Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Introduction Assignment 0 6 Functional Programming Please read this entire document before beginning. In this assignment, we are going to implement a handful of common
Introduction
Assignment
Functional Programming
Please read this entire document before beginning.
In this assignment, we are going to implement a handful of common functional building blocks using our Scheme interpreter. We will then use those building blocks to solve a simple problem.
The functions we will be writing all involve recursion, so lets review how to write a recursive function. We will use the factorial function as an example:
First, we need to establish a base case, or the set of conditions under which we can stop recursively calling the function, and return a result. For the factorial function, this is the case when n When we reach the condition n we know that we can stop recursively calling the factorial function, and just return the value
define fact lambda nif n
Next, we need to establish our recursive case. To implement the recursive case, we use a simpler version of our problem, and assume that we have a function that is capable of solving that simpler version. It is important that this simpler version of our problem gets us closer to our base case.
For our n factorial problem, our recursive case involves the simpler problem of finding n and then multiplying the result by n Note that for any positive value of n subtracting one from n will get us closer to our base case of n
We assume the existence of a function simplefact capable of solving the simpler version of our problem:
define fact lambda nif n simplefact n n Finally, we replace the call to simplefact with a call to fact, and we have our implementation:
define fact lambda nif n fact n n
You should follow these same steps for the functions we will be writing in Scheme in this assignment.
text file called core.scm to your srcmainresources directory. We will use this file to store the definitions of our new Scheme functions.
Finally, you need to add the tests for this assignment. On Brightspace, attached to this assignment, you will find a file named IntegrationTest.java. Download this file and copy it into your local repositorys srctestjavacadalcsci directory. Then commit and push the updates to GitLab.
Now you should be ready to begin tackling this assignment.
The Assignment
In this assignment, we are going to write subroutines in Scheme, and have our interpreter load those subroutines when it starts up so that they will be available in the REPL just like the procedures we wrote in the last three assignments. We will then use those subroutines to solve a simple problem.
Update the REPL
The first step in this assignment is to update the Main class to load the contents of core.scm so that any subroutines defined there can be available in our readevaluateprint loop. If you look in the testEval function of the IntegrationTest class, you will find an easy way to load this file upon startup. Simply load the entire file as a String, tokenize it Parse the resulting sequence of tokens into abstract syntax trees, and evaluate them. Be sure to hold on to the referencing environment between evaluations since we expect those evaluations to add new definitions.
The range Function mark
The range function accepts three integer arguments: start, stop, step and returns a list containing all the integers from start inclusive and stop exclusive incrementing by step. You can assume that the step argument will never be zero.
range
range
range
range
The filter Function
mark
The filter function accepts two arguments; the first is a predicate and the second is a list. The filter function applies the predicate to each element of the list, and returns the sublist of elements for which the predicate returns true.
filter list? no yes
Symbolname'yes'
filter lambda x #tthe quick brown foxSymbolname'the' Symbolname'quick' Symbolname'brown' Symbolname'fox'
filter lambda x #fjumps over the lazy dog
filter lambda x x z #f #t
Symbolnamez true
filter lambda xremainder x range
The foldl Function mark
The foldl or foldleft function combines the elements of a list using a binary operator starting with some initial value. It has the form:
foldl op init l For example:
foldl
Here, the foldl function computes resulting in It starts by using the operator to combine the first element of the list and the initial value Then it combines the result of that operation with and so on
Another example:
foldl cons
Step by Step Solution
There are 3 Steps involved in it
Step: 1
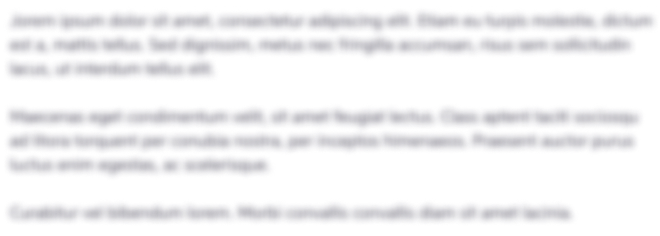
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started