Question
InfoTc 1040 Introduction to Problem Solving and Programming Users Class (Use Python 3.4.2) In this programming assignment, you will create the following: - A User
InfoTc 1040 Introduction to Problem Solving and Programming Users Class (Use Python 3.4.2)
In this programming assignment, you will create the following:
- A User class that stores information about an user.(last module)
- A Users class that stores and displays User objects.
- A usermanager program that uses the User and Users classes.
These elements are described below.
User Class
Write a class named User that has the following attributes and methods. Save this class as User.py
Attributes:
_username | To store the name of the player |
_wins | To store the number of wins |
_ties | To store the number of ties |
_losses | To store the number of losses |
Methods:
_init_:
This method takes 4 parameters, the username, wins, ties, and losses (when initializing the last 3 will be zeroes eg. (John,0,0,0)) It should create __username, __wins, __ties, and __losses based on those parameters and it should create __created based on the current time
get_username:
Returns the value of __username field
get_user_wins:
Return the value of the __wins field
set_user_wins:
Takes in 1 parameter and sets __wins to that parameter
get_user_ties:
Returns the value of the __ties field
set_user_ties:
Takes in 1 parameters and sets __ties to that parameter
get_user_losses:
Returns the value of the __losses field
set_user_losses:
Takes in 1 parameter and sets __losses to that parameter
display_user:
Displays the username and stats (check sample output for formatting)
Users Class
Write a class named Users with the following attributes and methods. Save this class as Users.py
Attributes
__user_list: a list used to store User objects.
Methods
#You will be making these same functions for the final project but adding some more error checking to them
__init__: use this method to create an empty list for __user_list.
create_user: this method should receive a User object and append it to the __user_list list.
#THIS IS EXTRA CREDIT read_user: this method should receive a name and return a found user object
delete_user: this method should receive a user name and then remove them from the list.
display_user: this method should display the current read user. By current. If we have a list of object and we read an object (grab one from the list) and have it locally to work with we would display that local object.
display_users: this method will print information about each of the User objects within __user_list. If no users have been added to the list, it should print out a message saying that there are no users.
UserManager Program
Once you have written the User and Users classes, create another program called usermanager.py. This program will use User.py and Users.py as modules.
In usermanager.py, create a Users object and print a menu with three options(four with extra credit): Create User, Read User, Delete User, Display User, Display Users, and Exit.
Create User:
Takes in 2 parameters, username and wins. Then checks to see if the user names exists. If it exists we return False, if it doesnt exist, we add it to the list and return True. #NOTE we are setting the wins with what the user types in, the ties and losses are still done by random.randint(1,10)
If the name exists, we print the error:
[!]Error: This User already exists.
Read User:
Takes in a parameter, username, and checks to see if it exists. If it exists, we return the True AND the user object which has the same name. If it doesnt exist, we return False and the user object. At the beginning of the function set found_user = None. We will return True, found_user or return False, found_user. To check if a user exists, we will compare each user name in the list (for user in self.__user_list:) to username. So compare each user.get_name() to username If the name doesnt exist, we print the error: [!]Error: This User doesn't exist.
Delete User:
Takes in a parameter, a username, and deletes that user from the list. If the name doesnt exist, we print the error: [!]Error: This User doesn't exist.
Display Current User: If you have read in a User or have created a User this will display the information about the current user. This will trigger the user.display_user() method
Display Users: choosing this option should trigger the Users objects display_users. This should output information about all of the users from the list.
Exit: this should exit the program. If exit is not selected, the program should loop and display the options again.
Program Output
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 4
There is no current user to display
User Menu --------- [1] Create User
[2] Read User
[3] Delete User [4] Display User [5] Display Users [6] Exit
What would you like to do? 5
User List --------- There are no users!
User Menu
--------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 1 What is the user's name? John How many wins does John have? 300
User Menu ---------
[1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 1 What is the user's name? John How many wins does John have? 200
[!]Error: This User already exists.
User Menu --------- [1] Create User
[2] Read User
[3] Delete User
[4] Display User
[5] Display Users [6] Exit
What would you like to do? 2
What is the user's name? Claire
[!]Error: This User doesn't exist.
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 1 What is the user's name? Claire How many wins does Claire have? 10
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 1 What is the user's name? Suzy How many wins does Suzy have? 200
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 2
What is the user's name? John
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 4
Username: John
Wins | Ties | Losses |
300 | 1 | 1 |
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 5 User List ---------
Username | Wins | Ties | Losses |
John | 300 | 1 | 1 |
Claire | 10 | 7 | 8 |
Suzy | 200 | 10 | 7 |
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 2
What is the user's name? Claire
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 4
Username: Claire
Wins | Ties | Losses |
10 | 7 | 8 |
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 3
What is the user's name? Suzy
Suzy has been removed!
User Menu --------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 5 User List ---------
Username | Wins | Ties | Losses |
John | 300 | 1 | 1 |
Claire | 10 | 7 | 8 |
User Menu
--------- [1] Create User
[2] Read User [3] Delete User
[4] Display User
[5] Display Users
[6] Exit
What would you like to do? 6
Thanks for using the user manager!
Testing
Run the program you write and verify that the information entered matches the information displayed and that the input prompts and output utilize the format specification provided.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
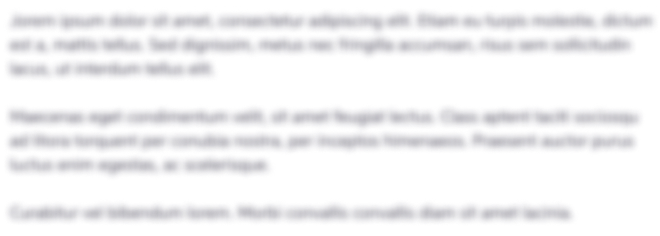
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started