Question
Included below are the All_test.java, Test_toString, ArrayStack, LinkedStack, and stacked interface.java classes package testStack; //import static org.junit.Assert.*; //import org.junit.After; //import org.junit.Before; //import org.junit.Test; import org.junit.runner.RunWith;
Included below are the All_test.java, Test_toString, ArrayStack, LinkedStack, and stacked interface.java classes
package testStack;
//import static org.junit.Assert.*; //import org.junit.After; //import org.junit.Before; //import org.junit.Test; import org.junit.runner.RunWith; import org.junit.runners.Suite; import org.junit.runners.Suite.SuiteClasses;
@RunWith(Suite.class) @SuiteClasses({ Test_toString.class }) //@SuiteClasses({ Test_toString.class, Test_sizeIs.class, Test_clear.class})
public class All_Tests { // dummy class used to call the suite of tests }
package testStack;
import static org.junit.Assert.*;
import org.junit.After; import org.junit.Assert; import org.junit.Before; import org.junit.Test;
import stackPackage.ArrayStack; import stackPackage.LinkedStack;
public class Test_toString {
/* * Class to test the toString method added to the Stack ADT of Lab03 * * test sizeIs on an empty stack * a stack with one element * a stack with many (but less than full) elements * and a "full" ArrayStack */ /* ArrayStack
@Test public void test_toString_on_an_emptyStack() { Assert.assertEquals("Empty Stack", stk1.toString()); } @Test public void test_toString_on_a_stack_with_1_element() { stk1.push(5); Assert.assertEquals("bottom|5|top", stk1.toString()); }
@Test public void test_toString_on_a_stack_with_multiple_elements() { stk1.push(5); stk1.push(4); stk1.push(3); Assert.assertEquals("bottom|5|4|3|top", stk1.toString()); } @Test public void test_toString_on_a_full_stack() { stk1.push(1); stk1.push(2); stk1.push(3); stk1.push(4); stk1.push(5);
Assert.assertEquals("bottom|1|2|3|4|5|top", stk1.toString()); } @After public void tearDown() throws Exception { }
}
package stackPackage;
public class LinkedStack
protected LLNode
public LinkedStack() {
top = null;
}
public void push(T element)
// Places element at the top of this stack.
{
LLNode
newNode.setLink(top);
top = newNode;
}
public void pop()
// Throws StackUnderflowException if this stack is empty,
// otherwise removes top element from this stack.
{
if (!isEmpty()) {
top = top.getLink();
} else
throw new StackUnderflowException("Pop attempted on an empty stack.");
}
public T top()
// Throws StackUnderflowException if this stack is empty,
// otherwise returns top element from this stack.
{
if (!isEmpty())
return top.getInfo();/* LinkedStack stk;
@Before
public void setUp() throws Exception {
stk = new LinkedStack
}
*/
else
throw new StackUnderflowException("Top attempted on an empty stack.");
}
public boolean isEmpty()
// Returns true if this stack is empty, otherwise returns false.
{
if (top == null)
return true;
else
return false;
}
}
package stackPackage;
//import java.util.Scanner;
public class ArrayStack
protected final int DEFCAP = 100; // default capacity
protected T[] stack; // holds stack elements
protected int topIndex = -1; // index of top element in stack
public ArrayStack() {
stack = (T[]) new Object[DEFCAP];
}
public ArrayStack(int maxSize) {
stack = (T[]) new Object[maxSize];
}
public void push(T element)
// Throws StackOverflowException if this stack is full,
// otherwise places element at the top of this stack.
{
if (!isFull()) {
topIndex++;
stack[topIndex] = element;
} else
throw new StackOverflowException("Push attempted on a full stack.");
}
public void pop()
// Throws StackUnderflowException if this stack is empty,
// otherwise removes top element from this stack.
{
if (!isEmpty()) {
stack[topIndex] = null;
topIndex--;
} else
throw new StackUnderflowException("Pop attempted on an empty stack.");
}
public T top()
// Throws StackUnderflowException if this stack is empty,
// otherwise returns top element from this stack.
{
T topOfStack = null;
if (!isEmpty())
topOfStack = stack[topIndex];
else
throw new StackUnderflowException("Top attempted on an empty stack.");
return topOfStack;
}
public boolean isEmpty()
// Returns true if this stack is empty, otherwise returns false.
{
if (topIndex == -1)
return true;
else
return false;
}
public boolean isFull()
// Returns true if this stack is full, otherwise returns false.
{
if (topIndex == (stack.length - 1))
return true;
else
return false;
}
}
package stackPackage;
public interface StackInterface
{
void pop() throws StackUnderflowException;
// Throws StackUnderflowException if this stack is empty,
// otherwise removes top element from this stack.
T top() throws StackUnderflowException;
// Throws StackUnderflowException if this stack is empty,
// otherwise returns top element from this stack.
boolean isEmpty();
// Returns true if this stack is empty, otherwise returns false.
String toString();
// returns a string containing contents of the stack from bottom to top.
// like "bottom|5|4|3|top" (if pushed in the order 5, 4, 3)
}
1. Download the file Lab03GenericStackADTSource.z ip to a directory available to Eclipse, and import it as an existing project as you have been doing with other labs. 2. Open the file All_Tests.java and run it as a JUnit Test. You should get a red bar, because the Test.toString class is not written yet. (The default toString is being used.) (a) Write methods toString) for the ArrayStack and the LinkedStack classes, and test them both to get a green bar. You will need to comment/uncomment in the top of Test toString.java to test both classes. The method should print the contents of the stack from bottom to top. That is, if the sequence of push operations was push(5); push (4); push (3); then the method returns the string "bottom | 51413ltop". It returns Empty Stack" if called with an empty stack. Note that the header is already entered in the file StackInterface.java. Note also that the test methods implement an integer stack (b) Write tests and methods for returning an int equal to the number of items on the stack, with the following signature: public int sizeIs) Write the tests first, so that you can go from red bar to green bar. When writing the tests, first make a plan using comments. Then write the tests, keeping the comments in the file. Note that you should add a header in the file StackInterface.java. (c) Use a similar test/write (red, green) process to create methods to reset the stack to its original state. On the array side, you should set stack[i] to null to be thorough. A header should be added to StackInterface.java. (d) Finally, write an additional test set that tests your toString methods if strings are stored in the stack, rather than integers 3. Answer the following questions is the file answers.txt (a) Why are we putting the method headers in file StackInterface.java, rather than BoundedStackInterface.java and UnboundedStackInterface.java? (b) What is the T in the declaration StackInterfaceStep by Step Solution
There are 3 Steps involved in it
Step: 1
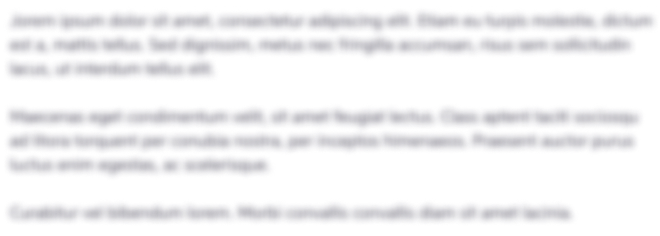
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started