Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Intro to SE ( ISAD 1 0 0 0 / 5 0 0 4 ) Worksheet 8 : White - Box Testing and Test Fixtures
Intro to SE ISAD Worksheet : WhiteBox Testing and Test Fixtures Java
CRICOS Provide Code: J Page of
public static void printCoordinatesdouble x double y double z
System.out.printffff
x y z;
Worksheet : WhiteBox Testing and Test Fixtures
Java
Updated: th May, th October
There are two versions of this worksheet. This is the Java version.
In this worksheet, youll practice whitebox test design, and the settingup and tearingdown
of test fixtures. You may refer lecture and both when doing this worksheet There are a
range of production code methods for you to implement test code for. The code is shown
below, and is also available in Utils.java.
For each production code method, do the following:
a Design your test cases:
Identify the paths through the production code.
Select test data for each test case. In other words, for each path, select inputs parameters console input, andor input files that will cause the production code to
follow that path.
For each test case, determine the expected results. This includes return values,
exceptions thrown, console output, and output files.
b Implement yourtest cases.
Its good experience to continue to use JUnit, although you can still perform the exercise
without it
c Run yourtests against the production code.
To ensure that your test code is working, its helpful to temporarily break the production
code. You could do this by editing the production code to alter the outputresults very
slightly.
printCoordinates
printCoordinates takes in x y and z coordinates, and prints them out in the formatx y z
with two decimal places each. The output will end in a new line.
This is a trivial case for test design. Since there are no conditional statements, there is only
one path, and hence one test case.
Intro to SE ISAD Worksheet : WhiteBox Testing and Test Fixtures Java
CRICOS Provide Code: J Page of
Warning: Be very careful with code that deletes files! Ensure the file you specify is
definitely the one you want to delete.
public static char readCharString validChars
Scanner sc new ScannerSystemin;
String line scnextLine;
whilelinelengthvalidChars.containsline
line scnextLine;
return line.charAt;
Note: Tearingdown console inputredirection in Java is much the same as for output:
import java.io;
InputStream originalIn System.in; Save original input
System.setIn; Redirect input
Do testing
System.setInoriginalIn; Restore input
readChar
readChar reads a single valid character from the user. The user enters a character, but
must reenter their input if its invalid; ie if the character they enter does not occur within the
validChars parameter.
Hint: there are two paths, and hence two test cases here.
Note: Rememberto tear down everything that you set up Specifically, to restore console output in Java, you must save a copy of the original, like this:
To delete temporary files:
import java.io;
new Filesomefiletxtdelete;
import java.io;
PrintStream originalOut System.out; Save original output
System.setOut; Redirect output
Do testing
System.setOutoriginalOut; Restore output
Intro to SE ISAD Worksheet : WhiteBox Testing and Test Fixtures Java
CRICOS Provide Code: J Page of
public static void guessingGameint number
Scanner sc new ScannerSystemin;
System.out.printEnter an integer: ;
int guess scnextInt;
whileguess number
ifguess number
System.out.printlnToo high.";
else
System.out.printlnToo low.";
System.out.printEnter an integer: ;
guess scnextInt;
System.out.printlnCorrect;
public static double sumFileString filename
double sum ;
try
Scanner sc new Scannernew Filefilename; Throws IOException
whileschasNextDouble
sum scnextDouble;
guessingGame
guessingGame runs a consolebased number guessing game. The user is repeatedly asked to
guess what the number is and is told whether their guess is too high, too low, or correct at
which point the game ends
Hint: the result of guessingGame consists of all the console output, including the prompt asking for input. Also note that println will print a newline character, while print wont
This will be important when determining the expected output.
sumFile
sumFile: opens a file assumed to contain a list of numbers and adds up the numbers, and
returns the total. If the file could not be opened, it returns instead.
Hint: the empty string is an invalid filename that, by definition, cannot be opened.
Intro to SE ISAD Worksheet : WhiteBox Testing and Test Fixtures Java
CRICOS Provide Code: J Page of
If using
Step by Step Solution
There are 3 Steps involved in it
Step: 1
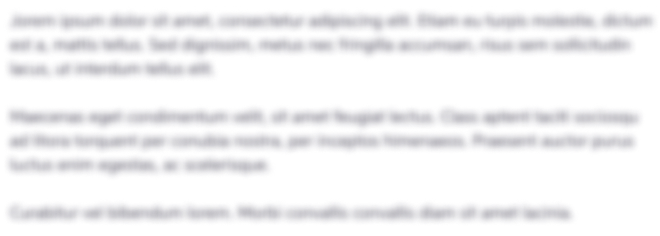
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started