****instructions: please implement the functions listed in node.h and clinkedlist.h in order to get main to work. ****
(C++)
#include
using namespace std;
#include "clinkedlist.h"
// global constants
const int MAX_ITEMS = 4;
// main
int main()
{
CLinkedList list;
int numToAdd[MAX_ITEMS] = {10, 12, 15, 5};
// The following steps will display, add, and remove items in the list to
// test all the functions.
// 1) Display an empty list
cout
cout
list.DisplayListAscending();
// 2) Clear an empty list
cout
if (!list.Clear())
{
cerr
}
// 3) Add some numbers
cout
for (int i = 0; i
{
cout
if (!list.Add(numToAdd[i]))
{
cerr
}
}
// 4) Display the current list in ascending and descending order
cout
cout
list.DisplayListAscending();
cout
list.DisplayListDescending();
// 5) Delete the first item value (5 and notice how the items get rearranged
cout
if (!list.Remove(5))
{
cerr
}
// 6) Display list
cout
list.DisplayListAscending();
// 7) Delete a middle item value (12 and notice how the items get rearrange
cout
if (!list.Remove(12))
{
cerr
}
// 8) Display list
cout
list.DisplayListAscending();
// 9) Delete the last item (value 15 and notice how the items get rearrange
cout
if (!list.Remove(15))
{
cerr
}
// 10) Display list
cout
list.DisplayListAscending();
// 11) Check if item value 333 exists in the list
cout
if (!list.Contains(333))
{
cerr
}
// 12) Delete item value 333, which is not in the list
cout
if (!list.Remove(333))
{
cerr
}
// 13) Clear the list
cout
if (!list.Clear())
{
cerr
}
// 14) Display list
cout
list.DisplayListAscending();
return 0;
} // end of "main"
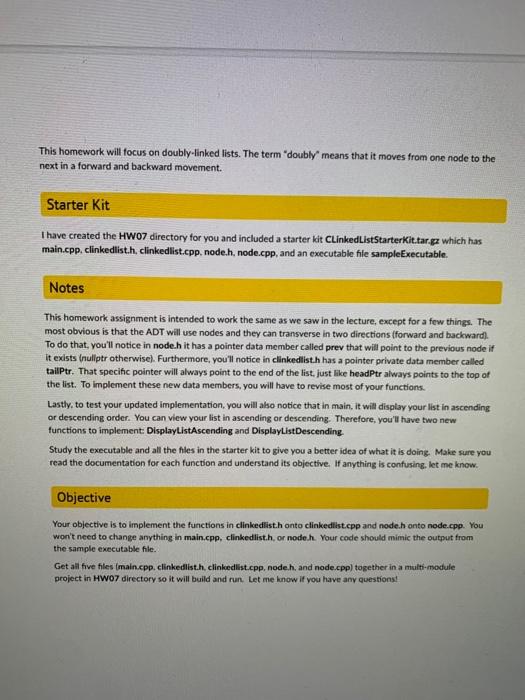
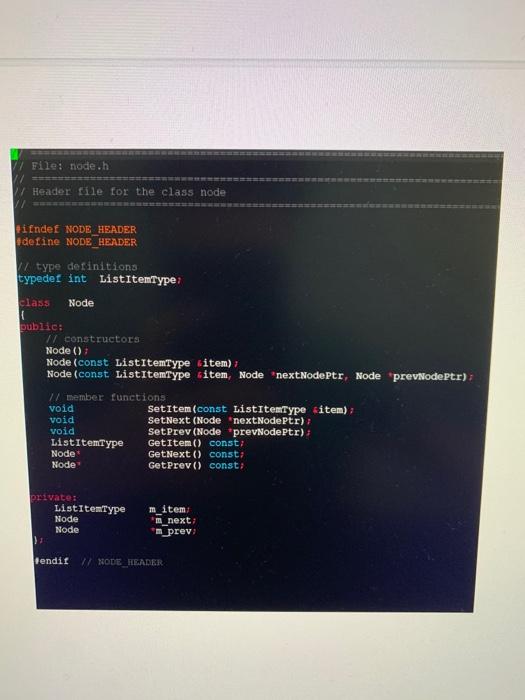
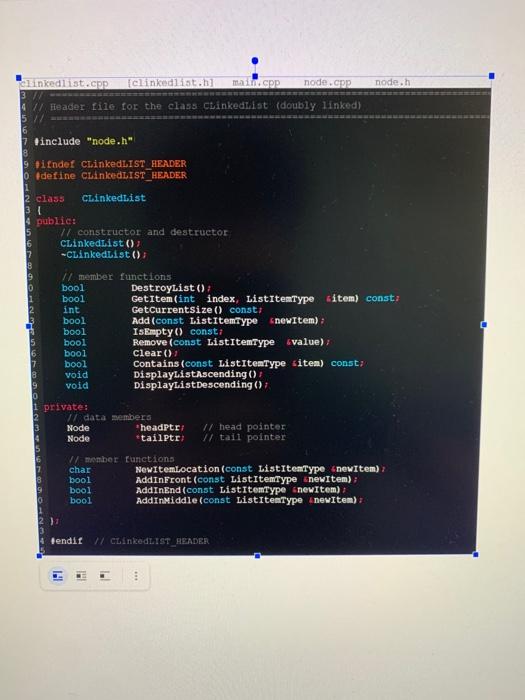
This homework will focus on doubly linked lists. The term "doubly means that it moves from one node to the next in a forward and backward movement. Starter Kit I have created the HW07 directory for you and included a starter kit CLinkedListStarterkit.tar.gz which has main.cpp, clinkedlist.h, clinkedlist.cpp, node.h, node.cpp, and an executable file sampleExecutable. Notes This homework assignment is intended to work the same as we saw in the lecture, except for a few things. The most obvious is that the ADT will use nodes and they can transverse in two directions (forward and backward). To do that, you'll notice in node.h it has a pointer data member called prev that will point to the previous node if It exists (nullotr otherwise). Furthermore, you'll notice in clinkedlist.h has a pointer private data member called tailPtr. That specific pointer will always point to the end of the list, just like headPtr always points to the top of the list. To implement these new data members, you will have to revise most of your functions. Lastly, to test your updated implementation, you will also notice that in main, it will display your list in ascending or descending order. You can view your list in ascending or descending. Therefore, you'll have two new functions to implement: DisplayListAscending and DisplayListDescending Study the executable and all the files in the starter kit to give you a better idea of what it is doing. Make sure you read the documentation for each function and understand its objective. If anything is confusing, let me know. Objective Your objective is to implement the functions in clinkedlist.h onto clinkedlist.cpp and node.h onto node.cpp. You won't need to change anything in main.cpp, clinkedlist.h. or node.h. Your code should mimic the output from the sample executable file. Get all five files (main.cpp.clinkedlist.h, clinkedlist.cpp nodeh, and node.cpp) together in a multi-module project in HW07 directory so it will build and run. Let me know if you have any questions! 17 File: node.h 1/ Header file for the class node 17 Hifndef NODE_HEADER define NODE_HEADER 1 type definitions typedef int ListItemType class Node C public: // constructors Node(); Node (const ListItemType item) Node (const ListItemType citem Node "nextNodeptr, Node "previodeptr); // member functions void SetItem(const ListItemType item) void SetNext (Node "nextNode Ptr); void SetPrev (Node prevNodeptr): ListItemType GetItem() consti Node GetNext() const; Node GetPrev() const: private: ListItemType Node Node ) m_item m_next m_prev: tendit // NODE HEADER node.h alinkedlist.cpp felinkedlist.nl main.cpp node.cop // Header file for the class CLinkedlist (doubly linked) 5 // ww tinclude "node.h" 9 fifndef ClinkedLIST HEADER O define clinkedLIST HEADER 2 class CLinkedlist 3 4 public: V/ constructor and destructor CLinkedlist(); -CLinkedlist(); // member functions bool DestroyList(); bool GetItem(int index, ListItemType (item) const: int GetCurrentsize() const: bool Add(const ListItemtype newItem): bool IsEmpty() consti bool Remove (const ListItemType evalue) bool clear() bool Contains (const ListItemType item) const: void DisplayListAscending(); void DisplayList Descending(); 19 0 private: data members Node headptr Node tailptr 1/ head pointer v/ tail pointer V member functions char NewItemLocation (const ListItemType