Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include //... more header as needed /********* DO NOT CHANGE THESE CONSTANTS IN YOUR FINAL SUBMISSION *********/ #define MAX_SIZE 20 // must be 20 in
#include//... more header as needed /********* DO NOT CHANGE THESE CONSTANTS IN YOUR FINAL SUBMISSION *********/ #define MAX_SIZE 20 // must be 20 in your final submission #define SUCCESS 0 char order[5]; // global variable, asc or desc /****************** YOUR CODE STARTS HERE ******************/ /************************************************************/ /* Input: array A with "siz" elements in it and an integer d Output: d is added to the array. Return a non-negative number if the addition is successful. Return a negative number if the addition is unsuccessful. Unsuccessful/Error condition(s): A reaches the MAX_SIZE already. */ int myAdd( int A[], int * siz, int d ) { /* ADD YOUR CODE HERE */ } /* Input: array A with "siz" elements in it, and an integer d Output: If d is found in the array, return the index of the cell containing d. Otherwise return a negative number if d is not found. Unsuccessful/Error condition(s): d is not found. note: this must be a 'binary search', and can be either iterative or recursive(i.e., no loops) for recursive, you may need a recursive helper funciton with heading similar to this int myBinarySearch_Recursive_helper( int A[], int d, int start, int end ) */ int myBinarySearch( int A[], int siz, int d ) { /* ADD YOUR CODE HERE */ } /* */ /* Input: array A with "siz" elements in it, and an integer d Output: Return a negative number if d is not found. Otherwise d is removed from the array and return 0. Error condition(s): d does not exist in A. */ int myRemove( int A[], int *siz, int d ) { /* ADD YOUR CODE HERE */ } /* Input: array A with "siz" elements Output: Display the array on the standard output with one space between every two numbers. Print a new line after the last element. */ void printArray( int A[], int siz ) { int i; printf("[ "); for ( i = 0; i = SUCCESS ) { printArray( myArray, size ); } else printf( "Failed to add %d. ", data ); break; case 'r': /* remove */ case 'R': retCode = myRemove( myArray, &size, data ); if ( retCode >= SUCCESS ) { printArray( myArray, size ); } else printf( "Failed to remove %d. ", data ); break; case 's': /* search */ case 'S': retCode = myBinarySearch( myArray, size, data ); if( retCode >= 0 ) printf( "Found %d at index %d. ", data, retCode ); else printf( "Not found %d. ", data ); break; case 'q': /* quit */ case 'Q': /* To quit the input, enter an arbitrary integer and character (action) 'q' or 'Q'. This is not elegant but makes the code simpler. */ /* Do nothing but exit the switch statement */ break; default: printf( "Invalid operation %c ", action ); } } while ( action != 'q' && action != 'Q' ); return 0; } // end main
Note: Keep the array sorted in order O(n)
Given an array arr of integers that is sorted (ascending or descending), and is maintained by its 'size', implement the following functions: - myAdd (arr, *int siz, d) : add an integer d to the array; Parameter siz is an integer pointer that stores the address of size variable defined in main. After adding, the array maintains sorted - ascending or descending as specified by the user at the beginning. Return a number 0 if the operation is successful; return a negative number if the operation is unsuccessful. The adding is unsuccessful if, before insertion, the MAX_SIZE has reached. - myremove (arr, *int siz, d) : remove integer d from the array; Parameter siz is an integer pointer that stores the address of size variable defined in main. Return a number 0 if the operation is successful; return a negative number otherwise (e.g., d is not found in the array). Assume no duplicate values in the array. After removal, the array remains sorted. - Note that you don't need to remove d from the memory. All you need is to make sure that d is no longer in the valid range of array, and thus does not show in the output of the current 'size' elements. And, all existing elements maintained sorted. - myBinarysearch (arr, int siz, d) : given an integer d, determine if d is in the array arr. Parameter siz is an integer that stores the current size of the array. If d is found in the array, return the index of the cell containing d. Return a negative number otherwise (e.g., d is not found in the array). Assume no duplicate values in the array. - Note that since the array is maintained sorted, instead of using linear search which has complexity O(n), you need to do binary search, which has complexity log(n). You may have learnt binary search in other courses. If not, search the literatures for more sort order: ascending (asc) or descending (desc) ? abc sort order: ascending (asc) or descending (desc) ? as sort order: ascending (asc) or descending (desc) ? Asc sort order: ascending (asc) or descending (desc) ? asc a 10 [10 ] a 98 [9810 ] a 5 [98510 ] a 67 [9851067] s 5 Found 5 at index 1 s 10 Found 10 at index 2 a 67 [98675 ad 67] a 90 Failed to add 90 r 67 [98675 ro 10] r 1 Failed to remove 1 s 5 Not found 5 r 67 [98510] r 5 [9810 ] s 5 Not found 5 r 5 Failed to remove 5 r 98 4 sort order: ascending (asc) or descending (desc) ? des sort order: ascending (asc) or descending (desc) ? Desc sort order: ascending (asc) or descending (desc) ? desc a 10 [10 ] a 98 [1098 ] a 5 [10598 ] a 67 [6710 s 98 ] s 5 Found 5 at index 2 s 10 Found 10 at index 1 a 67 [671056798] a 90 Failed to add 90 r 67 [1056798] r 1 Failed to remove 1 s 5 Not found 5 r 67 [10598 ] r 5 [1098] s 5 Not found 5 r 5 Failed to remove 5 r 98 [10] r 10 [1] F 10 Failed to remove 10 s 6 Not found 6 a 17 [ 17 ] a 2 [ 172] a 13 [ 17132] a 15 [1715132] r 13 [ 17 15 2 2 ] q 100Step by Step Solution
There are 3 Steps involved in it
Step: 1
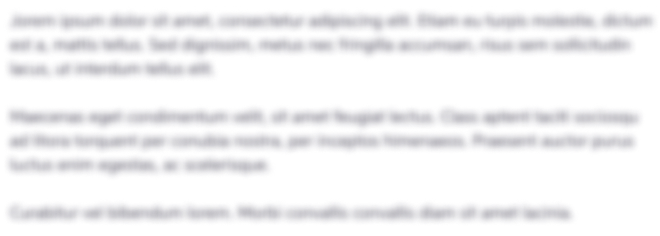
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started