Question
JAVA Assignment 9: This assignment will focus on the use of classes, objects, object/instance variables, constructor methods, class methods, object/instance methods. Follow the directions below
JAVA Assignment 9: This assignment will focus on the use of classes, objects, object/instance variables, constructor methods, class methods, object/instance methods.
Follow the directions below to submit Assignment 9:
1. You will modify the AnyString class (INCLUDED BELOW). If your solution was not working use the solution file(s) provided.The AnyString class should be modified to add the following methods:
a. Add a constructor method that will accept a character array argument. The character array argument should be used to set the object/instance variable.
b. An object/instance method toString() that accepts no arguments and returns the object/instance variable.
c. An object/instance method equals() that accepts an AnyString object and returns true if the argument contains a object/instance variable value that is equal to the object/instance variable value of the object calling the method or false if not equal.
d. An object/instance method isLetters() that accepts no arguments and returns true is the object/instance variable is all letters or false if not all letters.
e. An object/instance method isNumeric() that accepts no arguments and returns true is the object/instance variable is all numbers or false if not all numbers.
2. Create a main() method for the AnyString class. **DO NOT USE the TestAnyStringClass to test your code**. The main() method should contain the following:
a. You should input a string.
b. Create an object of the AnyString class.
c. Call all the methods in the AnyString class, verify that they work, and display the results.
d. Create a character array and create an object of the AnyString class.
e. Call all the methods in the AnyString class, verify that they work, and display the results.
---------------------------------------------------------------------------------------------------------------------------------
****Included below is the AnyString Class code to be modified****
import java.util.*;
class AnyString
{
String str;
AnyString(String s1)
{
str=s1;
}
String getString()
{
//returns the entered string value
return str; }
String lowercase()
{
//returns entered string in lowercase
return str.toLowerCase(); }
String uppercase()
{
//returns entered string in uppercase
return str.toUpperCase();
}
int getLength()
{
//returns length of entered string
return str.length();
}
}
class TestAnyStringClass
{
public static void main(String args[])
{
String strD,st;
String lw,upr;
int length;
Scanner sc=new Scanner(System.in);
System.out.println("Enter a string ");
//accepting string value from user
strD=sc.next();
//initializing class AnyString and passing accepted value to constructor
AnyString a1=new AnyString(strD);
//calling getString() to get entered value
st=a1.getString();
System.out.println("Entered String is "+st);
//calling lowercase() to get entered value in lowercase
lw=a1.lowercase();
System.out.println("Conversion of String into Lowercase : "+lw);
//calling uppercase() to get entered value in uppercase
upr=a1.uppercase();
System.out.println("Conversion of String into Uppercase : "+upr);
//calling getLength() to get the length of entered value
length=a1.getLength();
System.out.println("Length of Entered string is "+length);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
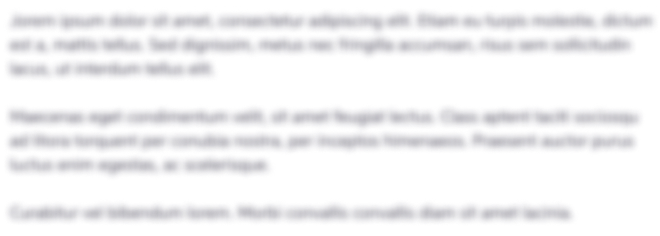
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started