Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Inheritance/ Polymorphism Questions public class Person private String name; private int age: //constructor public Person (String n, int a) { name = n; age =
Inheritance/ Polymorphism Questions
public class Person private String name; private int age: //constructor public Person (String n, int a) { name = n; age = a; //other methods not shown public class Child extends Person private String school; // constructor public Child (String n, int a, Strings) { ........missing code public String getSchool() { return school; Which of the following replacements for missing code in the Child class constructor would compile without error? A. supern, a); C.name = n; schools; age = a; B. school = 5; school = 5; supern, a); D. Super(n); age = a; school = 5; E. Supern, a, s); Assume that the Child constructor has been defined correctly. Consider the following code segment. Person p = new Child("Chris", 10, "Lincoln School"); System.out.println(p.getSchool()); hich of the following statements about this code segment is true? A. It will not compile because the type of pis Person, and class Person has no getSchool method. B. It will not compile because the type of pis Person, and the type of the value assigned to p is Child. C. It will compile, but there will be a runtime error when the first line is executed because the type of pis Person, and the type of the value assigned to p is Child D. It will compile, but there will be a runtime error when the second line is executed because the type of pis Person, and class Person has no getSchool method. E. It will compile and run without error, and will print Lincoln School Questions 3 & 4 refer to the following incorrect) definitions of the Car and SportsCar classes public class Car { private double price; private double milesPerGallon; private int daysSince Oilchange; //constructor public Car (double p, double mps) { price = p; milesPerGallon = mpg: daysSinceOilchange = 0; public boolean needsOilchangel ) { return (daysSinceOilchange >= 90); public void setDaysSinceOilchange(int days) { daysSince Oilchange = days; public int getDaysSinceOilchangel) { return daysSinceOilchange; public class SportsCar extends Car { private int maxSpeed: //constructor public SportsCar (double p, double mpg, int max) { maxSpeed = max; //override the needsOilchange method public boolean needsOilchangel ) { return (getDaysSinceOilchange() >= 30); 3. The SportsCar constructor shown above does not compile. Which of the following correctly explains the error? A. Parameters p and mpg are not used. B. The order of the parameters is not correct. C. The types of the left and right-hand sides in the assignment to maxSpeed do not match. D. The price, milesPerGallon, and daysSinceOilchange fields are not initialized E. There is no explicit call to the superclass constructor, and the superclass has no default (no-argument) constructor 4. Assume that the Sports Car constructor has been defined correctly. Consider the following code segment. Car myCar = new SportsCar(30000, 14.5, 120); myCar.setDaysSinceOilchange(40); if (myCar.needsOilchangel) System.out.println("Change oil"); else System.out.println("Do not change oil"); Which of the following statements about this code segment is true? A. It will compile and run without error and will print Change oil. B. It will compile and run without error and will print Do not change oil. C. It will not compile because the type of myCar is Car, and the type of the value assigned to myCar is Sports Car. D. It will not compile because myCar points to a SportsCar, and Sports Car has no setDaysSinceOilchange method. E. It will compile, but there will be a runtime error when the second line is executed because myCar points to a SportsCar, andSports Car has no setDaysSinceOilchange method. 5. Consider writing a program to be used to manage information about the animals on a farm. The farm has three kinds of animals: cows, pigs, and goats. The cows are used to produce both milk and meat. The goats are used only to produce milk, and the pigs are used only to produce meat. Assume that an Animal class has been defined. Which of the following is the best wasy to represent the remaining data? A. Define two subclasses of the Animal class: Milk Producer and MeatProducer. Define two subclasses of the Milk Producer class: Cow and Goat; and define two subclasses of the MeatProducer class: Cow and Pig. B. Define three subclasses of the Animal class: Cow, Goat, and Pig. Also define two interfaces: Milk Producer and Meat Producer. Define the Cow and Goat classes to implement the Milk Producer interface, and define the Cow and Pig classes to implement the Meat Producer interface. C. Define five new classes, not related to the Animal class: Cow, Goat, Pig, Milk Producer, and Meat Producer D. Define five subclasses of the Animal class: Cow, Goat, Pig, Milk Producer, and Meat Producer. E. Define two subclasses of the Animal class: Milk Producer and Meat Producer. Also define three interfaces: Cow, Goat, and Pig. Define the Milk Producer class to implement the Cow and Goat interfaces, and define the MeatProducer class to implement the Cow and Pig interfaces. Questions 6 - 8 refer to the following incomplete definitions of the Book and TextBook classes (note that TextBook is a subclass of Book) public class Book private String name; private double price; //constructor public Book (String n, double p) { name = n; price : System.out.println("created a book"); public static Book cheapestbook (Book( ) booklist) //precondition: booklist.length > 0 //return the book with the lowest price ....missing code public class Text Book extends Book { private String class Usedin; public TextBook (String n, double p, String class) { //constructor supern, p); classUsedin class; System.out.println("created a textbook"); public static Textbook getBookForClass(String classname, Textbook[ ) textbooklist) { I/return the first textbook in textbooklist that is used in the given class for null if there is no such textbook) ...missing code 6. Assume that the following declarations have been made: Book b; Book) B; TextBook tb; TextBook[TB; And that all four variables have been properly initialized. Which of the following statements will not compile? A. b = Book.cheapestBook(B); B. b = Book.cheapestBook(TB); C. b = TextBook.getBookForClass("Intro to Java", TB); D. tb = TextBook.getBookForClass("Intro to Java", B): E. tb = TextBook.getBookForClass("Intro to Java", TB): 7. Note that methods cheapestBook and getBookForClass are declared static. Which of the following statements is true? A. It is appropriate for the two methods to be static because they both operate on arrays passed as parameters rather than on fields of the two classes. B. Although the code works as is, because the two methods return single objects rather than arrays, they really shouldn't be declared static. C. It is appropriate for the cheapestBook method to be either static or nonstatic, but because Textbook is a subclasss of Book, the getBookForClass method must be static if the cheapestBook method is static. D. Because the two methods are static, the missing code for the method bodies cannot contain any recursive calls. E. It is appropriate for the two methods to be static, but if they had return type void they could not be static. 8. Consider the following declaration and initialization: Book oneBook = new Textbook("ABC", 10.50, "kindergarten"); Which of the following statements about this code is true? A. The code will not compile because the type of the left-hand side of the assignment is Book, and the type of the right-hand side is Textbook B. The code will compile, but there will be a runtime error when it is executed because the type of the left-hand side of the assignment is Book, and the type of the right-hand side is TextBook. C. The code will compile and execute without error; only created a book will be printed. D. The code will compile and execute without error; only created a textbook will be printed The code will compile and execute without error, and both created a book and created a textbook will be printed. Questions 9 & 10 refer to the following Horse, WorkHorse, and Race Horse classes public class Horse { private int age; private double price; public Horse(int a, double p) // constructor age = a; price = p; public int getAge() { return age; } public double getPricel) { return price; } price void setAge(int newAge) ( age = newAge; } public void set Price (double newPrice) { price - newPrice;) public void haveBirthday() { age++; price = price. 1 price; public class WorkHorse extends Horse private double weight; //constructor public WorkHorse(int a, double p, double w) { super(a, p); weight = w; public class Race Horse extends Horse //constructor public Race Horsefint a, double p) { superla, p); public void have Birthday() { setAge(getAge( ) + 1); setPrice(getPrice )..2* getPrice()); 9. Which of the following statements will compile without error? A. Horse h = new WorkHorse/2, 1000); B. Horse h = new RaceHorse/2, 1000); C. WorkHorse h = new Race Horse/2, 1000); D. RaceHorse h = new WorkHorse2, 1000); E. Race Horse h= new Horse(2, 1000); 10. Assume that variable h has been declared to be of type Horse, & has been initialized to represent a horse whose price is 100 Which of the following statements about the call h.have Birthdayl) is true? A. Whatever kind of horse variable h actually points to the price of that horse after the call will be 90. B. Whatever kind of horse variable h actually points to the price of that horse after the call will be 80. C. If variable h actually points to a Race Horse object, then the price of that horse after the call will be 80. D. If variable h actually points to a Work Horse object, there will be a runtime error, since class Work Horse has no havebirthday method. E. if variable h actually points to a WorkHorse object, the price of that horse after the call will still be 100, since class WorkHorse has no havebirthday method. public class BankAccount private double myBalance; public BankAccount) (myBalance = 0; } public BankAccount(double balance) myBalance = balance; } public void deposit(double amount) { mybalance += amount; } public void withdraw(double amount) { myBalance - amount; } public double getBalance() return myBalance;) ) public class SavingsAccount extends BankAccount private double myinterestrate; public SavingsAccount { /* implementation not shown */ } public SavingsAccount(double balance, double rate) { /* implementation not shown */ } public void addinterest) // Add interest to balance { /* Implementation not shown */ } } public class Checking Account extends Bank Account private static final double FEE - 2.0; private static final double MIN_BALANCE = 50.0, public CheckingAccount(double balance) { /* implementation not shown */) /* FEE of $2 deducted if withdrawal leaves balance less than MIN BALANCE Allows for negative balance. / public void withdraw(double amount) { /* implementation not shown 7) ) 11. Of the methods shown, how many different nonconstructor methods can be invoked by a Savings Account object? d4 5 12. Which of the following correctly implements the default constructor of the SavingsAccount class? myinterest Rate = 0 super il super myinterest Rate = 0; Ill super : a. I only b. I and I only C. ll and lll only d. ill only e L II, and 13. Which is a correct implementation of the constructor with parameters in the SavingsAccount class? a. myBalance = balance; Csuper : myinterestRate = rate; myinterest Rate = rate; b.getBalance() = balance; d. super balance myinterest Rate = rate; myinterest Rate = rate; e. super balance, rate): 14. Which is a correct implementation of the Checking Account constructor? super(balance); Il supert): deposit(balance); Ill deposit(balance); a. I only b. ll only ill only d. tl and ill only e. 1.1, and it C 15. Which is correct implementation code for the withdraw method in the Checking Account class? a. super withdraw(amount) if (myBalance 








Step by Step Solution
There are 3 Steps involved in it
Step: 1
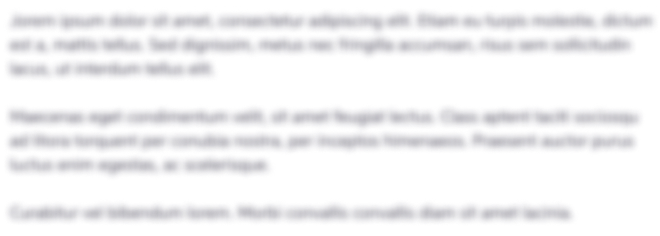
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started