Answered step by step
Verified Expert Solution
Question
1 Approved Answer
interval _ map is a data structure that associates keys of type K with values of type V . It is designed to be used
intervalmap is a data structure that associates keys of type K with values of type V It is designed to be used efficiently in situations where intervals of consecutive keys are associated with the same value. Your task is to implement the assign member function of this data structure, which is outlined below. intervalmap is implemented on top of std::map. For more information on std::map, you may refer to cppreference.com. Each keyvaluepair kv in intervalmap::mmap means that the value v is associated with all keys from k including to the next key excluding in mmap. The member intervalmap::mvalBegin holds the value that is associated with all keys less than the first key in mmap. Example: Let M be an instance of intervalmap where MmvalBeginA MmmapBA Then M represents the mapping A A A B B A A A The representation in the std::map must be canonical, that is consecutive map entries must not contain the same value: AA is not allowed. Likewise, the first entry in mmap must not contain the same value as mvalBegin. Initially, the whole range of K is associated with a given initial value, passed to the constructor of the intervalmap data structure. Key type K besides being copyable and assignable, is lessthan comparable via operator and does not implement any other operations, in particular no equality comparison or arithmetic operators. Value type V besides being copyable and assignable, is equalitycomparable via operator and does not implement any other operations. Your task is to implement the function assign. Your implementation is graded by the following criteria in this order: Type requirements are met: You must adhere to the specification of the key and value type given above. Correctness: Your program should produce a working intervalmap with the behavior described above. In particular, pay attention to the validity of iterators. It is illegal to dereference end iterators. Consider using a checking STL implementation such as the one shipped with Visual C or GCC Canonicity: The representation in mmap must be canonical. Running time: Imagine your implementation is part of a library, so it should be bigO optimal. In addition: Do not make bigO more operations on K and V than necessary because you do not know how fast operations on KV are; remember that constructions, destructions and assignments are operations as well. Do not make more than one operation of amortized Olog N in contrast to O running time, where N is the number of elements in mmap. Otherwise favor simplicity over minor speed improvements. My code thus far!!! Please help in C I keep failing requirement All other requirements are working! Type requirements are met: You must adhere to the specification of the key and value type given above. Code: #include #include template class intervalmap friend void IntervalMapTest; V mvalBegin; std::map mmap; public: Constructor associates the whole range of K with val intervalmapV const& val : mvalBeginval Assign value val to the interval keyBegin keyEnd void assignK const& keyBegin, K const& keyEnd, V const& val if keyBegin keyEnd return; empty interval, do nothing auto itLow mmap.lowerboundkeyBegin; if itLow mmap.begin auto itPrev std::previtLow; if itPrevsecond val itLow mmap.eraseitLow; auto itHigh mmap.lowerboundkeyEnd; if itLow itHigh mmap.eraseitLow itHigh; mmapkeyEnditHigh mmap.end itHighsecond : mvalBegin; if mvalBegin val itLow mmap.begin && keyBegin mmap.beginfirst mmapkeyBegin val; if keyBegin mmap.beginfirst && mvalBegin val mmap.erasemmap.begin; Lookup the value associated with key V const& operatorK const& key const auto it mmap.upperboundkey; if it mmap.begin return mvalBegin; else return itsecond; ; int main intervalmap MA; MassignB; MassignC; for int i ; i ; i std::cout Mi std::endl; return ;
intervalmap is a data structure that associates keys of type K with values of type V It is designed to be used efficiently in situations where intervals of consecutive keys are associated with the same value. Your task is to implement the assign member function of this data structure, which is outlined below.
intervalmap is implemented on top of std::map. For more information on std::map, you may refer to cppreference.com.
Each keyvaluepair kv in intervalmap::mmap means that the value v is associated with all keys from k including to the next key excluding in mmap. The member intervalmap::mvalBegin holds the value that is associated with all keys less than the first key in mmap.
Example: Let M be an instance of intervalmap where
MmvalBeginA
MmmapBA
Then M represents the mapping
A
A
A
B
B
A
A
A
The representation in the std::map must be canonical, that is consecutive map entries must not contain the same value: AA is not allowed. Likewise, the first entry in mmap must not contain the same value as mvalBegin. Initially, the whole range of K is associated with a given initial value, passed to the constructor of the intervalmap data structure.
Key type K
besides being copyable and assignable, is lessthan comparable via operator and
does not implement any other operations, in particular no equality comparison or arithmetic operators.
Value type V
besides being copyable and assignable, is equalitycomparable via operator and
does not implement any other operations.
Your task is to implement the function assign. Your implementation is graded by the following criteria in this order:
Type requirements are met: You must adhere to the specification of the key and value type given above.
Correctness: Your program should produce a working intervalmap with the behavior described above. In particular, pay attention to the validity of iterators. It is illegal to dereference end iterators. Consider using a checking STL implementation such as the one shipped with Visual C or GCC
Canonicity: The representation in mmap must be canonical.
Running time: Imagine your implementation is part of a library, so it should be bigO optimal. In addition:
Do not make bigO more operations on K and V than necessary because you do not know how fast operations on KV are; remember that constructions, destructions and assignments are operations as well.
Do not make more than one operation of amortized Olog N in contrast to O running time, where N is the number of elements in mmap.
Otherwise favor simplicity over minor speed improvements.
My code thus far!!! Please help in C I keep failing requirement All other requirements are working!
Type requirements are met: You must adhere to the specification of the key and value type given above.
Code:
#include
#include
template
class intervalmap
friend void IntervalMapTest;
V mvalBegin;
std::map mmap;
public:
Constructor associates the whole range of K with val
intervalmapV const& val : mvalBeginval
Assign value val to the interval keyBegin keyEnd
void assignK const& keyBegin, K const& keyEnd, V const& val
if keyBegin keyEnd
return; empty interval, do nothing
auto itLow mmap.lowerboundkeyBegin;
if itLow mmap.begin
auto itPrev std::previtLow;
if itPrevsecond val
itLow mmap.eraseitLow;
auto itHigh mmap.lowerboundkeyEnd;
if itLow itHigh
mmap.eraseitLow itHigh;
mmapkeyEnditHigh mmap.end itHighsecond : mvalBegin;
if mvalBegin val itLow mmap.begin && keyBegin mmap.beginfirst
mmapkeyBegin val;
if keyBegin mmap.beginfirst && mvalBegin val
mmap.erasemmap.begin;
Lookup the value associated with key
V const& operatorK const& key const
auto it mmap.upperboundkey;
if it mmap.begin
return mvalBegin;
else
return itsecond;
;
int main
intervalmap MA;
MassignB;
MassignC;
for int i ; i ; i
std::cout Mi std::endl;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
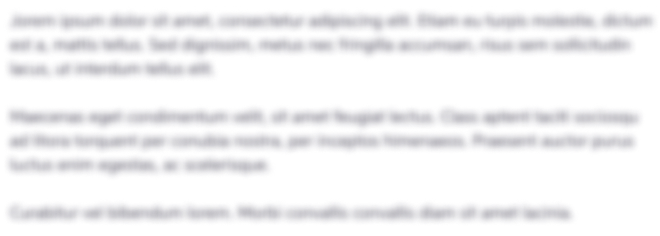
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started