Question
Introduction The aim of this homework is to practice on file operations and dictionaries. In addition, please try to utilize functions since they would reduce
Introduction
The aim of this homework is to practice on file operations and dictionaries. In addition, please try to utilize functions since they would reduce the complexity of your program.
Description
You are given a file named "turkiye_spotify_data.txt", which stores the daily ranking of top 200 songs listened in Turkiye by Spotify users between 1st January 2017 to 9th January 2018 (including both). Your task is to develop a Python program which, given a year and a month, recommends that month's top hits in sequence until the song the user wants to hear is found.
In your program, the user will provide two inputs: year and month. Herein, the year must be either 2017 or 2018, and the month must be between 1 and 12, if the year is 2017; and only 1, if the year is 2018. Your program should make sure that the inputs provided obey the rules mentioned. Your code will then process the given Spotify data to find the song with the most streams in the specified year and month.
As your program suggests songs to its user, it will also ask whether they want to listen to this song or not. When the answer is yes, your program should share the url of the song and finish afterwards. Otherwise, your program should continue suggesting upcoming songs based on the streaming number in the asked month, until the user decides to listen. Please see the "Sample Runs" section for better understanding on the flow of the program, the inputs and the outputs.
Input File
You will be given only one input file sample. The name of this input file will be turkiye_spotify_data.txt.
This file contains information about the songs on Spotify, including the song's position on the chart, the track name, artist, number of streams, URL, release date, and region.
Each line of this file represents a different song, and the data for each song is separated by a horizontal tab character ("\t").
You can assume that the file information will be given in the correct format. Thus, you don't have to perform any format check on the file content. You can also assume that there are no duplications and no empty lines in the file. However, you cannot make any assumptions on the number of lines of this file. Keep this in mind while you're preprocessing the content of the file. THE FOLLOWING RED PART WAS ADDED LATER, PLEASE CHECK IT OUT Note that there can be different versions of some songs with the same name (but with a different url). For instance "Cheap Thrills" from "Sia" has two different versions in January 2017 with the following links: 'https://open.spotify.com/track/27SdWb2rFzO6GWiYDBTD9j' 'https://open.spotify.com/track/378iszndTZAR4dH8kwsLC6' Please consider them as the same song, and consider their both streaming counts, but the link for this song should be the first link that appears on this month. So, the track name + artist name together should be unique. And the link for 'Cheap Thrills, Sia' in January 2017 should be the following one: 'https://open.spotify.com/track/27SdWb2rFzO6GWiYDBTD9j'
Input and Output
The inputs of the program and their order are explained below. It is extremely important to follow this order since we automatically process your programs. Thus your work will be graded as 0 unless the order is entirely correct. Please see the "Sample Runs" section for some examples.
The prompts of the input statements to be used has to be exactly the same as the prompts of the "Sample Runs".
Do not change the file name. It should be "turkiye_spotify_data.txt". While trying your code on CodeRunner, do not provide any path to open the file. You just need to execute the following command: open("turkiye_spotify_data.txt")
Your program should display the following prompt to get the year input from the user.
Enter the year:
If the user enters an invalid input, then your program should keep asking till a valid one is entered.
The valid inputs are 2017 and 2018.
You can assume that the year value will be entered as an integer.
After you get the correct input for the year, then your program should display the following prompt to get the month from the user.
Enter the month:
If the user enters an invalid month, then your program should keep asking till a valid one is entered.Valid inputs are
numerics between 1 and 12, when the year is 2017,
only 1, when the year is 2018.
You can assume that the month value will be entered as an integer.
Once the year and the month information is received properly, your program should suggest the most streamed song of the date entered and ask the user if they want to listen to this song. Your program should keep asking this way until the user wants to listen to the song. The format your program should use to display the results must be exactly as follows:
NEW SUGGESTION: SongName, ArtistName (Total stream number in this month: NumberOfStreams)
Do you want to listen this song (enter either yes or no):
If the answer is "yes", the output should be:
Enjoy SongName, ArtistName. Here is the url for you: Url
"yes" and "no" answers should be case insensitive, and if any input other than these answers is entered, your program should ask the question again until a correct/valid input is entered by the user.
If the answer is "no", then your program should suggest the upcoming most streamed song. You can assume that the streaming numbers within each month are different for each song. And also you may assume that the user will enter "yes" at some point before we consume all songs streamed in this month.
Please see the "Sample Runs" section for examples.
Sample Runs
Below, we provide some sample runs of the program that you will develop. The italic and bold phrases are inputs taken from the user. You have to display the required information in the same order and with the same words and characters as below.
Sample Run 1
Enter the year: 2017
Enter the month: 11
NEW SUGGESTION: Etei Belinde, Manu Baba (Total stream number in this month: 1671410)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: rockstar, Post Malone (Total stream number in this month: 1531063)
Do you want to listen this song (enter either yes or no): yes
Enjoy rockstar, Post Malone. Here is the url for you: https://open.spotify.com/track/7wGoVu4Dady5GV0Sv4UIsx
Sample Run 2
Enter the year: 2023
Enter the year: 2016
Enter the year: 2017
Enter the month: 6
NEW SUGGESTION: Despacito - Remix, Luis Fonsi (Total stream number in this month: 1665129)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Shape of You, Ed Sheeran (Total stream number in this month: 1455589)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Attention, Charlie Puth (Total stream number in this month: 1094736)
Do you want to listen this song (enter either yes or no): yes
Enjoy Attention, Charlie Puth. Here is the url for you: https://open.spotify.com/track/4iLqG9SeJSnt0cSPICSjxv
Sample Run 3
Enter the year: 2018
Enter the month: 7
Enter the month: 4
Enter the month: 1
NEW SUGGESTION: Yanyoruz, Burak King (Total stream number in this month: 790051)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Heyecan Yok, Gazapizm (Total stream number in this month: 675705)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Gmn Beni ukura, Eypio (Total stream number in this month: 560320)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: rockstar, Post Malone (Total stream number in this month: 536499)
Do you want to listen this song (enter either yes or no): yes
Enjoy rockstar, Post Malone. Here is the url for you: https://open.spotify.com/track/7wGoVu4Dady5GV0Sv4UIsx
Sample Run 4
Enter the year: 2017
Enter the month: 2
NEW SUGGESTION: Shape of You, Ed Sheeran (Total stream number in this month: 2050088)
Do you want to listen this song (enter either yes or no): nr
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Deli, Norm Ender (Total stream number in this month: 1084866)
Do you want to listen this song (enter either yes or no): yes
Enjoy Deli, Norm Ender. Here is the url for you: https://open.spotify.com/track/2BZn6a1rAFnKWmD4McsIAn
Sample Run 5
Enter the year: 2017
Enter the month: 4
NEW SUGGESTION: Shape of You, Ed Sheeran (Total stream number in this month: 2144459)
Do you want to listen this song (enter either yes or no): YES
Enjoy Shape of You, Ed Sheeran. Here is the url for you: https://open.spotify.com/track/7qiZfU4dY1lWllzX7mPBI3
Sample Run 6
Enter the year: 2018
Enter the month: 1
NEW SUGGESTION: Yanyoruz, Burak King (Total stream number in this month: 790051)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Heyecan Yok, Gazapizm (Total stream number in this month: 675705)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Gmn Beni ukura, Eypio (Total stream number in this month: 560320)
Do you want to listen this song (enter either yes or no): o
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: rockstar, Post Malone (Total stream number in this month: 536499)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: ki Ak, Ersay ner (Total stream number in this month: 514589)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: mkanszm, Ezhel (Total stream number in this month: 478214)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Zalm, Ceylan Ertem (Total stream number in this month: 452216)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Geceler, Ezhel (Total stream number in this month: 433961)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Havana, Camila Cabello (Total stream number in this month: 423176)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: New Rules, Dua Lipa (Total stream number in this month: 406164)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: ehrimin Tad, Ezhel (Total stream number in this month: 380631)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: zlmedin mi?, Simge (Total stream number in this month: 358308)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Him & I (with Halsey), G-Eazy (Total stream number in this month: 342199)
Do you want to listen this song (enter either yes or no): no
NEW SUGGESTION: Etei Belinde, Manu Baba (Total stream number in this month: 308352)
Do you want to listen this song (enter either yes or no): yes
Enjoy Etei Belinde, Manu Baba. Here is the url for you: https://open.spotify.com/track/3lPrs1fylHXjPPfSioDXDg
Step by Step Solution
There are 3 Steps involved in it
Step: 1
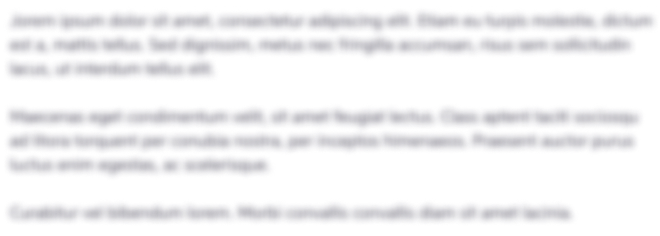
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started