Question
JAVA A Queue is an abstract data structure in which elements are added to the rear and removed from the front. This structure is often
JAVA
A Queue is an abstract data structure in which elements are added to the rear and removed from the front. This structure is often referred to as a FIRST IN FIRST OUT (FIFO) structure. There are many examples of queues in the real world: (1) waiting lines such as standing in line for movie tickets, (2) our digestive system, and (3) cars driving on a one lane one way street. Make sure understand the typical actions of a queue, such as enqueue(), dequeue(), size(), isEmpty(), before you begin. This assignment has an accompanying ZIP file that was provided in Blackboard. Download the ZIP file and unzip the contents. Do not move any of the files around within the folder created when the file is unzipped. The zipped file contains: 1. A TeamQueue.java file 2. A StudentRoster.txt file 3. A jsjf folder You will start with the TeamQueue.java file to complete your work. TeamQueue.java will need to use the CircularArrayQueue class and the QueueADT interface (provided in the jsjf folder and discussed in the chapter you read this week), and a StudentRoster.txt file. The StudentRoster.txt file contains a list of student attending an Hour of Code workshop at BCTC. For more information on this international initiative check out www.code.org The Hour of Code event will systematically divide these students into 8 teams. Each team will be assigned a specific computer lab: Team 1 will be in Room 221 Team 2 will be in Room 222 Team 3 will be in Room 233 Team 4 will be in Room 114 Team 5 will be in Room 215 Team 6 will be in Room 216 Team 7 will be in Room 117 Team 8 will be in Room 308 Part 1: Update TeamQueue.java to read student names from the StudentRoster.txt file, store the names in a queue, and sequentially assign each student to 1 of 8 teams. The first student is assigned to team 1, the second to team 2, the third to team 3, , the eighth student is assigned to team 8, the ninth student is assigned to team 1, the tenth student is assigned to team 2, etc. Use the Scanner class to read lines (names) from the StudentRoster.txt file. Place each name in the queue. (Earlier in the class you learned how to read from text files.) After each name is in the queue, read each name from the queue (in FIFO order) and create a report (formatted output file) which lists the team assignments. Include headings, a report date, and a count at the end of the report of the number of students in total participating in the Hour of Code event. The report should list each students team number and room location. Sample output for the student section of the report could be: Hosea A. Johnson Team 1 (Room 220) Lizzie J. Thomson Team 2 (Room 222) Deborah A. McMichael Team 3 (Room 209) Joseph A. Reid Team 4 (Room 207) Brittney E. Parker Team 5 (Room 312) Melissa R. Flynn Team 6 (Room 310) Molly M. Johanson Team 7 (Room 203) Frank K. Mosqueda Team 8 (Room 315) Patricia C. Cannon Team 1 (Room 220) Charlene R. Sears Team 2 (Room 222) Etc. Part 2 Copy your program and create a second version. Name the second version TeamQueues2 Now that Part 1 is working you know you can add things to a queue and remove things from a queue and assign a student to the correct team. Modify the program so that the program loads the data into the queue but does not remove them and assign them to a team yet. Then ask the user what they want to do: (1) Add another student from the keyboard (for students who register late on site) (2) Remove a student from the queue and assign them to a team (3) End the program This new feature will allow you to see the normal functioning of a queue (some go into the queue, some come out, but not necessarily all at the same time). Notice in Part 2 you are not removing all students at once. You only remove as they user indicates form the menu options (1, 2, or 3).
TeamQueue.java
/* --------------------------------
Fill in your information here
--------------------------------- */
import jsjf.CircularArrayQueue;
import java.util.Scanner;
import java.io.*;
public class TeamQueue
{
/* This program should use the Scanner class to input names from a file
Each name is placed in a queue.
Each name is then removed from the queue in order and assigned to a team number
The team number and names are then displayed.
*/
public static void main (String[] args)
{
CircularArrayQueue queue = new CircularArrayQueue();
}
}
StudentRoster.txt
Hosea A. Johnson Lizzie J. Thomson Deborah A. McMichael Joseph A. Reid Brittney E. Parker Melissa R. Flynn Molly M. Johanson Frank K. Mosqueda Patricia C. Cannon Charlene R. Sears Emily J. Washington Laverne G. Peppers Ahmad E. Lovelady Linda C. Miranda Ruth R. Caprio Dewey D. Berger Joel D. Jordan Kathleen C. Martin Marjorie S. Lyons Connie S. Harris Sandra K. Harshbarger Eugene H. Farley Frankie T. Redd Frank M. Hagerman George A. Martin Troy A. Garcia Leslie H. Smith Bernita S. Mayer Patrica J. Williamson Barry L. Jean Armando R. Simental Jill M. Marshall Michael M. Westerman Allison C. Glickman Micheal O. Hardin Mary T. Ali Kay R. Reyes Lynne L. Burris Cathy D. Olmsted Hildegard B. Twombly Joseph S. Feldman Sarah J. Moses Marilyn R. Ellis Walker D. Ouellette Tammy L. Fuller Steven S. Coleman Vincent M. Fraser John S. Bugarin Scott M. Shell Justin M. Edwards Alicia C. Smith Roosevelt A. Yandell Kimberly W. Hart Cynthia A. Campbell Thomas J. Rodriquez Catherine J. Henson Courtney S. Wiggins Anthony C. Yoder David P. Manley Ann A. Gilbert Miguel M. Garceau Trevor M. Miller Barry P. Johnson Amber T. Martinez Rosa S. Delbosque Don J. Beeson Jonathon J. Walker Jason J. Johnson Marianne P. Niemi Catherine T. Wilkerson Mitchell A. Gomez William J. Hendrick Gerda A. Peterson Wayne S. McRae Randall M. Houston Miguel J. Kearse
CircularArrayQueue.java
package jsjf;
import jsjf.exceptions.*;
/**
* CircularArrayQueue represents an array implementation of a queue in
* which the indexes for the front and rear of the queue circle back to 0
* when they reach the end of the array.
*
* @author Java Foundations
* @version 4.0
*/
public class CircularArrayQueue implements QueueADT
{
private final static int DEFAULT_CAPACITY = 100;
private int front, rear, count;
private T[] queue;
/**
* Creates an empty queue using the specified capacity.
* @param initialCapacity the initial size of the circular array queue
*/
public CircularArrayQueue (int initialCapacity)
{
front = rear = count = 0;
queue = (T[]) (new Object[initialCapacity]);
}
/**
* Creates an empty queue using the default capacity.
*/
public CircularArrayQueue()
{
this(DEFAULT_CAPACITY);
}
/**
* Adds the specified element to the rear of this queue, expanding
* the capacity of the queue array if necessary.
* @param element the element to add to the rear of the queue
*/
public void enqueue(T element)
{
if (size() == queue.length)
expandCapacity();
queue[rear] = element;
rear = (rear+1) % queue.length;
count++;
}
/**
* Creates a new array to store the contents of this queue with
* twice the capacity of the old one.
*/
private void expandCapacity()
{
T[] larger = (T[]) (new Object[queue.length *2]);
for (int scan = 0; scan < count; scan++)
{
larger[scan] = queue[front];
front = (front + 1) % queue.length;
}
front = 0;
rear = count;
queue = larger;
}
/**
* Removes the element at the front of this queue and returns a
* reference to it.
* @return the element removed from the front of the queue
* @throws EmptyCollectionException if the queue is empty
*/
public T dequeue() throws EmptyCollectionException
{
if (isEmpty())
throw new EmptyCollectionException("queue");
T result = queue[front];
queue[front] = null;
front = (front+1) % queue.length;
count--;
return result;
}
/**
* Returns a reference to the element at the front of this queue.
* The element is not removed from the queue.
* @return the first element in the queue
* @throws EmptyCollectionException if the queue is empty
*/
public T first() throws EmptyCollectionException
{
if (isEmpty())
throw new EmptyCollectionException("queue");
return queue[front];
}
/**
* Returns true if this queue is empty and false otherwise.
* @return true if this queue is empty
*/
public boolean isEmpty()
{
return (count == 0);
}
/**
* Returns the number of elements currently in this queue.
* @return the size of the queue
*/
public int size()
{
return count;
}
/**
* Returns a string representation of this queue.
* @return the string representation of the queue
*/
public String toString()
{
String result = "";
int index = front;
for (int scan = 0; scan < count; scan++)
{
result += queue[index];
index = (index + 1) % queue.length;
}
return result;
}
}
QueueADT.java
package jsjf;
import jsjf.exceptions.*;
/**
* CircularArrayQueue represents an array implementation of a queue in
* which the indexes for the front and rear of the queue circle back to 0
* when they reach the end of the array.
*
* @author Java Foundations
* @version 4.0
*/
public class CircularArrayQueue implements QueueADT
{
private final static int DEFAULT_CAPACITY = 100;
private int front, rear, count;
private T[] queue;
/**
* Creates an empty queue using the specified capacity.
* @param initialCapacity the initial size of the circular array queue
*/
public CircularArrayQueue (int initialCapacity)
{
front = rear = count = 0;
queue = (T[]) (new Object[initialCapacity]);
}
/**
* Creates an empty queue using the default capacity.
*/
public CircularArrayQueue()
{
this(DEFAULT_CAPACITY);
}
/**
* Adds the specified element to the rear of this queue, expanding
* the capacity of the queue array if necessary.
* @param element the element to add to the rear of the queue
*/
public void enqueue(T element)
{
if (size() == queue.length)
expandCapacity();
queue[rear] = element;
rear = (rear+1) % queue.length;
count++;
}
/**
* Creates a new array to store the contents of this queue with
* twice the capacity of the old one.
*/
private void expandCapacity()
{
T[] larger = (T[]) (new Object[queue.length *2]);
for (int scan = 0; scan < count; scan++)
{
larger[scan] = queue[front];
front = (front + 1) % queue.length;
}
front = 0;
rear = count;
queue = larger;
}
/**
* Removes the element at the front of this queue and returns a
* reference to it.
* @return the element removed from the front of the queue
* @throws EmptyCollectionException if the queue is empty
*/
public T dequeue() throws EmptyCollectionException
{
if (isEmpty())
throw new EmptyCollectionException("queue");
T result = queue[front];
queue[front] = null;
front = (front+1) % queue.length;
count--;
return result;
}
/**
* Returns a reference to the element at the front of this queue.
* The element is not removed from the queue.
* @return the first element in the queue
* @throws EmptyCollectionException if the queue is empty
*/
public T first() throws EmptyCollectionException
{
if (isEmpty())
throw new EmptyCollectionException("queue");
return queue[front];
}
/**
* Returns true if this queue is empty and false otherwise.
* @return true if this queue is empty
*/
public boolean isEmpty()
{
return (count == 0);
}
/**
* Returns the number of elements currently in this queue.
* @return the size of the queue
*/
public int size()
{
return count;
}
/**
* Returns a string representation of this queue.
* @return the string representation of the queue
*/
public String toString()
{
String result = "";
int index = front;
for (int scan = 0; scan < count; scan++)
{
result += queue[index];
index = (index + 1) % queue.length;
}
return result;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
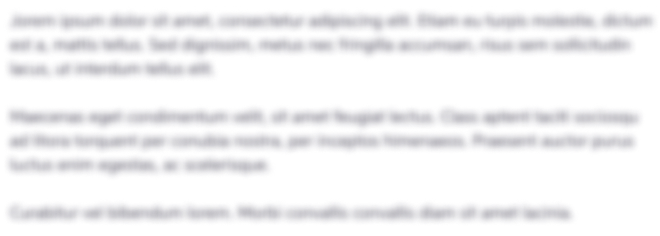
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started