Question
Java Answers Only Follow instructions exactly Create your own code For the first part of this lab you will need to write a method that
Java Answers Only
Follow instructions exactly
Create your own code
For the first part of this lab you will need to write a method that has the following header:
/** * Performs linear search to find the index of an element, or -1 if * the element is not in the list. * * @param str String to look for * @param list List to search * @postcond prints the count of the number of steps taken to the screen * @return -1 if str is not in list, or the index of the first occurrence of * str if it is in the list. */ public static int linearSearch(String str, Listlist)
Ignore the @postcond for a moment and focus on the @return. Use the notes from this week's lectures to implement linear search so that it returns back the correct index of the first value and make sure it passes the unit tests given with this project.
Note that we have given you a main method for this lab. If you need to change it to test your code that's great, but once your code is working you should restore the main method to the following:
public static void main(String[] args) { Listlist = Arrays.asList("james","ran","to","the","market"); System.out.println("Looking for to"); int idx = linearSearch("to",list); System.out.println("to found at index "+idx); System.out.println(); System.out.println("Looking for market"); idx = linearSearch("market", list); System.out.println("market found at index "+idx); System.out.println(); System.out.println("looking for monday"); idx = linearSearch("monday",list); System.out.println("monday found at index "+idx); }
Once you have completed the first part if you run that main method you should see the following as output:
Looking for to to found at index 2 Looking for market market found at index 4 looking for monday monday found at index -1
For the second part of this lab, you will need to modify your linearSearch method so that it prints out the number of comparisons being made at each step. After making these changes if you run the main method you should see the following output:
Looking for to Comparing james and to 1 comparisons Comparing ran and to 2 comparisons Comparing to and to 3 comparisons to found at index 2 Looking for market Comparing james and market 1 comparisons Comparing ran and market 2 comparisons Comparing to and market 3 comparisons Comparing the and market 4 comparisons Comparing market and market 5 comparisons market found at index 4 looking for monday Comparing james and monday 1 comparisons Comparing ran and monday 2 comparisons Comparing to and monday 3 comparisons Comparing the and monday 4 comparisons Comparing market and monday 5 comparisons monday found at index -1
Visually inspect your output to make sure it matches the above before you submit your final lab.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
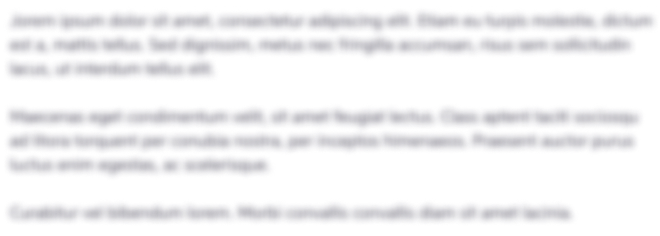
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started