Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include using namespace std; struct Node { int data; Node * left; Node * right; } ; Node * createNode ( int data ) {
#include
using namespace std;
struct Node
int data;
Node left;
Node right;
;
Node createNodeint data
Node newNode new Node;
newNodedata data;
newNodeleft NULL;
newNoderight NULL;
return newNode;
Node insertNode root, int data
if root NULL
root createNodedata;
else if data rootdata
rootleft insertrootleft, data;
else
rootright insertrootright, data;
return root;
void preorderTraversalNode root
if root NULL
return;
cout rootdata ;
preorderTraversalrootleft;
preorderTraversalrootright;
int main
Node root NULL;
root insertroot;
root insertroot;
root insertroot;
root insertroot;
root insertroot;
root insertroot;
cout "Preorder Traversal: ;
preorderTraversalroot;
return ;
Using the code above, make the following Program Changes
Modify the program so that it includes struct s for each of the eight shapes that were the subject of Assignment Name these as follows: Square, Rectangle, Circle, Triangle, Cube, Box, Cylinder, and Prism. Include attributes of the double data type for the required dimensions for each for example, length and width for the Rectangle Provide a descriptive name for each of these data elements for example, "radius" instead of r Do NOT include any other attributes, and do NOT include any "member functions" avoid the temptation to extend the specifications beyond what is required. If you include attributes for area, volume, or perimeter, you are not doing this right!
Write supporting functions to do console output each shape for example, void outputBoxostream& const Box&; which should output to either cout or fout whichever is the first parameter in a call, using the same format as v Note that cout is an object of type ostream So use cout as the first parameter in the function call in order to produce console output. Use the exact same supporting functions for text file output, which should output to fout Create, open, and close fout in int main fout is also an object of type ostream Remember, the ROUNDING Format the calculated results to digits after the decimal outsetfios::fixed; out.precision; But echo the input values without formatting. outunsetfios::fixed; out.precision;
Use any type of array for your bag C C or STL vector your choice. STL vectors track their or size, so if you use a C or C array instead, you'll have to use an int to track size yourself.
Write loops one to process the input file and fill the bag, one for console output, one for TXT output, and one for deallocation. If you don't have loops, or if you do more than one of these things in any single loop, you're not doing this right!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
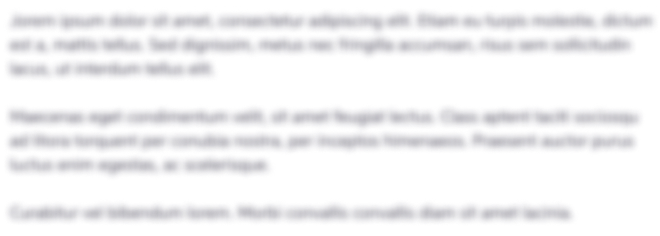
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started