Question
#include #include struct card { char suit ; char face; }; void createDeck( card* deck, char *suits, char *faces) { int areEqual(struct card deck1[52], struct
#include #include struct card { char suit ; char face; }; void createDeck( card* deck, char *suits, char *faces) { int areEqual(struct card deck1[52], struct card deck2[52]) { for(int i=0; i<52; i++) { if(deck1[i].suit != deck2[i].suit || deck1[i].face != deck2[i].face) return -1; } return 1; } void printDeck(struct card deck[52]) { for(int i=0; i<4; i++) { for(int j=0; j<13; j++) { // need to print 10 for 0 (as we are storing 0 for 10) if(j == 4) { printf("%c10 ", deck[13*i+j].suit, deck[13*i+j].face); } else { printf("%c%c ", deck[13*i+j].suit, deck[13*i+j].face); } } printf(" "); } } void createCopy(struct card *deck, struct card *newDeck) { for(int i=0; i<52; i++) { newDeck[i].suit = deck[i].suit; newDeck[i].face = deck[i].face; } } void perfectShuffle(struct card *deck, struct card *newDeck) { int k=0; //newDeck = new struct card[52]; for(int i=0; i<26; i++) { newDeck[k].suit = deck[i].suit; newDeck[k].face = deck[i].face; newDeck[k + 1].suit = deck[i+26].suit; newDeck[k + 1].face = deck[i+26].face; k += 2; } } int main() { struct card deck[52]; struct card temp[52]; struct card start[52]; char suits[4] = {'C', 'D', 'H', 'O'}; char faces[13] = {'A', 'K', 'Q', 'J', '0', '9', '8', '7', '6', '5', '4', '3', '2'}; // 0 is for 10, since face can store a character for(int i=0; i<4; i++) { for(int j=0; j<13; j++) { deck[13*i + j].suit = suits[i]; deck[13*i + j].face = faces[j]; } } int shuffles = 0; createCopy(deck, start); for(int i=0; i<10; i++) { printf(" "); createCopy(deck, temp); perfectShuffle(temp, deck); // printf(" "); printDeck(deck); // printf(" "); // printDeck(temp); shuffles ++; if(areEqual(deck, start) == 1) break; } printf(" Number of shuffles for perfect shuffle = %d",shuffles); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
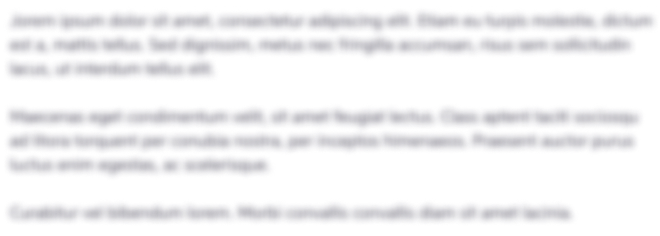
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started