Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include #include #include #include #include #include using namespace std; / / Base class for all types of clients class Client { protected: string name; string
#include
#include
#include
#include
#include
#include
using namespace std;
Base class for all types of clients
class Client
protected:
string name;
string address;
string phone;
string id;
string password;
double balance;
public:
Clientstring name, string address, string phone, string id string password
: namename addressaddress phonephone idid passwordpassword balance
virtual void depositdouble amount
balance amount;
cout "Deposit successful. New balance: balance endl;
virtual void withdrawdouble amount
if amount balance
cout "Insufficient funds." endl;
else
balance amount;
cout "Withdrawal successful. New balance: balance endl;
virtual void viewBalance
cout "Account Balance: balance endl;
virtual void transferClient& recipient, double amount
if amount balance
cout "Insufficient funds." endl;
else
balance amount;
recipient.depositamount;
cout "Transfer successful. New balance: balance endl;
virtual void viewTransactionHistory
Implement transaction history retrieval
cout "Transaction History" endl;
;
Derived class for User Clients
class UserClient : public Client
private:
string cnic;
double dailyWithdrawalLimit;
string cardNumber;
string pin;
public:
UserClientstring name, string address, string phone, string cnic, string id string password, double dailyWithdrawalLimit
: Clientname address, phone, id password cniccnic dailyWithdrawalLimitdailyWithdrawalLimit
void depositdouble amount override
Client::depositamount;
Update transaction history
void withdrawdouble amount override
if amount dailyWithdrawalLimit
cout "Exceeded daily withdrawal limit endl;
else
Client::withdrawamount;
Update transaction history
void transferClient& recipient, double amount override
if recipientid id
cout "Cannot transfer to the same account." endl;
else
Client::transferrecipient amount;
void requestLoandouble amount
Implement loan request mechanism
;
Derived class for Company Clients
class CompanyClient : public Client
private:
string companyName;
string taxNumber;
double dailyWithdrawalLimit;
public:
CompanyClientstring companyName, string address, string taxNumber, string id string password, double dailyWithdrawalLimit
: ClientcompanyName address, NA id password companyNamecompanyName taxNumbertaxNumber dailyWithdrawalLimitdailyWithdrawalLimit
void depositdouble amount override
Client::depositamount;
Update transaction history
void withdrawdouble amount override
if amount dailyWithdrawalLimit
cout "Exceeded daily withdrawal limit endl;
else
Client::withdrawamount;
Update transaction history
void transferClient& recipient, double amount override
if recipientid id
cout "Cannot transfer to the same account." endl;
else
Client::transferrecipient amount;
void requestLoandouble amount
Implement loan request mechanism
;
Class for Banking Employees
class BankingEmployee
private:
string id;
string password;
public:
BankingEmployeestring id string password
: idid passwordpassword
void viewAllAccounts
Read and display all client accounts from files
cout "Viewing all accounts" endl;
void approveAccountClient& client
Implement account approval mechanism
cout "Account approved" endl;
void rejectAccountClient& client
Implement account rejection mechanism
cout "Account rejected" endl;
void approveLoanRequestCompanyClient& company
Implement loan approval mechanism
cout "Loan request approved" endl;
void rejectLoanRequestCompanyClient& company
Implement loan rejection mechanism
Step by Step Solution
There are 3 Steps involved in it
Step: 1
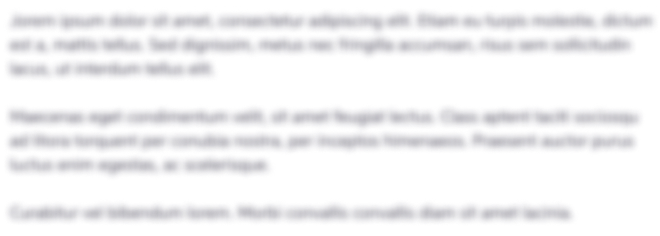
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started