Question
Input is from a file: The first upper case character on each line determines what type of object it is (C for Circle, R for
Input is from a file: The first upper case character on each line determines what type of object it is (C for Circle, R for Rectangle, F for Fraction, I for FeetInches). A C will be followed by a Double (the radius of the Circle you will create) An R will be followed by 2 Doubles, the length and width of the Rectangle you will create An F will be followed by 2 ints, the numerator and denominator An I will be followed by 2 ints, the feet and inches. After you create each object put it into an ArrayList. (There will be 4 ArrayLists, one for Circles, one for Rectangles, one for Fractions, one for FeetInches) USE A SWITCH STATEMENT to determine which constructor to call (which object to make). After you have input all your objects into the ArrayLists print them all out, First all the Circles from that ArrayList, then all the Rectangles, etc. State clearly what you are printing. e.g. These are the Circles:
This is what I have so far:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner;
public class H6 {
public static class FeetInches { private int feet; private int inches;
public FeetInches() {//write the code for the default constructor here feet = 0; inches = 0; } public FeetInches(int f, int i) { feet = f + i/12; inches = i%12; } public void setFeet(int f){ feet = f; } public void setInches(int i){ feet = feet + i/12; inches = i%12; } public int getFeet(){ return feet; } public int getInches(){ return inches; } public String toString()
{
return " Feet: " + feet + " Inches: " + inches ;
} } public static class Fraction { //default constructor private int numerator; private int denominator; //Constructor public Fraction() { numerator = 0; denominator=1; } //Checks if final fraction is positive and divides gcd public Fraction(int n, int d) { int g=gcd(n,d); if (n < 0 || d < 0) System.out.println("Enter a Postive Number"); numerator = n / g; denominator = d / g; } //Getters and Setters public int getNumerator() { return numerator; }
public void setNumerator(int n) { int d=denominator; int g=gcd(n,d); if (n<0) System.out.println("Enter a Postive Number"); numerator = n / g; denominator = d / g; }
public int getDenominator() { return denominator; }
public void setDenominator(int d) { int n=numerator; int g=gcd(n,d); if (d<0) System.out.println("Enter a Postive Number"); numerator = n / g; denominator = d / g; } //Adds Fractions public Fraction add(Fraction g) { int a=this.numerator; int b=this.denominator; int c=g.numerator; int d=g.denominator; Fraction v=new Fraction(a*d+b*c,b*d); return v; } //Subtracts Fractions public Fraction subtract(Fraction g) { int a=this.numerator; int b=this.denominator; int c=g.numerator; int d=g.denominator; Fraction v=new Fraction(a*d-b*c,b*d); return v; } //Multiply Fractions public Fraction multiply(Fraction g) { int a=this.numerator; int b=this.denominator; int c=g.numerator; int d=g.denominator; Fraction v=new Fraction(a*c,b*d); return v; } //Divide Fractions public Fraction divide(Fraction g) { int a=this.numerator; int b=this.denominator; int c=g.numerator; int d=g.denominator; Fraction v=new Fraction(a*d,b*c); return v; } //toString public String toString() { return numerator + "/" + denominator; } //Calculates greatest common factor private int gcd(int int1, int int2) { if ((int1 % int2) == 0) { return int2; } else{ return gcd(int2, int1 % int2); } } } public static class Circle {
// attribute
private double radius;
// constructors
public Circle() {
radius = 0.0;
}
public Circle(double r) {
radius = r;
}
// accessors
public double getRadius() {
return radius;
}
// mutators
public void setRadius(double r) {
radius = r;
}
// methods
public double circumference() {
return 2 * Math.PI * radius;
}
public double area() {
return Math.PI * radius * radius;
}
public String toString() {
return "Circle of radius " + radius;
} } public static class Rectangle
{
private double length, width;
public Rectangle()
{
length=0;
width=0;
}
public Rectangle(double len, double wid)
{
length=len;
width=wid;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double area()
{
return length*width;
}
public double perimeter()
{
return 2*(length+width);
}
public String toString()
{
return "Length: " + length + " Width: " + width;
} } public static void main(String[] args) {
File inFile = new File("H6.in");
Scanner fileInput = null;
try {
fileInput = new Scanner(inFile);
} catch (FileNotFoundException ex) {
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
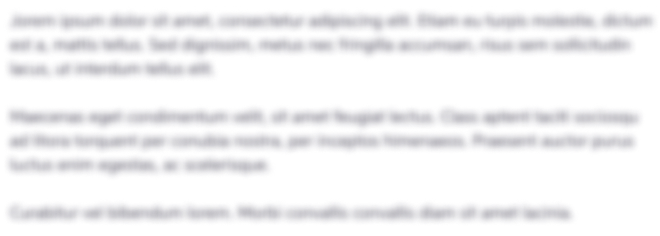
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started