Question
Instructions The calendar will be 7 cells wide. Each cell is boarded by a vertical bar | and six spaces wide not counting the vertical
Instructions
The calendar will be 7 cells wide. Each cell is boarded by a vertical bar "|" and six spaces wide not counting the vertical bars. The top row contains the abbreviations for the days, (i.e., Sun, Mon, Tue, Wed, Thu, Fri, Sat.) The days names will be centered above each cell. Below the day names there will be a boarder made up of dashes "-"with a plus "+" over each cell boarder. The days numbers will be left padded so that the least significant number lines up under the last letter of the day name.
Padding
One tricky part of this program is making the various columns line up properly with proper widths. We will learn better ways of formatting output in the next chapter. For now, you may copy the following helper method into your program and call it to turn a number into a left-padded string of a given exact width. For example the call System.out.print(padded(7, 5)); prints " 7" (the number 7 with four leading spaces).
/** * Returns a string of the number n, left padded with spaces * until it is at least the given width. * * @param n the number to be padded into the string. * @param width the width of the pad. * @return a padded string. */ publicstatic String padded(int n,int width ) { String s ="" + n; for (int i = s.length(); i < width; i++ ) { s =" " + s; } return s; }
Example
A transcript of the program should look like the example provided below.
How many days are in the month? 31 What day is the first Sunday? M2 The month has 31 and the first Sunday is 2 M Sun Mon Tue Wed Thu Fri Sat M+------+------+------+------+------+------+------+ M| | | | | | | 1 | M| 2| 3 | 4 | 5| 6 | 7 | 8 | M| 9| 10 |11 | 12| 13 |14 | 15 | M| 16| 17 |18 | 19| 20 |21 | 22 | M| 23| 24 |25 | 26| 27 |28 | 29 | M| 30| 31 | | | | | | M+------+------+------+------+------+------+------+
Comments
JavaDoc comments will be used to document the class and all methods. A JavaDoc comment is opened with a forward slash and 2 asterisks, and it is closed with an asterisk and a forward slash, (i.e., /** */). The first line a brief description which is usually followed by a black line and then a longer description. JavaDoc comments may contain tags. Tags are introduced with each symbol, (i.e., @.)This program will use the @author, @param, and @return tags.
/** * Estimate PI by dividing the area of circle by the square of the radius. * */ publicclass AppoximatePi { /** * Main method for the ApproximatePi program. * * @param args an array of command line arguments */ publicstaticvoid main(String[] args) { double r = 0.0; for (int i = 1; i <= 10; i++) { r = Math.pow(10,i); double pi = (2.0 * halfArea( r )) / (r * r); System.out.println( "At a radius of " + r + "\tthe estimate for PI is " + pi + " with a difference of " + Math.abs( Math.PI - pi ) ); } } /** * Find the positive value of y based upon the value of x and the radius. * * @param x the position on the x axis. * @param r the radius of the circle. * @return the positive value of y for x with a circle centered at the origin and a radius of r. */ publicstaticdouble findY(double x,double r ) { double y = Math.sqrt(r * r - x * x); return y; } /** * Compute the half area of a circle by summing the rectangles with a width of one. * * @param r the radius of the circle. * @return half of the area of circle with a radius of r */ publicstaticdouble halfArea(double r ) { double a = 0.0; for (double x = -r; x <= r; x++) { double y = findY( x, r ); a += y; } return a; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
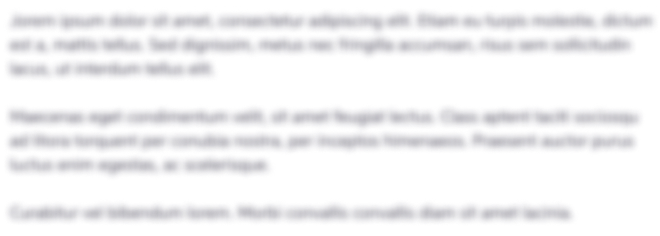
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started