Question
Instructions Using the BCException.java and the BankCustomer.java from the first part of the assignment you will implement a driver class called Bank.java to manipulate an
Instructions
Using the BCException.java and the BankCustomer.java from the first part of the assignment you will implement a driver class called Bank.java to manipulate an ArrayList of BankCustomer objects.
Your code should read bank customers information from the user. It will then create a bank customer objects and add them to an arraylist in a sorted fashion (sorted by account number). The arraylist should be sorted in ascending order at all times. The arraylist should not contain null objects at any point. You may NOT add a BankCustomer and then sort the arraylist, you should insert the new element in the appropriate position. The arraylist should not contain duplicate bank customers. For the purpose of this assignments duplicate bank customers are those that have the same account number.
When removing a bank customer you should find the customer using the account number. You will search for the account number and if found, delete the customer from the arraylist. If not found you should display a message saying so and go back to the main menu.
Printing all the bank customers should be done in ascending order, which is the same way that the arraylist is sorted.
Bank.java should not end execution if an exception happens. You should handle exceptions gracefully. Execution should end only when the user decides to exit by selecting 0 from the menu. (50 points)
Your code should display the following menu:
1. Add a bank customer (50 points)
2. Remove a bank customer (50 points)
3. Print all bank customers (50 points)
0. Exit
The program should end only when the user selects 0 to exit, otherwise the menu should continue to loop executing options as selected by the user. Feel free to use any extra helper/static methods to make your code more efficient and legible. Not required, but you may want to do it for extra credit, as extra credit is given for well-written code.
You should submit the driver class as well as your BankCustomer.java and BCException.java (again) (40 points). If previously, your code allowed for the creation of invalid objects you must review and fix your code or you will get deductions in this assignment as well.
Below is my BankCustomer.java and BCException.java
BankCustomer.java
public class BankCustomer {
private int acctNumber; private double balance; private String name;
public BankCustomer(int acctNumber, double balance, String name) throws Exception{ this.setAcctNumber(acctNumber); this.setBalance(balance); this.setName(name); } public BankCustomer(){ this.acctNumber = 10001; this.balance = 120.0; this.name = "sample"; } public void setAcctNumber(int acctNumber) throws Exception { if (acctNumber >= 10001 && acctNumber <= 99999){ this.acctNumber = acctNumber; } else{ BCException me = new BCException(); me.setMessage("The account number passed " + acctNumber + " is not valid"); throw (me); } } public void setBalance(double balance) throws Exception { if (balance >= 100){ this.balance = balance; } else { BCException me = new BCException(); me.setMessage("The amount added " + balance + " should be more than 100"); throw (me); } } public void setName(String name) throws Exception { if (name.trim().length() >= 5){ this.name = name.trim(); } else { BCException me = new BCException(); me.setMessage("The name " + name + " should be atleast 5 non-blank characters"); throw (me); } } public int getAcctNumber() { return acctNumber; }
public double getBalance() { return balance; }
public String getName() { return name; } }
BCException.java
public class BCException extends Exception{ //one instance variable private String message; public void setMessage(String message){ this.message = message; } public String getMessage(){ return this.message; } }
Please help me create a driver class plus the array requirement that is needed based on the instruction given plus " You should submit the driver class as well as your BankCustomer.java and BCException.java (again) (40 points). If previously, your code allowed for the creation of invalid objects you must review and fix your code or you will get deductions in this assignment as well. " If there is something wrong with my BankCustomer.java or BCException.java please point it out and help me fix it.
Thanks again for helping.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
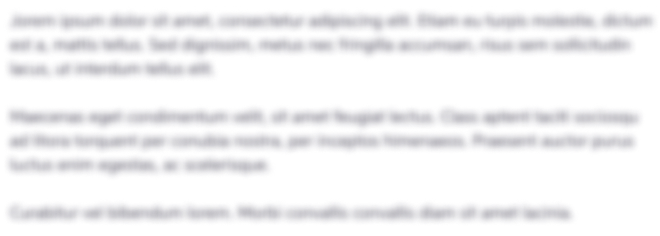
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started