Question
Introduction to Programming Introduction Throughout much of the semester we have been working with the cards program and have developed it in multiple forms. We
Introduction to Programming
Introduction
Throughout much of the semester we have been working with the cards program and have developed it in multiple forms. We shall continue this development process by refactoring the last instance of the program so as to use multiple classes.
You will write classes that model a Card, a Deck of Cards, and a Hand which holds Cards. Finally, you will write a main class, Poker, that will create a Deck of Cards, shuffle the Deck, and then deal out Cards to multiple Hands.
For the development process, we shall work from the bottom up. Please read and follow the instructions below to write the specified four classes. Please submit the four Java source code files with the names:
Card.java
Deck.java
Hand.java
Poker.java
to the Blackboard Assignment/Exam3.
class Card [20]
This class represents a single card. It maintains the cards card number. It can provide the cards suit and rank, and a human readable version of the card.
[4] Define two symbolic constants as arrays of String elements named SUITS and RANKS. These arrays will be identical to the arrays we used in the previous version of the program. Based on these arrays define additional symbolic constants names NSUITS, NRANKS, and NCARDS (the last being the product of the first two).
[3] There will be one instance field named cardNum of type int. Declare this field.
[3]Define a single constructor that accepts a card number and assigns it to the instance field.
[4] Define two accessor methods similar to the ones previously written in the older version of the program:
int rank()
int suit()
[4] Define the method String toString() that will return a String of the form: rank of suit.
[2] You may write a local main() method that you can use to unit test the correctness of this class.
class Deck [30]
This class represents a deck of cards (we assume a normal card deck without any jokers). It maintains an array of cards and keeps track of where in the deck we are while cards are being dealt. It provides for constructing a new, ordered, deck of cards, shuffling the deck, and producing a String version of the deck for display purposes (most likely for debugging).
[4] Import classes that will be needed by this class:
java.util.Random
java.util.Arrays
java.util.NoSuchElementException
Import the static fields from the Card class so that we do not need to prefix their names with the class name, Card (e.g., Card.NCARDS can be referred to by just NCARDS).
import static exam3.Card.*;
[3] Declare 3 instance fields:
Card[] deck the array to hold the cards in the deck
int nextCard the index in the deck which will be the next card to deal out
Random rand a random number generator used by shuffle (this is an instance field since we only need one generator that may be invoked multiple times if we shuffle the deck multiple times).
[7] Define a no-argument constructor.
[2] Instantiate the deck array with NCARDS elements.
[2] Set each element of the deck array with the Card element whose cardNum matches its index in the deck.
[2] Set nextCard to 0 to indicate that we will start dealing from the top of the deck.
[1] Instantiate a new random number generator.
[4] Define the method void shuffle().
[2] This method should be the same as the array version that we developed earlier.
[2] You might wish to also define a private method void swap(int i, int j). This method uses the instance field deck so that no array needs to be specified in the parameter list.
[6] Define the method String toString().
[5] This will create a new String that contains NRANKS rows of NSUITS columns with each column 17 characters wide (left justified) followed by a single space character (17 characters is the length of the longest Card string).
[1] Terminate the String with an additional new line character to allow space after the display of this String.
[4] Define the method Card deal().
Check if the deck is empty (i.e., nextCard >= NCARDS). If it is empty then throw new NoSuchElementException(String.valueOf(this.nextCard));
If not empty then return the next Card in the deck and increment the nextCard field.
[2] You may write a local main() method that you can use to unit test the correctness of this class.
class Hand [20]
The Hand class is used to hold a hand of some number of Cards.
[1] Define a symbolic constant CARDS_PER_HAND = 5.
[4] Declare 2 instance fields:
[3] Card[] hand the array to hold the cards in this hand
[1] int nCards the count of the number of cards currently in the hand
[5] Define a no-argument constructor.
[4] Instantiate the hand array with CARDS_PER_HAND elements.
[1] Set nCards to 0 to indicate an empty hand.
[5] Define the method void addCard(Card card).
[3] Set the Card in the hand with index nCards to the parameter value.
[2] Increment nCards.
[5] Define the method String toString().
Use Arrays.toString(this.hand) to build the return value.
class Poker [30]
This is the main class for the program (game).
[1] Define the symbolic constant NHANDS to have the value 4.
[4] Instantiate a new Deck with the name deck.
[5] Construct and instantiate an array of Hand elements, named hand, having NHANDS elements.
For each element of hand instantiate a new Hand object instance. Each of these hands will initially be empty.
[3] Shuffle the deck.
[2] Print out the shuffled deck.
[10] Create two nested loops.
[3] The outer loop will iterate Hand.CARDS_PER_HAND times using the loop control variable kard.
[3] The inner loop will iterate NHANDS times using the loop control variable hand.
[4] Using deck.deal() to deal the next card from the deck and add that card to the hand specified by the inner loop control variable.
This will deal 5 cards to each of the 4 hands that we set up in step 3, above.
[5] For each hand just dealt display the contents of the hand: System.out.printf(Hand %d: %s%n, hand + 1, hands[hand]);
Test out your program and upload all 4 of the class source code files (*.java) to the Assignment/Exam3 folder.
You may find it helpful to write small main() methods for the Card and Deck classes to unit test your class definitions. When running the Poker class, only its main() method is invoked and the other classes main() methods are ignored.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
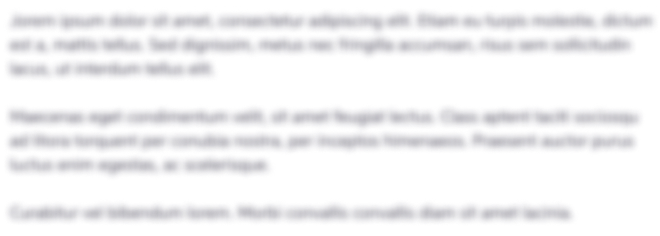
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started