Question
#include using namespace std; #include #include #include //the doubly linked list that allows us to store 2 different lists one for a complete list and
#include
using namespace std;
#include
#include
#include
//the doubly linked list that allows us to store 2 different lists one for a complete list and one for a even list
struct node {
int data;
node *next,*previous;
}*head=NULL,*tail=NULL ,*curr=NULL, *headeven=NULL ,*taileven=NULL;
using namespace std;
//precondition:error message of type string
//post condition: file filled with string error messages
//description:
void sendtoerrorlog(string errormessage)
{
fstream logfile("log.txt",ios::app);
logfile << errormessage;
logfile << endl;
logfile.close();
}
//precondition:integer data from a file
//postcondition:added node to a link list
//description: creates a doubly linked list that can be traversed to sort data from a file
void deletenode(int num)
{
int i=0;
string error;
curr = head;
node *temp,*temp2;
while (curr != NULL)
{
if (curr->data == num)
{
temp=curr->next;
temp2 = curr->previous;
curr = NULL;
curr = temp2;
curr->next = temp;
i++;
cout << "deleted " << num << endl;
//error = char(num);
error= to_string(num);
error.append(" was deleted");
sendtoerrorlog(error);
}
else
{
curr= curr->next;
}
}
if (i == 0)
{
cout << " sorry none of the integers you entered exist thus you cannot delete them" << endl;
}
}
void addnode(int dat)
{
if (head == NULL)
{
curr = new node;
curr->data = dat;
head = curr;
tail = curr;
curr->previous = NULL;
curr->next = NULL;
}
else
{
curr = new node;
curr->data = dat;
curr->previous = tail;
tail->next = curr;
tail = curr;
tail->next = NULL;
}
if (dat % 2 == 0)
{
if (headeven == NULL)
{
curr = new node;
curr->data = dat;
headeven = curr;
taileven = curr;
curr->previous = NULL;
curr->next = NULL;
}
else
{
curr = new node;
curr->data = dat;
curr->previous = tail;
taileven->next = curr;
taileven = curr;
taileven->next = NULL;}}
static int i = 0;
if (i != 0)
{
string error2 = to_string(dat);
error2.append(" was added");
sendtoerrorlog(error2);
}
i++;
}
void printreverseeven()
{
int i = 0;
curr = tail;
while (curr != NULL)
{
if (curr != NULL&& curr->previous!=NULL)
{
if (curr->data % 2 == 0)
{
i++;
cout << curr->data << endl;
}
}
curr = curr->previous;
}
}
void printeven()
{
curr = head;
while (curr != NULL)
{
curr = curr->next;
if (curr != NULL)
{
if (curr->data % 2 == 0)
{
cout << curr->data << endl;
}
}
}
}
void fowardprintnode()
{
curr = head;
while (curr!= NULL)
{
curr = curr->next;
if (curr != NULL)
{
cout << curr->data << endl;
}
}
}
void printreverse()
{
curr = tail;
while (curr != NULL)
{
curr = curr->previous;
if (curr != NULL&&curr->previous!=NULL)
{
cout << curr->data << endl;
}
}
}
void sortnode()
{
int temp=0,temp2=0;
curr = tail;
while (curr != NULL)
{
temp = curr->data;
if (curr->previous != NULL)
{
curr = curr->previous;
if (curr->data > temp)
{
temp2 = curr->data;
curr = curr->next;
curr->data = temp2;
curr = curr->previous;
curr->data = temp;
}
}
else { curr = curr->previous; }
}
}
void readfile()
{
int h;
ifstream myReadFile;
myReadFile.open("integer.dat");
int output[100];
int z = 0;
if (myReadFile.is_open()) {
cout << "file is open" << endl;
if (!(myReadFile >> h))
{
cout << ", but the file is empty" << endl;
}
else
{
output[z] = h;
if (output[z] < 0) { sendtoerrorlog("error one or more integers are negative");
cout << "error one or more integeres are negative."< } while (!myReadFile.eof()) { addnode(output[z]); z++; myReadFile >> output[z]; if (output[z] < 0) { sendtoerrorlog("error one or more integers are negative"); cout << "error one or more integeres are negative." << endl; } } addnode(output[z]); } } for (int i=0;i<10000;i++) { sortnode(); } myReadFile.close(); } int main() { int answer = 0, integer = 0; bool h = false; cout << "Welcome to integer enterer 5000!" << endl; char choice, ascenddecending; bool correct = false; readfile(); cout << "we have 2 different lists for you to print out:" << endl; cout << "The even list or the complete list enter L for complete list and E for only the even list to be displayed." << endl; cin >> choice; if ((choice != 'l' && choice != 'L' && choice != 'e' && choice != 'E')) { correct = true; } while (correct) { cout << "wrong input please reenter" << endl; cin >> choice; if (choice == 'l' || choice == 'L' || choice == 'e' || choice == 'E') { correct = false; } } cout << "would you like to print the list in ascending or descending order?"; cin >> ascenddecending; if (ascenddecending != 'a' && ascenddecending != 'A' && ascenddecending != 'd' && ascenddecending != 'D') { correct = false; } while (correct) { cout << "wrong input please reenter" << endl; cin >> choice; if (ascenddecending == 'a' || ascenddecending == 'A' || ascenddecending || 'd' && ascenddecending == 'D') { correct = false; } } if ((choice == 'l' || choice == 'L') && (ascenddecending == 'a' || ascenddecending == 'A')) { //print full list and in ascending order fowardprintnode(); } if ((choice == 'l' || choice == 'L') && (ascenddecending == 'd' || ascenddecending == 'D')) { //print full list and in desending order printreverse(); } if ((choice == 'e' || choice == 'e') && (ascenddecending == 'd' || ascenddecending == 'D')) { //print even list and in desending order printreverseeven(); } if ((choice == 'e' || choice == 'e') && (ascenddecending == 'a' || ascenddecending == 'a')) { //print even list and in ascending order printeven(); // } cout << "would you like to add or delete an integer?0 for delete 1 for add" << endl; cin >> answer; while ((cin.fail()) || (answer != 0 && answer != 1)) { cout << "wrong answer please enter a 0 or 1" << endl; cin.clear(); cin.ignore(1000, ' '); cin >> answer; } if (answer == 1) { cout << "enter your integer please" << endl; cin >> integer; if (integer < 0) { h = true; } while (cin.fail() || h) { cout << "Error: Enter only a positive integer please" << endl; cout << "Enter in a new value " << endl; cin >> integer; if (h >= 0) { h = false; } } addnode(integer); } else if (answer == 0) { cout << "enter your integer please" << endl; cin >> integer; if (integer < 0) { h = true; } while (cin.fail() || h) { cout << "Error: Enter only a positive integer please" << endl; cout << "Enter in a new value " << endl; cin >> integer; if (h >= 0) { h = false; } } deletenode(integer); } system("pause"); return 0; } convert this c++ code to pseudocode please
Step by Step Solution
There are 3 Steps involved in it
Step: 1
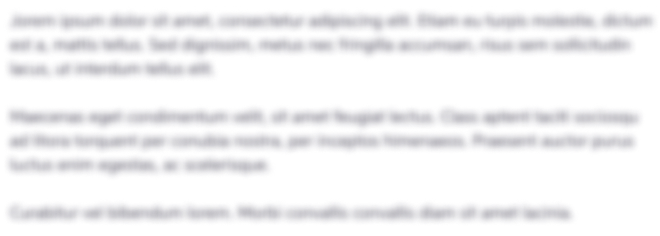
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started