Question
Introduction Welcome to Rad.io, you've been hired to work on our music streaming app, think of it as Spotify only more rad! You're in charge
Introduction
Welcome to Rad.io, you've been hired to work on our music streaming app, think of it as Spotify only more rad! You're in charge of handling our customers song list. When a user selects a playlist it will load into the list a number of songs. Users can skip to the next song, move to the previous, they can select a song to play next or select a song to add to the end of their list.
Objective
You are given two header files, Song.hpp and RadList.hpp. Song holds a songs name, artist, album, duration (in seconds) and whether or not it contains explicit lyrics. RadList keeps track of all Song objects in the linked list List. RadList also has an iterator that points to the Song object currently playing.
Complete the implementation of these classes, and make sure all tests pass. Your instructor may require you to keep your header and implementation code seperate in both .hpp and .cpp files. Your code is tested in the provided main.cpp.
You will need to implement the following functions:
- Song Constructor - This should initialize all Song member variables.
- Song Getters - name, artist, album, minutes, seconds and explicit lyrics should all be returned with these member functions. Keep in mind the duration in seconds is stored. The minutes() and seconds() getters should return the minutes and leftover seconds of a song. For example a song with a duration of 291 seconds should return 4 minutes and 51 seconds.
- next() - This should move the nowPlaying iterator to the next song in the list.
- prev() - This should move the nowPlaying iterator to the previous song in the list.
- nowPlaying() - This should return the current Song the nowPlaying iterator is currently pointing to.
- addToList() - This should add the passed in song to the end of the list.
- playNext() - This should add the passed in song as the next song in the list right after the current song playing.
I need help! I can't seem to figure out the last piece of code. I think I completed the Song.cpp correctly but I can't seem to figure out the code for RadList.hpp. Please help! Thanks in advance!
//DownTempo.csv
All In Forms, Bonobo, Black Sands, 291, false Recurring, Bonobo, Days to Come, 307, false Awake, Tycho, Awake, 283, false Dye the Water Green, Bibio, Silver Wilkinson, 325, false
//Song.hpp
#pragma once
#include
class Song {
private:
std::string name_;
std::string artist_;
std::string album_;
unsigned int duration_;
bool explicit_lyrics_;
public:
Song(std::string, std::string, std::string, unsigned int, bool);
std::string name();
std::string artist();
std::string album();
unsigned int minutes();
unsigned int seconds();
bool explicit_lyrics();
};
Song::Song(std::string name, std::string artist, std::string album, unsigned int duration, bool lyrics)
{
this->name_ = name;
this->artist_ = artist;
this->album_ = album;
this->duration_ = duration;
this->explicit_lyrics_ = lyrics;
};
std::string Song::name()
{
return name_;
};
std::string Song::artist()
{
return artist_;
};
std::string Song::album()
{
return album_;
};
unsigned int Song::minutes()
{
return duration_ / 60;
};
unsigned int Song::seconds()
{
return duration_ % 60;
};
bool Song::explicit_lyrics()
{
return explicit_lyrics_;
};
//RadList.hpp
#pragma once
#include
#include
#include
#include
#include
#include "Song.hpp"
class RadList {
private:
std::list queue_;
std::list::iterator nowPlaying_;
public:
void loadPlaylist(const std::string&);
void next();
void prev();
Song nowPlaying();
void addToQueue(const Song&);
void playNext(const Song&);
};
void RadList::loadPlaylist(const std::string& filename) {
std::ifstream playlist(filename);
if (playlist.is_open()) {
std::string name, artist, album, duration, explicit_lyrics, toss;
// Read in everything from comma seperated values file
while (std::getline(playlist, name, ',')) {
std::getline(playlist, toss, ' '); // ignore leading space
std::getline(playlist, artist, ',');
std::getline(playlist, toss, ' '); // ignore leading space
std::getline(playlist, album, ',');
std::getline(playlist, toss, ' '); // ignore leading space
std::getline(playlist, duration, ',');
std::getline(playlist, toss, ' '); // ignore leading space
std::getline(playlist, explicit_lyrics);
// Construct Song and add to queue
queue_.push_back(Song(name, artist, album, stoi(duration), explicit_lyrics == "true"));
}
playlist.close();
nowPlaying_ = queue_.begin();
} else {
throw std::invalid_argument("Could not open " + filename);
}
}
void RadList::next()
{
}
void RadList::prev()
{
}
Song RadList::nowPlaying()
{
}
void RadList::addToQueue(const Song& s)
{
}
void RadList::playNext(const Song& s)
{
}
//main.cpp
#include
#include
#include "Song.hpp"
#include "RadList.hpp"
using std::string;
using std::cout;
using std::endl;
// Helper Functions
template
void assertEquals(string, T, T);
int main(int argc, char const *argv[]) {
RadList jukebox;
jukebox.loadPlaylist("Downtempo.csv");
assertEquals("RadList.name()", static_cast("All In Forms"), jukebox.nowPlaying().name());
jukebox.next();
assertEquals("RadList.artist()", static_cast("Bonobo"), jukebox.nowPlaying().artist());
jukebox.prev();
assertEquals("RadList.minutes()", static_cast(4), jukebox.nowPlaying().minutes());
assertEquals("RadList.seconds()", static_cast(51), jukebox.nowPlaying().seconds());
jukebox.next();
jukebox.addToQueue(Song("Black Canyon", "Ana Caravelle", "Basic Climb", 457, false));
jukebox.next();
jukebox.playNext(Song("Tumbleweed", "9 Lazy 9", "Sweet Jones", 192, false));
assertEquals("RadList.name()/album()", jukebox.nowPlaying().name(), jukebox.nowPlaying().album());
jukebox.addToQueue(Song("Building Steam With A Grain Of Salt", "DJ Shadow", "Endtroducing...", 399, true));
jukebox.next();
assertEquals("RadList.playNext())", static_cast("9 Lazy 9"), jukebox.nowPlaying().artist());
jukebox.next();
assertEquals("RadList.album()", static_cast("Silver Wilkinson"), jukebox.nowPlaying().album());
jukebox.next();
assertEquals("RadList.addToQueue()", static_cast("Ana Caravelle"), jukebox.nowPlaying().artist());
jukebox.next();
assertEquals("RadList.explicit_lyrics()", true, jukebox.nowPlaying().explicit_lyrics());
return 0;
}
template
void assertEquals(string test_name, T expected, T actual) {
if (actual == expected) {
cout << "[PASSED] " << test_name << endl;
} else {
cout << "[FAILED] " << test_name
<< " - Expected: " << expected
<< ", Actual: " << actual
<< endl;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
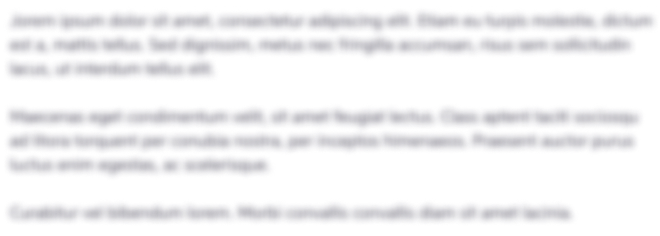
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started