Question
Java ADT Implementation: Fully develop/finish the methods for the Linked Implementation of the ADT Bag (i.e. LinkedBag) presented in chapter 3. Use the provided source
Java
ADT Implementation: Fully develop/finish the methods for the Linked Implementation of the ADT Bag (i.e. LinkedBag) presented in chapter 3. Use the provided source code as your starting point incorporate the code from the book (for all methods except the array constructor, cloning constructor, difference(), intersection(), union()). Note that I removed the inner class Node to its own separate class. You must use its methods in your LinkedBag implementation. Style requirement: you must qualify all instance variable access with this. (e.g. this.numberOfEntries). When a method manipulates multiple instances of a Bag, you should also qualify method invocations with this. (e.g. this.add() if theres also a Bag otherBag). Modify add() to prevent the addition of null. If the argument is null, throw an IllegalArgumentException with the message "entry cannot be null". Note that elsewhere in LinkedBag you will need to reverse the order of equals() invocations so the item in the Bag is the instance context and pass anEntry as the argument this will eliminate unexpected NullPointerExceptions. difference(): bag1.difference( bag2 ) returns a new LinkedBag containing all entries from bag1 (this), minus the entries contained in bag2. Remember that bags can contain duplicates if bag1 contains A, B, B, C and bag2 contains A, B, D, the resulting bag contains B, C. Do not modify the contents of bag1 or bag2! intersection(): bag1.intersection( bag2 ) returns a new LinkedBag containing all entries that appear in both bag1 (this) and bag2. Again, remember that bags can contain duplicates if bag1 contains A, B, B, C and bag2 contains A, B, D, the resulting bag contains A, B. The contents of bag1 and bag2 are not changed. union(): bag1.union( bag2 ) returns a new LinkedBag containing all entries in bag1 (this) and all entries in bag2. If bag1 contains A, B, B, C and bag2 contains A, B, D, the resulting bag contains A, A, B, B, B, C, D. Neither bag1 nor bag2 are modified. Remember that Bags are unordered so the contents may differ in order from that described (above) but the resulting bags will hold duplicates, etc. as described.
For the toString() method, the required format is [] for an empty Bag, [abc] if theres only one item in the Bag, and [abc, , ghi] for multiple items (separated by , ). Test your LinkedBag class well complete the provided LinkedBagStudentTests class: call all (BagInterface) methods with valid and invalid inputs/conditions to make sure your class behaves predictably/correctly. Use main() and the provided testXxx() stubs: each testXxx() must run completely independently of the others: o you are not permitted to use any class (static) variables o you may not change the method headers for any of the testXxx() methods o they are not permitted to take any parameters and probably dont return any results to main() do not do any console input (you may read from a file in the data folder you must use a relative path so it will run on my computer) display information about what youre testing and whether the tests succeeded or failed
public final class LinkedBag
/* * instance variables */ private Node
/* * constructors */
/** * Initialize a new, empty LinkedBag */ public LinkedBag() { // TODO Auto-generated constructor stub
} // end no-arg constructor
/** * Initialize a new LinkedBag and populate it with the contents of * {@code sourceBag} * * @param sourceBag * another bag containing zero or more entries to copy to the newly * instantiated LinkedBag * Note: {@code sourceBag} is an instance of any class that implements * {@code BagInterface} - it doesn't have to be another * {@code LinkedBag} */ public LinkedBag( final BagInterface
} // end 1-arg 'cloning' constructor
/** * Initialize a new LinkedBag and populate it with the contents of * {@code initialContents} * * @param initialContents * an array of zero or more entries to copy to the newly instantiated * LinkedBag */ public LinkedBag( final T[] initialContents ) { // TODO Auto-generated constructor stub
} // end 1-arg 'array' constructor
/* * public LinkedBag API methods */
@Override public boolean add( final T newEntry ) { // TODO Auto-generated constructor stub return false ;
} // end add()
@Override public void clear() { // TODO Auto-generated constructor stub
} // end clear()
@Override public boolean contains( final T anEntry ) { // TODO Auto-generated constructor stub
return false ;
} // end contains()
@Override public BagInterface
return null ;
} // end difference()
@Override public int getCurrentSize() { // TODO Auto-generated constructor stub return 0 ;
} // end getCurrentSize()
@Override public int getFrequencyOf( final T anEntry ) { // TODO Auto-generated constructor stub
return 0 ;
} // end getFrequencyOf()
@Override public BagInterface
return null ;
} // end intersection()
@Override public boolean isEmpty() { // TODO Auto-generated constructor stub return false ;
} // end isEmpty()
@Override public T remove() { // TODO Auto-generated constructor stub
return null ;
} // end no-arg remove()
@Override public boolean remove( final T anEntry ) { // TODO Auto-generated constructor stub
return false ;
} // end 1-arg remove()
@Override public T[] toArray() { // TODO Auto-generated constructor stub
return null ;
} // end toArray()
@Override public String toString() { /* * we'll build a string of comma-separated text representations of each * entry and surround them with square brackets */ final StringBuilder resultingString = new StringBuilder() ;
// TODO Auto-generated constructor stub
return resultingString.toString() ;
// going forward, we'll let the Arrays.toString( this.toArray() ) do the // work for us
} // end toString()
@Override public BagInterface
return null ;
} // end union()
// end of LinkedBag API methods
/* * private utility methods */
/** * Find a node in the chain with data that matches the parameter. * * @param anEntry * the entry to match * @return a reference to a node containing a matching entry or {@code null} * if not found * private Node
} // end getReferenceTo()
/** * (optional) test driver for LinkedBag * * @param args * -unused- */ public static void main( final String[] args ) {
Step by Step Solution
There are 3 Steps involved in it
Step: 1
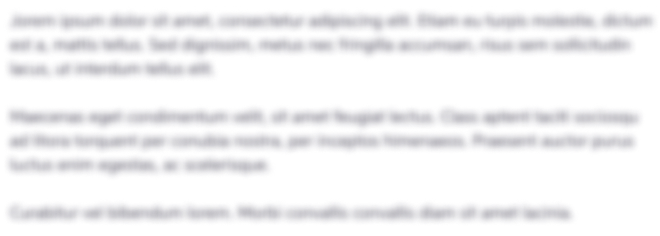
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started