-separated values) file. The CSV file consists of 47 columns as shown below. The first two columns are always the first name and the last name. The order of the remaining 45 columns are unknown, and they can be in any order First Name and Last Name. L1 through L10 for 10 labs. B1 through B10 for 10 textbook exercises. 1 through Q11 for 11 quizzes. P1 through P6 for 6 projects. El through E8 for 8 exams (tracing and coding are considered as two different exams) The first row (or line) of the CSV file is the header, i.e. column names. Each following row represents a student. The first name and the last name in each row will not be blank or contain a space. Each score under the 45 headings will be either blank (wit no score) or a real number. A blank score is considered as O in some calculations and is ignored in the others You are asked to implement the following five functions in the functions.c file. double getMin (char csvfile, char column[])Given a CSV file, return the minimum score of the specified column. The blank cells are excluded from the calculation double getMax (char csvfile[,char column [])Given a CSV file, return the maximum score of the specified column. The blank cells are excluded from the calculation double getAvg (char csvfile, char column [1)Given a CSV file, return the average score of the specified column. The blank cells are excluded from the calculation int getCount (char csvfilet,char column [i,double threshold) Given a CSV file, return the number of students with their specified scorethreshold. The blank cells are excluded from the calculation double getGrade (char csvfile[, char first,cha last[ Given a CSV file, return the weighted average of the specified student. A blank cel is viewed as 0 in the calculation. The weight percentage for cach column is specified below. The lowest quiz score w be dropped from the We 1% for each lab t Percenta Score L1 thro (21 throu h Q1 1 B1 through B10 P1 through P6 LIO 0-100 0-10 0-100 0-100 | 1 % for each 1% for each exercise 2% for PI 4% each for P2, P3 5% each for P4, P5, P6 5% for each exam 7.5% for each exam El through E6 E7, E8 0-50 0-75
Step by Step Solution
There are 3 Steps involved in it
Step: 1
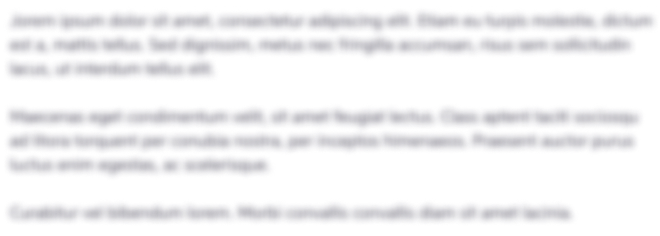
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started