Question
#include using namespace std; // constants for maximum width and character symbols const unsigned int max_width = 120; char background_char = '.'; char main_char =
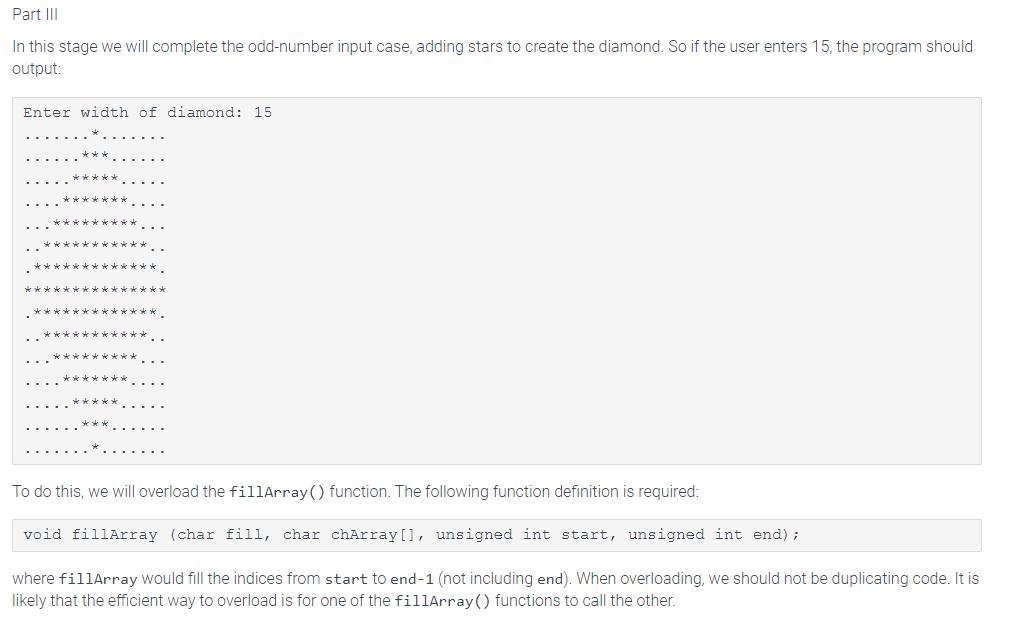
#include
using namespace std;
// constants for maximum width and character symbols
const unsigned int max_width = 120;
char background_char = '.';
char main_char = '*';
char main_char_left = '/';
char main_char_right = '\\';
// function prototype declarations
void fillArray(char fill, char chArray[], unsigned int width);
void printArray(const char chArray[], const unsigned int width);
int main() {
unsigned int user_input;
// prompting the user to enter the width of the diamond
cout << "Enter width of diamond: ";
cin >> user_input;
// checking if user input is greater than the maximum width
if (user_input > max_width) {
// displaying an error message and exit with an error code
cout << "Enter width of diamond: " << user_input << endl;
cout << "Max width is " << max_width << "." << endl;
return 1;
} else {
cout << "Enter width of diamond: Error! Max width is " << max_width << " . Exiting." << endl;
}
// adjusting width for even numbers (make it odd)
if (user_input % 2 == 0) {
user_input--;
}
// declaring and initializing a 2D array to store the diamond pattern
char diamond[100][100]; // Use a sufficiently large array size
// filling the array with background characters
for (int i = 0; i < user_input; i++) {
fillArray(background_char, diamond[i], user_input);
}
// output the diamond pattern
for (int i = 0; i < user_input; i++) {
printArray(diamond[i], user_input);
cout << endl;
}
return 0;
}
// function to fill an array with the specified character
void fillArray(char fill, char chArray[], unsigned int width) {
for (unsigned int i = 0; i < width; i++) {
chArray[i] = fill;
}
}
// function to print the array
void printArray(const char chArray[], const unsigned int width) {
for (unsigned int i = 0; i < width; i++) {
cout << chArray[i];
}
Part III In this stage we will complete the odd-number input case, adding stars to create the diamond. So if the user enters 15, the program should output: Enter width of diamond: 15 * ** To do this, we will overload the fillArray() function. The following function definition is required: void fillArray (char fill, char chArray[], unsigned int start, unsigned int end); where fillArray would fill the indices from start to end-1 (not including end). When overloading, we should not be duplicating code. It is likely that the efficient way to overload is for one of the fillArray() functions to call the other.
Step by Step Solution
3.32 Rating (149 Votes )
There are 3 Steps involved in it
Step: 1
In order to address the challenge of overloading the fillArray function to create a diamond pattern in C it is essential to have a solid understanding of function overloading and how it can be utilize...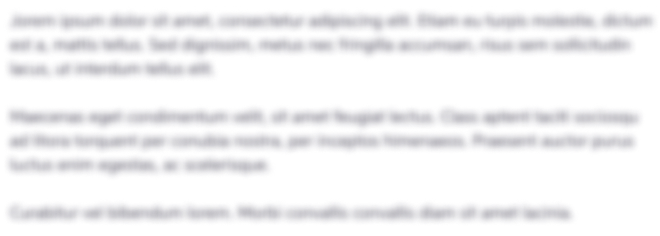
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started