Question
ja va file /* * A simple program to test a class Professor */ public class QO3 { public static void main(String[] args) { Professor
java file
/*
* A simple program to test a class Professor
*/
public class QO3
{
public static void main(String[] args) {
Professor rachel = new Professor("Rachel");
Professor joey = new Professor("Joey");
Professor ross = new Professor("Ross");
Professor monica = new Professor("Monica");
Professor chandler = new Professor("Chandler");
Professor phoebe = new Professor("Phoebe");
rachel.addCollaborator(joey);
rachel.addCollaborator(ross);
rachel.addCollaborator(monica);
rachel.addCollaborator(chandler);
rachel.addCollaborator(phoebe);
joey.addCollaborator(rachel);
joey.addCollaborator(ross);
joey.addCollaborator(monica);
joey.addCollaborator(chandler);
joey.addCollaborator(phoebe);
System.out.println(rachel.collaboratorsInCommon(joey));
System.out.println(joey.collaboratorsInCommon(rachel));
rachel.delCollaborator(monica);
System.out.println(rachel.collaboratorsInCommon(joey));
rachel.delCollaborator(chandler);
System.out.println(rachel.collaboratorsInCommon(joey));
rachel.delCollaborator(phoebe);
System.out.println(rachel.collaboratorsInCommon(joey));
rachel.delCollaborator(ross);
System.out.println(rachel.collaboratorsInCommon(joey));
System.out.println("Expected:\nRoss Monica Chandler Phoebe\nRoss Monica Chandler Phoebe\nRoss Chandler Phoebe\nRoss Phoebe\nRoss");
}
}
Document.java
/*
* Simple Document class
*/
public class Document
{
private String id;
private String header;
private String body;
private String footer;
public Document(String id)
{
this.id = id;
this.footer = "";
this.header = "";
this.body = "";
}
public void print()
{
System.out.println(header + "\n\n" + body + "\n\n" + footer);
}
public String getId()
{
return id;
}
public void setId(String id)
{
this.id = id;
}
public String getHeader()
{
return header;
}
public void setHeader(String header)
{
this.header = header;
}
public String getBody()
{
return body;
}
public void setBody(String body)
{
this.body = body;
}
public String getFooter()
{
return footer;
}
public void setFooter(String footer)
{
this.footer = footer;
}
}
Expense Document.java
import java.util.ArrayList;
//-----------Start below here. To do: approximate lines of code = 1
// Make the class ExpenseDocument a subclass of Document
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String employeeName, employeeId;
private ArrayList
public ExpenseDocument(String id, String name, String employeeId)
{
super(id);
this.employeeName = name;
this.employeeId = employeeId;
items = new ArrayList
}
//Overrides the print() method in class Document
public void print()
{
String itemList = "";
//-----------Start below here. To do: approximate lines of code = 5
// Set the document header to "Expense Report"
// Then append each item in the list of items to the itemList variable above.
// Append each item to itemList (declared above) by adding the item number (indexed from 1) followed by a ". " followed by
// the item string itself followed by a "\n". Set the document body variable to the employee name followed by
// " Employee Id: " followed by the employeeId followed by "\n\nExpenses:\n" followed by string itemList; Then
// print the expense report by using the print() method in the superclass Document
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
//-----------Start below here. To do: approximate lines of code = 2
// Create a method void addItem(String item) that adds the expense item to the array list of items
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
//-----------Start below here. To do: approximate lines of code = 2
// Create a method void clearItems() that removes all the items from array list of items
//Hint: look at the documentation for class ArrayList for a suitable method to use
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
DonationReceipt.java
/*
* A DonationReceipt is a Document that specifies the receiver has donated an amount of money to a charitable
* organization
*/
//-----------Start below here. To do: approximate lines of code = 1
// make class DonationReceipt a subclass of Document
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String organization;
private String receiver;
private String address;
private String receiptNumber;
private String amount;
private String date;
public DonationReceipt(String id)
{
super(id);
}
public DonationReceipt(String id, String receiver, String organization, String number, String amount, String date)
{
super(id);
this.receiver = receiver;
this.organization = organization;
this.receiptNumber = number;
this.amount = amount;
this.date = date;
}
// Set the body of the receipt. Overrides the setBody() method in Document
public void setBody(String receiptBody)
{
//-----------Start below here. To do: approximate lines of code = 2
// Set the string body variable, inherited from Document, to contain
// the given receiptBody string followed by the receiver followed by "\n\nReceipt#: " followed by
// the receiptNumber followed by "\nAmount: " followed by the amount followed by "\nDate: " followed by the date string
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
public String getOrganization()
{
return organization;
}
public void setOrganization(String organization)
{
this.organization = organization;
}
public String getReceiver()
{
return receiver;
}
public void setReceiver(String receiver)
{
this.receiver = receiver;
}
public String getAddress()
{
return address;
}
public void setAddress(String address)
{
this.address = address;
}
public String getReceiptNumber()
{
return receiptNumber;
}
public void setReceiptNumber(String receiptNumber)
{
this.receiptNumber = receiptNumber;
}
public String getAmount()
{
return amount;
}
public void setAmount(String amount)
{
this.amount = amount;
}
public String getDate()
{
return date;
}
public void setDate(String date)
{
this.date = date;
}
}
If you can please double check all works smoothly and have extra screenshots of the output showing as expected. Thank you
Step by Step Solution
3.41 Rating (151 Votes )
There are 3 Steps involved in it
Step: 1
Short Summary Implemented the program as per requirementAttached source code and sample output Source Code import javautilArrayList A Professor has a ...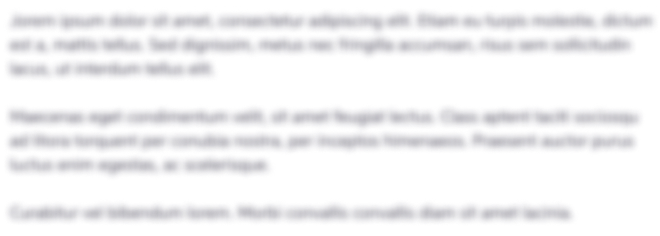
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started