Question
Java Assignment, you will write a subclass of the Memory Calc class. This new calculator called ScientificMemCalc, should be able to do every thing that
Java Assignment, you will write a subclass of the Memory Calc class. This new calculator called ScientificMemCalc, should be able to do every thing that the MemoryCalc could do plus raise the current value to a power and compute the natural logarith of the current value. You will need to override the display Menu method to add the new options. Be sure to only add code to the ScientificMemCalc class if it is necessary. Leverage the code from the base MemoryCalc class whenever possible. Use the power of inheritance to do this rather than cutting or pasting or otherwise duplicating the code. Finally, write a new class called ScientificCalcDriver that shows the functionality of your new class.
Here is my previous code.
Memory Class Code
import java.util.Scanner;
public class Memory {
public static void main(String[] args) // Main function
{
MemoryCalculator c = new MemoryCalculator(0);
Scanner choose = new Scanner(System.in);
int choice = 0;
String prompt;
boolean right = false;
double operand2;
do // Loop until user wants to quit
{
if(c.getCurrentValue() > 0)
System.out.println("The current value is " + c.getCurrentValue()); //Displaying current value
choice = c.displayMenu(choose); //Displaying the user's menu
if ((choice <= 6) && (choice > 0)) //Checking for a valid option to be selected
{
switch(choice) //Calling the appropriate function
{
case 1: operand2 = c.getOperand("What is the second number? ");
c.add(operand2);
break;
case 2: operand2 = c.getOperand("What is the second number? ");
c.subtract(operand2);
break;
case 3: operand2 = c.getOperand("What is the second number? ");
c.multiply(operand2);
break;
case 4: operand2 = c.getOperand("What is the second number? ");
c.divide(operand2);
break;
case 5: case 6: c.currentValue = 0;
System.out.println("Goodbye!");
break;
}
}
else
{
right = true;
System.out.println("I'm sorry, " + choice + " wasn't one of the options. Please try again.");
}
}while (choice != 6);
}
MemoryCalculator Code
import java.util.Scanner;
public class MemoryCalculator {
public static double currentValue=0; //Variable to hold result
public MemoryCalculator(double currentValue)
{
this.currentValue = currentValue;
}
public static int displayMenu(Scanner choose) // Displaying the user's menu
{
int choice;
System.out.println(" ");
System.out.println("Menu ");
System.out.println("1. Add");
System.out.println("2. Subtract");
System.out.println("3. Multiply");
System.out.println("4. Divide");
System.out.println("5. Clear");
System.out.println("6. Quit");
System.out.println(" ");
System.out.print("What would you like to do? ");
choice = choose.nextInt();
return choice;
}
public static double getOperand(String prompt)
{
Scanner choose = new Scanner(System.in);
System.out.print(prompt);
double option = choose.nextDouble();
return option;
}
public double getCurrentValue()
{
return this.currentValue;
}
public void add(double operand2)
{
this.currentValue += operand2;
}
public void subtract(double operand2)
{
this.currentValue -= operand2;
}
public void multiply(double operand2)
{
this.currentValue *= operand2;
}
public void divide(double operand2)
{
if (operand2 == 0)
{
System.out.println("The current value is NaN. ");
clear();
}
else
{
this.currentValue /= operand2;
}
}
public void clear()
{
this.currentValue = 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
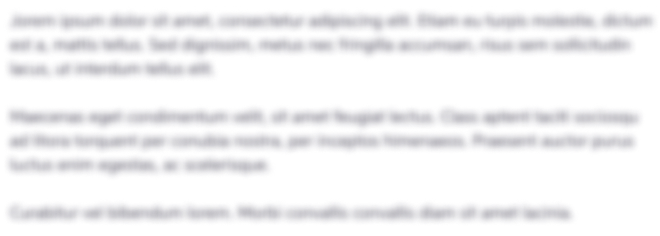
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started