Question
Its a java question I want to create the following game; A 6 by 5 maze where a mouse m rooms in it, with a
Its a java question
I want to create the following game;
A 6 by 5 maze where a mouse m rooms in it, with a number of boxes where some of the boxes contain cheese and the other are traps.
the maze grid inputFile.txt is as follows,
m . . . .
. . b . .
. . . . .
b b . . .
. . . . b
. . . . .
// 6 by 5 grid with
4 number of boxes
2number of cheese
3 0 -- cheese position
1 2cheese position, the remaining boxes are traps.
Add the following to enable moving along the Maze
count variables: one to track how many cheese crumbs Mouse has collected, and
one to track how many moves Mouse has made moving through the grid
Create 1 boolean instance variable to indicate that Mouse is still loose and roaming the Maze.
Create a method void move(char direction). This will move Mouse in the direction
specified. The following characters will represent the movements along the grid
- w up
- s down
- a - left
- d - right
Throw appropriate IndexOutOfBoundsException for moving off the grid. If the move is
valid, then process the move using the following helper method.
Create method private void processMove(char direction), which determines the next
outcome of the game based on the direction from the move() method.
- A move onto a valid path (.) increases the number of moves Mouse has made.
- A move onto a b can either:
- increase Mouses cheese collection by 1 (as well as her move count), or
- end the game (and increase her move count) by calling the endTerror()method you will implement in the next phase.
Update the grid to show Mouses movements. If she moves, replace her last position in the
grid with . and set the char at the new position to s. If Mouse collects a cheese, replace the
b with s to show where she currently is. When she moves from that location, update the
grid accordingly.
Game ending condition
Mouse has to keep scouring the Maze for boxes of cheese crumbs. She only stops when any of
these happen:
- Mouse has collected all the cheese in the Maze, or
- Mouse is caught by a trap in a box.
To do this:
Create method private void endTerror(), that changes the value of the variable indicating
that Mouse is still roaming the Maze.
Create a method boolean isRoamingMaze() that tells the outside world if Mouse is still on
the loose in the Maze!
Update the processMove method to check if the game is over, and calling endTerror to
end the game.
Extracting data
First, create your own Exception type named DataDoesNotExistException. For this class,
implement only a constructor that receives a String message.
Then, add two methods to your maze class:
char [ ] extractRow(int rowNum) that extracts the row specified by the passed
integer. 0 will fetch the first row, 1 the second (index-by-zero rules).
- char [ ] extractColumn(int colNum), which extracts a single column, much like
extract row.
Both methods should throw a DataDoesNotExistException when passed a row or column
number that does not exist in your Maze grid.
Both methods should throw a DataDoesNotExistException when passed a row or column
number that does not exist in your city grid.
For example, if your city is a 3x3 grid and the extractRow() method is passed a -1
Exception Thrown: DataDoesNotExistException: BigCity grid does not have a row index of -1
Write a toString with Mouses stats!
When Mouses reign of terror is done in a Big Maze, update the output of your toString() method to include one of the following on a new line in addition printing out the map.
For an extra, optional challenge: watch out for singular and plural tenses.
Sample output:
Mouse outsmarted the exterminators and got away.
Moves 5
cheese collected 2
traps escaped - 3
Mouses reign of terror came to an end when she met a trap instead of cheese.
Moves 10
cheese collected 1
If the game is still running, print out just the map.
Hint: You will know if Mouse was captured if she is no longer roaming the Maze and somehow there are still cheese crumbs left.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
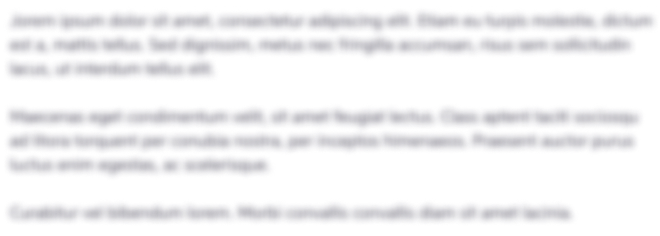
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started