Question
Instructions: Compile the C programs in one of the CSE (cse01 cse06) servers and make sure its working. Comment your code. Create a Makefile and
Instructions: Compile the C programs in one of the CSE (cse01 cse06) servers and make sure its working. Comment your code. Create a Makefile and a readme file that describes the working and usage of the code.
Objective: Simulate TCP 3-way handshake and closing a TCP connection at the application layer using a client-server architecture.
Requirements: 1. Create a C based client-server architecture using TCP sockets
Procedure: 1. Create a C-based server that can runs on cse01.cse.unt.edu and accepts single clients request on using sockets 2. Create a C-based client that runs on cse02.cse.unt.edu and connects to the server 3. On the client side, create a TCP segment as shown in Figure 1 with C structure that has the following fields a. 16-bit source and destination port [Type: unsigned short int] b. 32-bit sequence number [Type: unsigned int] c. 32-bit acknowledgement number [Type: unsigned int] d. 4-bit data offset or header length (in multiples of 32-bit) [Type: unsigned short int for combined fields of d, e, and f] e. 6-bit reserved section
2. The server should run on cse01.cse.unt.edu machine and the client should run on cse02.cse.unt.edu machine
3. The client should be able to communicate with the server using TCP segments
4. The client should be able to demonstrate a 3-way TCP handshake with the server
5. The client should be able to demonstrate closing a TCP connection.
Procedure: 1. Create a C-based server that can runs on cse01.cse.unt.edu and accepts single clients request on using sockets
2. Create a C-based client that runs on cse02.cse.unt.edu and connects to the server
3. On the client side, create a TCP segment as shown in Figure 1 with C structure that has the following fields
a. 16-bit source and destination port [Type: unsigned short int]
b. 32-bit sequence number [Type: unsigned int]
c. 32-bit acknowledgement number [Type: unsigned int]
d. 4-bit data offset or header length (in multiples of 32-bit) [Type: unsigned short int for combined fields of d, e, and f]
e. 6-bit reserved section
f. 6-bit flags g. 16-bit receive window for flow control [Type: unsigned short int, set to zero]
h. 16-bit checksum [Type: unsigned short int, computed after the header and data is populated]
i. 16-bit urgent data pointer [Type: unsigned short int, set to zero]
j. 32-bit Options [Type: unsigned int, set to zero]
4. The variable length payload or the data field is assumed it to be of 0 bytes, as we are going to demonstrate TCP 3-way handshaking and closing a TCP connection
5. Find the source port, destination port, and header length and populate the corresponding fields of all the client and server TCP segments created below
6. Demonstrate TCP handshaking by following the below steps. Refer to Figure 2.
a. Create a connection request TCP segment as follows
i. Assign a random initial client sequence number and acknowledgement number as zero
ii. Set the SYN bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
b. The server responds to the request by creating a connection granted TCP segment. Create a connection granted response as follows
i. Assign a random initial server sequence number and acknowledgement number equal to initial client sequence number + 1
ii. Set the SYN bit and ACK bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
c. The client responds back with an acknowledgement TCP segment. Create an acknowledgement TCP segment as follows
i. Assign a sequence number as initial client sequence number + 1 and acknowledgement number equal to initial server sequence number + 1
ii. Set the ACK bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
7. Demonstrate closing of a TCP connection by following the below steps. Refer to Figure 3.
a. Create a close request TCP segment as follows
i. Assign a 1024 as client sequence number and acknowledgement number as 512
ii. Set the FIN bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
b. The server responds back with an acknowledgment TCP segment. Create an acknowledgement TCP segment as follows
i. Assign 512 as server sequence number and acknowledgement number equal to client sequence number + 1
ii. Set the ACK bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
c. The server again sends another close acknowledgement TCP segment. Create the TCP segment as follows
i. Assign 512 as server sequence number and acknowledgement number equal to client sequence number + 1
ii. Set the ACK bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
d. The client responds back with an acknowledgement TCP segment. Create an acknowledgement TCP segment as follows
i. Assign a sequence number as client sequence number + 1 and acknowledgement number equal to server sequence number + 1
ii. Set the ACK bit to 1
iii. Compute the 16-bit checksum of the entire TCP segment and populate the checksum field
8. Assume client and server has no timeout constraints
9. Write to a file (server.out and client.out) and print to the console the TCP segments transmitted and received (on both server side and client side).
10. An example code (cksum.c) to compute the checksum is given on Blackboard.
(below)
checksum.c example
#include#include struct tcp_hdr{ unsigned short int src; unsigned short int des; unsigned int seq; unsigned int ack; unsigned short int hdr_flags; unsigned short int rec; unsigned short int cksum; unsigned short int ptr; unsigned int opt; }; int main(void) { struct tcp_hdr tcp_seg; unsigned short int cksum_arr[12]; unsigned int i,sum=0, cksum, wrap; tcp_seg.src = 65234; tcp_seg.des = 40234; tcp_seg.seq = 1; tcp_seg.ack = 2; tcp_seg.hdr_flags = 0x2333; tcp_seg.rec = 0; tcp_seg.cksum = 0; //Needs to be computed tcp_seg.ptr = 0; tcp_seg.opt = 0; memcpy(cksum_arr, &tcp_seg, 24); //Copying 24 bytes printf ("TCP Field Values "); printf("0x%04X ", tcp_seg.src); // Printing all values printf("0x%04X ", tcp_seg.des); printf("0x%08X ", tcp_seg.seq); printf("0x%08X ", tcp_seg.ack); printf("0x%04X ", tcp_seg.hdr_flags); printf("0x%04X ", tcp_seg.rec); printf("0x%04X ", tcp_seg.cksum); printf("0x%04X ", tcp_seg.ptr); printf("0x%08X ", tcp_seg.opt); printf (" 16-bit values for Checksum Calculation "); for (i=0;i<12;i++) // Compute sum { printf("0x%04X ", cksum_arr[i]); sum = sum + cksum_arr[i]; } wrap = sum >> 16; // Wrap around once sum = sum & 0x0000FFFF; sum = wrap + sum; wrap = sum >> 16; // Wrap around once more sum = sum & 0x0000FFFF; cksum = wrap + sum; printf(" Sum Value: 0x%04X ", cksum); /* XOR the sum for checksum */ printf(" Checksum Value: 0x%04X ", (0xFFFF^cksum));
Step by Step Solution
There are 3 Steps involved in it
Step: 1
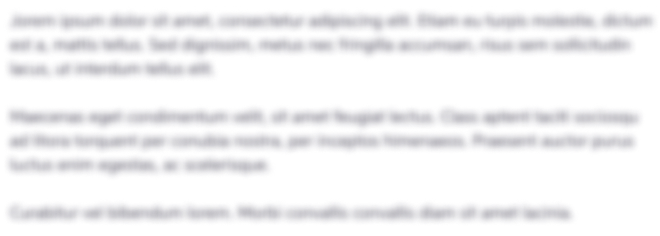
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started