Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
ITSC 1213 Lab 2 In this lab, you will develop a program to simulate a lottery. One can choose to implement everything in the main
ITSC 1213 Lab 2 In this lab, you will develop a program to simulate a lottery. One can choose to implement everything in the main method. However, this is not the best approach because it is difficult for us to read and understand a long method. Instead, you will modularize the program into methods, with each performing a major task. OVERVIEW 1) Part A: void methods 2) Part B: Value-returning methods 3) Part C: Array parameters 4) Part D: Overloaded methods 5) Part E: Java documentation for methods Part A: void methods Objective: Be able to write a method that does not return a value 1) In NetBeans, create a new project called Lab2Lottery. 2) Below the main method, but within the Lab2Lottery class, create a static method called displayRules that takes no parameters and does not return a value. (static is a java keyword, which you will learn more about in the near future. For now, just remember that if a method is static, you can call it without creating an object first.) Begin by typing the method header and an empty method body as below: public class Lab2 Lottery { para args the command line arguments public static void main(String] args) { // TODO code application Logic here public static void displayRules() { 3) This method will simply print out the rules of the lottery. The rules should appear to the user as: Welcome to the Fantasy Five Lottery. Each player picks 5 numbers between 0-35. Drawings are held Monday through Saturday at 5:00 pm. Best of luck in your game! Complete the body of this method. Complete the body of this method. 4) Test your method by calling it in the main method by typing: displayRules(); 5) Click the run button and you should see the rules in the output window. Show your method and output to the professor or a TA to get credit for this part. Part B: Value-return methods Objective: Be able to write a method that returns a certain value In Part A, you defined a method that does not take any arguments and does not return a value. It simply prints out several messages. In this part, you are going to practice writing methods that return a value. Specifically, you will define a method that will generate the winning numbers, and another method that will get a player's lottery picks. 1) Below the displayRules() method, create a static method called generate Winning Numbers that has no parameters. This method will return an array of five integers. You cannot use void as its return type. What will be the return type? 2) Here is some pseudocode to help you implement your new method: Create an integer array with 5 elements. Create an object of the Random class REPEAT 5 times: Generate a random integer in the range of O through 35. Store the random integer in the array Return the integer array 3) Next, below the generate WinningNumbers() method, create a static method called getPlayerPicks() that takes no parameters. This method will return an array of five integers. 4) This method will ask the user to enter 5 integers in the range of through 35, which will be stored in an integer array. At the end, the method will return the integer array. Note that your program must check if the user's input is in the range of O through 35 before storing it in the array. Hint: you need a loop for this. 5) To test your methods, call them in the main method by typing: 5) To test your methods, call them in the main method by typing int[] winning Numbers = generateWinningNumbers(); int[] player Picks = getPlayerPicks(); 6) Then, add statements in the main method to print out each clement of winning Numbers and playerPicks. 7) Click the run button and you should get a similar output as that given below. Note that your numbers may be different but they must be in the range of O through 35. Winning ticket ist 8 12 24 28 19 Player's pick ist & 29 1 33 25 8) Show your method and output to the professor or a TA to get credit for this part. Part C: Array parameters Objective: Be able to write a method that takes arrays as arguments. So far, you are able to display the rules, generate winning tickets, and get a player's lottery picks. Next you are going to define a method to compare the corresponding elements in the two arrays and return the number of digits that match. For example, if the winning ticket is 8 12 24 28 19', and the player's pick is *8 29 1 33 25', the method returns 1. 1) Below the getPlayerPicks() method, create a static method called matchNumbers that takes two integer arrays as parameters. Further, this method will return the number of digits that match. 2) Implement the method. 3) To test your method, call it in the main method by typing: int matching = matchNumbers (winningNumbers, playerPicks) ; System.out.println("Number of matching digits: " + matching); 4) Show your method and output to the professor or a TA to get credit for this part. Part D: Overloaded methods Objective: Be able to overload methods. In this part, you are going to define methods to calculate the Part D: Overloaded methods Objective: Be able to overload methods. In this part, you are going to define methods to calculate the prize amount for players. You will define two methods for this, one calculates the prize based on the number of matching digits, while the other calculates the prize based on two integer arrays. Since these two methods have the same purpose, they will have the same name, but they will operate on different data, i.e., different parameters. 1) Below the match Numbers method, create a static method called calculate Prize() that takes the number of matching digits as a parameter. Further, this method does not return anything. 2) The prize is calculated as follow: O or 1 position matching: $0 2 positions matching: S10 3 positions matching: 550 4 positions matching: $250 5 positions matching: Jackpot 3) Your method will print out the prize that the player wins, e.gr if the player's pick matches 2 positions, she or he will see "Congratulations! You won $10!" 4) To test your method, call it in the main method by typing: calculatePrize (matching); 5) Next, you will define the overloaded calculate Prize() method that takes two integer arrays as parameters. It does not return anything. 6) This method first finds out the number of matching digits and then display the prize amount that player won. The prize is calculated with the same logic as the other method. You must do this in only two lines of code. Hint: Call other methods that you have already defined. 7) To test your method, call it in the main method by typing calculatePrize(winningNumbers, playerPicks); 8) Show your method and output to the professor or a TA to get credit for this part. 5) Next, you will define the overloaded calculate Prize() method that takes two integer arrays as parameters. It does not return anything 6) This method first finds out the number of matching digits and then display the prize amount that player won. The prize is calculated with the same logic as the other method. You must do this in only two lines of code. Hint: Call other methods that you have already defined. 7) To test your method, call it in the main method by typing: calculatePrize(winning Numbers, playerPicks); 8) Show your method and output to the professor or a TA to get credit for this part Part E: Java documentation for methods Objective: Be able to write Javadoc comments for methods. 1) Add Javadoc comments for each of the 6 static methods that you created. They should include - A one-line summary of what the method does. - @param listing and describing each of the parameters in the parameter list (if any). - @return describing the information that is returned to the calling statement (if any). 2) When you are done, generate the Javadoc (click Run Generate Javadoc). Check the method summary and the method details to ensure your comments were put into the Java Documentation correctly. 3) Show your Javadoc of the Lab2 Lottery class to the Professor or a TA to get credit for this part. You're done! If you are using the lab computer, remember to logout. So what did you learn in this lab? 1) Practiced how to define a method and call a method 2) Practiced how to overload a method 3) Practiced writing and generating Javadoc for methods Page 7 of 4 IN JAVA PLEASE






Step by Step Solution
There are 3 Steps involved in it
Step: 1
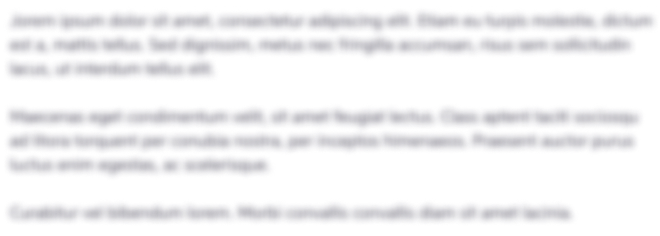
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started