Answered step by step
Verified Expert Solution
Question
1 Approved Answer
-------------------------------------------- #include #include #include using std::string; using std::cin; using std::cout; using std::cerr; using std::endl; using std::stringstream; //****************** //The Node class //****************** template class Node {
--------------------------------------------
#include | |
#include | |
#include | |
using std::string; | |
using std::cin; | |
using std::cout; | |
using std::cerr; | |
using std::endl; | |
using std::stringstream; | |
//****************** | |
//The Node class | |
//****************** | |
template | |
class Node { | |
public: | |
T data{}; | |
Node | |
}; | |
//****************** | |
// The linked list base class | |
//****************** | |
template | |
class LinkedListBase { | |
public: | |
~LinkedListBase(); | |
T getFifthElement() const { cerr | |
void insertNewFifthElement(const T& data) { cerr | |
void deleteFifthElement() { cerr | |
void swapFifthAndSeventhElement() { cerr | |
T getLast() const; | |
void pushFront(const T& data); | |
void pushBack(const T& data); | |
void popFront(); | |
void popBack(); | |
string getStringFromList(); | |
protected: | |
Node | |
Node | |
unsigned int count{ 0 }; | |
}; | |
template | |
LinkedListBase | |
Node | |
while (temp) { | |
head = head->link; | |
delete temp; | |
temp = head; | |
} | |
} | |
//This method helps return a string representation of all nodes in the linked list, do not modify. | |
template | |
string LinkedListBase | |
stringstream ss; | |
if (!this->head) { | |
ss | |
} | |
else { | |
Node | |
ss data; | |
currentNode = currentNode->link; | |
while (currentNode) { | |
ss data; | |
currentNode = currentNode->link; | |
}; | |
} | |
return ss.str(); | |
} | |
template | |
T LinkedListBase | |
if (this->tail) { | |
return this->tail->data; | |
} | |
else { | |
throw 1; | |
} | |
} | |
template | |
void LinkedListBase | |
if (!head) { | |
// Scenario: The list is empty | |
Node | |
temp->data = data; | |
this->head = temp; | |
this->tail = temp; | |
count++; | |
} | |
else { | |
// Scenario: One or more nodes | |
Node | |
temp->data = data; | |
temp->link = this->head; | |
this->head = temp; | |
count++; | |
} | |
} | |
template | |
void LinkedListBase | |
if (!head) { | |
//Scenario: The list is empty | |
Node | |
temp->data = data; | |
this->head = temp; | |
this->tail = temp; | |
count++; | |
} | |
else { | |
Node | |
temp->data = data; | |
this->tail->link = temp; | |
this->tail = temp; | |
count++; | |
} | |
} | |
template | |
void LinkedListBase | |
if (!head) { | |
// Scenario: The list is empty | |
cout | |
return; | |
} | |
else if (this->head == this->tail) { | |
// Scenario: One node list | |
this->tail = nullptr; | |
delete this->head; | |
this->head = nullptr; | |
count--; | |
} | |
else { | |
// Scenario: General, at least two or more nodes | |
Node | |
temp = this->head->link; | |
delete this->head; | |
this->head = temp; | |
count--; | |
} | |
} | |
template | |
void LinkedListBase | |
if (!this->head) { | |
// Scenario: The list is empty | |
cout | |
return; | |
} | |
else if (this->head == this->tail) { | |
// Scenario: One node list | |
this->tail = nullptr; | |
delete this->head; | |
this->head = nullptr; | |
count--; | |
} | |
else { | |
// Scenario: General, at least two or more nodes | |
Node | |
temp = head; | |
while (temp->link != this->tail) { | |
temp = temp->link; // This is like i++; | |
} | |
// temp is now at the second to tail node | |
delete this->tail; | |
this->tail = temp; | |
this->tail->link = nullptr; | |
count--; | |
} | |
} | |
//********************************** | |
//Write your code below here | |
//********************************** | |
template | |
class SinglyLinkedList : public LinkedListBase | |
public: | |
// TODO, your methods declarations here | |
}; | |
// TODO, your method definitions here | |
//********************************** | |
//Write your code above here | |
//********************************** |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
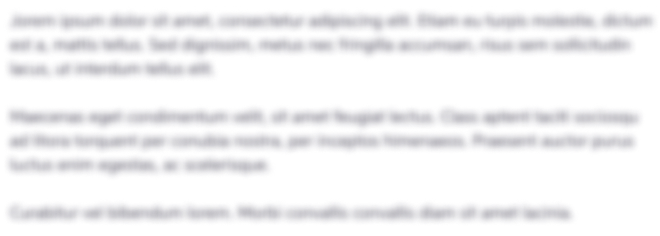
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started