Question
#include #include #include #include using namespace std; #define MAX 32 class Person { protected: char name[MAX]; public: Person() { name[0] = '0'; } ~Person() {
#include
#define MAX 32
class Person { protected: char name[MAX]; public: Person() { name[0] = '\0'; } ~Person() { } void operator=(const Person& src) { strcpy(name, src.name); } void inputPerson() { char n[MAX]; cout << "Enter person's name: "; cin.getline(n, MAX); setName(n); } void printPerson() { cout << "Name: " << getName(); } void setName(const char* n) { strcpy(name, n); } const char* getName() const { return name; } }; class Faculty : public Person { protected: char department[MAX]; public: Faculty() { department[0] = '\0'; } ~Faculty() { } void operator=(const Faculty& src) { Person::operator=(src); strcpy(department, src.department); }
void inputPerson() { cout << "Please enter Faculty Information: "; Person::inputPerson(); char d[MAX]; cout << "Enter faculty's department: "; cin.getline(d, MAX); setDepartment(d); } void printPerson() { Person::printPerson(); cout << " Department: " << getDepartment() << endl; } const char* getDepartment() const {return department;} void setDepartment(const char* d) { strcpy(department, d); } }; class Student : public Person { protected: char major[MAX]; public: Student() { major[0] = '\0'; } ~Student() { } void operator=(const Student& src) { Person::operator=(src); strcpy(major, src.major); } void inputPerson() { cout << "Please enter Student Information: "; Person::inputPerson(); char m[MAX]; cout << "Enter student's major: "; cin.getline(m, MAX); setMajor(m); } void printPerson() { Person::printPerson(); cout << "\tMajor: " << getMajor() << endl; } const char* getMajor() const {return major;} void setMajor(const char* m) { strcpy(major, m); } };
#define CLASSROOM_CAP 35 /*********************************/ //Need to define class CourseInterface{ protected: char title[MAX]; char type[MAX]; Faculty Lecturer; Student* roster; int count,capacity; public: CourseInterface(); CourseInterface(const CourseInterface&); void operator=(const CourseInterface&); ~CourseInterface(); void setTitle(const char*); void setType(const char*); const char* getTitle() const; const int getCount() const; const char* getType() const; virtual void inputCourse() = 0; virtual void printCourse() = 0; };
//Need to define class Course : public CourseInterface{ public: Course(); Course(const Course&); void operator=(const Course&); ~Course(); void inputCourse(); void printCourse(); };
//Need to define class Lecture : public Course{ public: Lecture(); Lecture(const Lecture&); ~Lecture(); void operator=(const Course&); };
//Need to define class Lab : public Course{ public: Faculty labIntructor; Lab(); Lab(const Lab&); ~Lab(); void operator=(const Course&); void inputCourse(); void printCourse(); void inputLabFaculty(); }; /*********************************/ class CourseInfo { protected: Course* lecture; Course* lab;
public: CourseInfo(){ lecture = new Lecture; lab = new Lab; } ~CourseInfo() { if(lecture) delete lecture; if(lab) delete lab; lecture=NULL; lab=NULL; } void inputCourseInfo() { cout << " Input Lecture Information "; lecture->inputCourse();
cout << " Input Lab Information "; lab->inputCourse(); } void printCourseInfo() { cout << " --Lecture-- "; lecture->printCourse();
cout << " --Lab-- "; lab->printCourse(); } };
/****CourseInterface class****/ CourseInterface::CourseInterface() { title[0] = '\0'; type[0] = '\0'; //strcpy(title, "default title"); roster = new Student[CLASSROOM_CAP]; // default or roster = NULL; capacity =0; count =0; } CourseInterface::CourseInterface(const CourseInterface& src){ strcpy(title, src.title); strcpy(type, src.type); if(src.roster != NULL){ roster =new Student[CLASSROOM_CAP]; roster = src.roster; } } void CourseInterface::operator=(const CourseInterface& p1){ if(roster == p1.roster){ return; } if(roster != NULL){ delete [] roster; roster = '\0'; } strcpy(title, p1.title); strcpy(type, p1.type); if(roster != NULL){ roster = new Student[CLASSROOM_CAP]; roster = p1.roster; } } CourseInterface::~CourseInterface() { title[0] = '\0'; type[0] = '\0'; if(roster != NULL ) delete [] roster; } void CourseInterface::setTitle(const char* ti){ strcpy(title, ti); } void CourseInterface::setType(const char* ty){ strcpy(type, ty); } const char* CourseInterface::getTitle() const{return title;} const int CourseInterface::getCount() const{return count;} const char* CourseInterface::getType() const{return type;} /****CourseInterface end****/
/****Course class****/ Course::Course(){ //CourseInterface c=new CourseInterface(); Faculty Lecturer; } Course::Course(const Course&c): CourseInterface(c){ //CourseInterface ci=new CourseInterface(c); } void Course::operator=(const Course&src){ CourseInterface::operator=(src); } Course::~Course(){} void Course::inputCourse(){ cout << "Please enter Course Title: "; char tit[CLASSROOM_CAP]; cin.getline(tit,CLASSROOM_CAP); cout << "Please enter Course Type: "; char typ[CLASSROOM_CAP]; cin.getline(typ,CLASSROOM_CAP); setTitle(tit); setType(typ); Faculty::inputPerson(); lecturer.inputPerson(); Student* roster;
for(int i=0;i /****Lecture class****/ Lecture::Lecture():Course(){ Course* crs= new Course(); } Lecture::Lecture(const Lecture&crs){ Course* crs1=new Course(crs); } Lecture::~Lecture(){} void Lecture::operator=(const Course&src){ Course::operator=(src); setType("Lecture"); } /****Lecture end****/ /****Lab class****/ Lab::Lab(){ Course* crs=new Course(); labIntructor = crs.Lecture(); setType("Lab"); } Lab::Lab(const Lab&lb){ Course* crs=new Course(lb); labIntructor = lb.labIntructor; setType("Lab"); } Lab::~Lab(){} void Lab::operator=(const Course&src){ Course::operator=(src); setType("Lab"); } void Lab::inputCourse(){ labIntructor.inputPerson(); Course::inputCourse(); } void Lab::printCourse(){ Course::printCourse(); labIntructor.printPerson(); } void Lab::inputLabFaculty(){ labIntructor.inputPerson(); } /****Lab end****/ int main() { Course *c= NULL; CourseInfo *ci = NULL; int choice; do{ cout << " "; cout << "1. Input Lecture " << "2. Input Lab " << "3. Print Course " << "4. Input CourseInfo " << "5. Print CourseInfo " << "0. Exit " << "Enter choice: "; cin >> choice; cin.ignore(256,' '); cout << " "; switch(choice) { case 1: if(c) { delete c; c=NULL; } c = new Lecture; c->inputCourse(); break; case 2: if(c) { delete c; c=NULL; } c = new Lab; c->inputCourse(); break; case 3: if(c) { c->printCourse(); }else { cout << "Input a course first "; } break; case 4: if(ci){ delete ci; c=NULL; } ci = new CourseInfo; ci->inputCourseInfo(); break; case 5: if(ci){ ci->printCourseInfo(); } else { cout << "Input a full course first "; } break; case 0: cout << "Good Bye "; if(c) delete c; if(ci) delete ci; c=NULL; ci=NULL; break; default: cout << "Invalid option "; } }while(choice!=0); cout << endl; return(0); } here is my code I have problem in my code; compiler notes: 267:25: error: cannot call member function 'void Faculty::inputPerson()' without object Faculty::inputPerson(); ^ :268:4: error: 'lecturer' was not declared in this scope lecturer.inputPerson(); ^ 273:33: error: cannot call member function 'void Student::inputPerson()' without object Student::inputPerson(); ^ In member function 'virtual void Course::printCourse()': 278:50: error: cannot call member function 'void Person::printPerson()' without object cout << "\tLecturer: " << Person::printPerson() << endl; ^ 280:26: error: cannot call member function 'void Student::printPerson()' without object Student::printPerson(); ^ In constructor 'Lab::Lab()': 303:27: error: request for member 'Lecture' in 'crs', which is of pointer type 'Course*' (maybe you meant to use '->' ?) labIntructor = crs.Lecture();in c++ ____________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
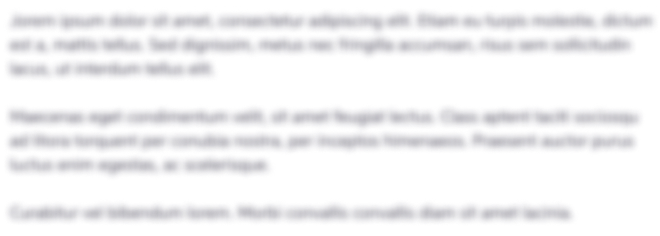
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started