Question
Is this java class diagram correct for the included program? ________________________________________________________________________________________________ import java.util.Scanner; public class Driver { //Main Method public static void main(String[] args) {
Is this java class diagram correct for the included program?
________________________________________________________________________________________________
import java.util.Scanner;
public class Driver
{
//Main Method
public static void main(String[] args)
{
//Opens the file
FileIO a1 = new FileIO("C:\\Users\\JamesJr\\OneDrive\\Documents\\School\\CURRENT QUARTER"
+ "\\Winter 2018\\Java 35A\\Assignments\\Assignment 3\\Salesdat.txt");
//reads the data, creates a franchise
Franchise franchise = a1.readData();
//Creates a scanner to take in user input
Scanner keyboard = new Scanner(System.in);
//calls the function doRun
doRun(franchise, keyboard);
}
//takes user input and and prints requested data
private static void doRun(Franchise franchise, Scanner keyboard)
{
char option;
int storeNumber;
float results [] = new float[5];
Store store = null;
do
{
//Gets Store Number from the user
storeNumber = getStoreNumber(keyboard) - 1;
store = franchise.getStore(storeNumber);
System.out.println();
//Gets menu option from the user
option = showMenu(keyboard);
System.out.println();
//Print Options
switch(option)
{
//when user selects a-g/A-G it calculates and prints selection
case 'A':
case 'a':
store.computeWeeklyTotals();
store.printWeeklyTotals();
break;
case 'B':
case 'b':
store.computeWeeklyAverages();
store.printWeeklyAverages();
break;
case 'C':
case 'c':
System.out.printf("%-25s: %.2f%n", "Total Sales for all Weeks",
store.getTotalSaleForAllWeeks());
System.out.printf("%n");
break;
case 'D':
case 'd':
System.out.printf("%-25s: %.2f%n", "Average Weekly Sales",
store.getAverageWeeklySales());
System.out.printf("%n");
break;
case 'E':
case 'e':
System.out.printf("%-25s: %d%n", "Week # with Highest Sales",
store.getWeekWithHighestSaleAmount() + 1);
System.out.printf("%n");
break;
case 'F':
case 'f':
System.out.printf("%-25s: %d%n", "Week # with Lowest Sales",
store.getWeekWeithLowestSaleAmount() + 1);
System.out.printf("%n");
break;
case 'G':
case 'g':
store.analyzeResults();
store.printResults();
break;
case 'X':
case 'x':
System.out.printf("GoodBye!.... Thanks for using our Sales App");
}
System.out.printf("");
}while(option != 'X');
}
//prints the menu
private static char showMenu(Scanner keyboard)
{
char option;
System.out.printf("STORE SALES APPLICATION MAIN MENU%n");
System.out.printf(" A - The total sales for each week.%n");
System.out.printf(" B - The average daily sales for each week.%n");
System.out.printf(" C - The total sales for all the weeks.%n");
System.out.printf(" D - The average weekly sales.%n");
System.out.printf(" E - The week with the highest amount in sales.%n");
System.out.printf(" F - The week with the lowest amount in sales.%n");
System.out.printf(" G - All of the above.%n");
System.out.printf(" X - Exit Application.%n");
System.out.printf("Enter selection: ");
option = keyboard.next().toUpperCase().charAt(0);
return option;
}
//takes in the user input of 1-6 for the store
public static int getStoreNumber(Scanner keyboard)
{
int storeNumber = 0;
do {
System.out.printf("WELCOME TO THE STORE SALES APPLICATION%n");
System.out.print("Please enter Store number (1-6): ");
storeNumber = keyboard.nextInt();
if(storeNumber 6)
{
System.out.println("Invalid Store number entered");
}
}while(storeNumber 6);
return storeNumber;
}
}
_________________________________________________________________________________________________
import java.io.*;
import java.util.*;
public class FileIO
{
private String fname = null;
private boolean DEBUG = false;
//constructor
public FileIO(String fname)
{
this.fname = fname;
}
public Franchise readData()
{
Franchise a1 = null;
//counter to count the number of lines
int counter = 0;
//attempts this block of code, if something goes wrong catch an exception
try
{
//opens a text file
FileReader file = new FileReader(fname);
//takes the text file and links file to a buffer
BufferedReader buff = new BufferedReader(file);
String temp;
boolean eof = false;
while (!eof)
{
String line = buff.readLine();
counter++;
//if line equals null it is the end of the file
if (line == null)
eof = true;
else
{
if (DEBUG)
System.out.printf("Reading" + line);
if (counter == 1)
{
//puts the line in temp
temp = line;
a1 = new Franchise(Integer.parseInt(temp));
if (DEBUG)
System.out.printf("d " + a1.numberOfStores());
}
if (counter == 2)
;
if (counter > 2)
{
int x = buildStore(a1, (counter-3), line);
if (DEBUG)
System.out.printf("Reading Store # "+(counter-2)+" "
+ "Number of weeks read = " + x);
if (DEBUG)
{
System.out.printf("Data read:");
a1.getStore(counter-3).printdata();
}
}
}
}
buff.close();
} catch (Exception e) //if code does not work catch exception
{
//prints error message
System.out.println("Error -- " + e.toString());
}
return a1;
}
public int buildStore(Franchise a1, int counter, String temp)
{
//Builds a new store
Store tstore = new Store();
String s1 = "";
float sale = 0.0f;
int week = 0;
int day = 0;
StringTokenizer st = new StringTokenizer(temp);
//loop to read 7 days of data, stops reading at 7 days
while (st.hasMoreTokens())
{
for(day=0;day
{
s1 = st.nextToken();
sale = Float.parseFloat(s1);
//sets the end of week by value
tstore.setSaleOfDay(week, day, sale);
}
week++;
}
a1.setStore(tstore);
return week;
}
}
________________________________________________________________________________________________
public class Franchise
{
//array of stores
private Store stores[];
//Maximum stores that can be accommodated.
private final int MAX_STORES;
//Current number of stores.
private int index;
//Constructor
public Franchise(int num)
{
//Max Number of Stores.
this.MAX_STORES = num;
//Allocate array memory.
this.stores = new Store[MAX_STORES];
//CURRENT number of stores.
this.index = 0;
}
/**
* Function to get given Store with in index.
*
* @param i as index
* @return store
*/
public Store getStore(int i)
{
if(isIndexValid(i))
return stores[i];
return null;
}
//checks if the given store number is valid
private boolean isIndexValid(int i)
{
//if in range, its valid
if(i >= 0 && i
{
return true;
}
return false;
}
//sets or updates the number of stores
public void setStore(Store store)
{
this.stores[index++] = store;
}
//gets the number of stores
public int numberOfStores()
{
return index;
}
}
_________________________________________________________________________________________________
public class Store
{
private final int MAX_WEEKS = 5;
private final int MAX_DAYS = 7;
// Array to accommodate Sales for 5 Week, 7 Days a Week.
private float salesByWeek[][];
private float totalSalesEachWeek [];
private float averageSalesEachWeek[];
private float totalSales;
private float weeklyAverage = 0;
private int weekWithHighestSale;
private int weekWithLowestSale;
//Constructor
public Store()
{
//Algorithms to calculate values sales of week, total sales, average sales
salesByWeek = new float[MAX_WEEKS][MAX_DAYS];
totalSalesEachWeek = new float[MAX_WEEKS];
averageSalesEachWeek = new float[MAX_WEEKS];
}
//sets the sale for a given day of week
public void setSaleOfDay(int week, int day, float sale)
{
this.salesByWeek[week][day] = sale;
}
//displays/prints data in a neat manner
public void printdata()
{
for (int i = 0; i
{
for (int j = 0; j
{
System.out.print(salesByWeek[i][j] + " ");
}
System.out.printf("");
}
}
float [] getSalesOfAWeek(int week)
{
float sales [] = new float[MAX_DAYS];
// If week is valid, store week sales into array
// and return
if(week >= 0 && week
{
for(int i=0; i
{
sales[i] = this.salesByWeek[week][i];
}
}
return sales;
}
//gets the sales of any given day of a week
float getSaleOfADay(int week, int day)
{
if(week >= 0 && week
{
if(day >= 0 && day
{
return this.salesByWeek[week][day];
}
}
//if no sale
return -1;
}
// a. total sales for week (float[]
//Calculates the sales for any given week
public float getTotalSalesForWeek(float weekSales [])
{
float sum = 0;
for(int i=0; i
{
sum += weekSales[i];
}
return sum;
}
// b. avg sales for week (float[]
//calculates average sales for the chosen week
public float getAverageSaleForWeek(float [] weekSales)
{
float sum = this.getTotalSalesForWeek(weekSales);
return sum / MAX_DAYS;
}
// c. total sales for all weeks - float - use a
//Calculates total sales for all the weeks sales
public float getTotalSaleForAllWeeks()
{
float total = 0;
for(int i=0; i
float [] weekSales = this.getSalesOfAWeek(i);
float sum = this.getTotalSalesForWeek(weekSales);
total += sum;
}
return total;
}
// d. average weekly sales - float - use b
//calculates the average weekly sales
public float getAverageWeeklySales()
{
float total = 0;
for(int i=0; i
{
float weeklySales [] = this.getSalesOfAWeek(i);
float average = this.getAverageSaleForWeek(weeklySales);
total += average;
}
return total / MAX_WEEKS;
}
// e. week with highest sale amt - float - use a
//calculates the week with the highest sales
public int getWeekWithHighestSaleAmount()
{
int maxWeek = 0;
float weekTotal = 0;
for(int week=0; week
{
float weeklySales [] = this.getSalesOfAWeek(week);
float total = this.getTotalSalesForWeek(weeklySales);
//Determine max weekly sales
if(total > weekTotal)
{
weekTotal = total;
maxWeek = week;
}
}
return maxWeek;
}
// f. week with lowest sale amt - float - use a
//calculates the week with the lowest sales
public int getWeekWeithLowestSaleAmount()
{
int minWeek = 0;
float weekTotal = Float.MAX_VALUE;
for(int week=0; week
{
float weeklySales [] = this.getSalesOfAWeek(week);
float total = this.getTotalSalesForWeek(weeklySales);
//Determine min weekly sales
if(total
{
weekTotal = total;
minWeek = week;
}
}
return minWeek;
}
//calculates the weekly totals
public void computeWeeklyTotals()
{
//total, Average sales for each week
for(int week=0; week
{
float weeklySales [] = this.getSalesOfAWeek(week);
this.totalSalesEachWeek[week] = this.getTotalSalesForWeek(weeklySales);
}
}
//calculates the weekly averages
public void computeWeeklyAverages()
{
//total, Average sales for each week
for(int week=0; week
{
float weeklySales [] = this.getSalesOfAWeek(week);
this.averageSalesEachWeek[week] = this.getAverageSaleForWeek(weeklySales);
}
}
// analyze results //call a through f or all of the above
public void analyzeResults()
{
//total, Average sales for each week
this.computeWeeklyTotals();
this.computeWeeklyAverages();
//Total and Average for all weeks
this.totalSales = this.getTotalSaleForAllWeeks();
this.weeklyAverage = this.getAverageWeeklySales();
//Highest, Lowest Sales.
this.weekWithHighestSale = this.getWeekWithHighestSaleAmount();
this.weekWithLowestSale = this.getWeekWeithLowestSaleAmount();
}
public void printWeeklyTotals()
{
int week;
System.out.printf("Weekly Total Sales:%n");
//Weekly totals
for(week = 0; week
{
System.out.printf("%10s#%d", "Week", week+1);
}
System.out.printf("%n");
for(week = 0; week
{
System.out.printf("%12.2f", this.totalSalesEachWeek[week]);
}
System.out.printf("%n%n");
}
//prints the weekly averages
public void printWeeklyAverages ()
{
int week;
System.out.printf("Weekly Average Sales:%n");
//Weekly totals
for(week = 0; week
{
System.out.printf("%10s#%d", "Week", week+1);
}
System.out.printf("%n");
for(week = 0; week
{
System.out.printf("%12.2f", this.averageSalesEachWeek[week]);
}
System.out.printf("%n%n");
}
// print() - prints - well formated - uses printf - prints only the things user wants
//displays/prinhts the results
public void printResults()
{
this.printWeeklyTotals();
this.printWeeklyAverages();
//Totals
System.out.printf("%-25s: %.2f%n", "Total Sales for all Weeks",
this.totalSales);
System.out.printf("%-25s: %.2f%n", "Average Weekly Sales",
this.weeklyAverage);
System.out.printf("%-25s: %d%n", "Week # with Highest Sales",
this.weekWithHighestSale + 1);
System.out.printf("%-25s: %d%n", "Week # with Lowest Sales",
this.weekWithLowestSale + 1);
System.out.println();
}
}
PEOPLE tMAX_WEEKS: int-5 +MAX DAYS: int-7 +salesByWeek: float[l +totalSalesEachWeek: float[] +averageSalesEachWeek: float +totalSales: float +weeklyAverage: float-0 +week WithHighestSale: int +weekWithLowestSale: int +Store +setSaleOfDay(int,int,float): void +printdata0: void +getSalesOfAWeek(int):float] +getSaleOfADay(int,int): float +getTotalSalesForWeek (float[]): float tgetAverageSaleForWeek (float]): float +getTotalSaleForAllWeeks0: float +getAverageWeeklySales0:float +get Week WithHighestSaleAmount0: int +get Week WithLowestSaleAmount: int +computeWeeklyTotals0: void +computeWeekly Averages0: void +analyzeResultsO:void +printWeeklyTotals0: void +printWeeklyAverages0: void +printResults0: void FILEIO +fname: string = null +DEBUG: Boolean false +FileIO(string) +readData0:Franchise +-buildStore(franchise, int, string): int DRIVER +Driver0 +main(String[]): void +doRun(Franchise,Scanner): void +showMenu(scanner): char +getStoreNumber(scanner): int FRANCHISE +MAX STORES: int +index: int +franchise(int) +getStore(int): store isIndex Valid(int): Boolean +setStore(Store):void Public Private Protected Package numberOfStores0: intStep by Step Solution
There are 3 Steps involved in it
Step: 1
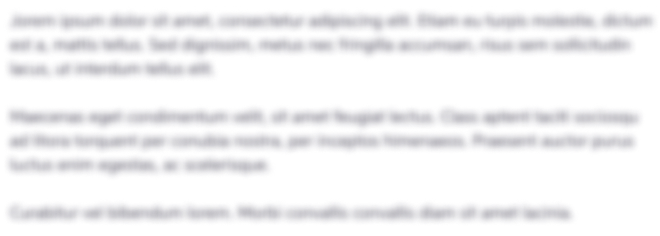
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started