Question
Java: Briefly explain the code in each area with /*comment*/ above it import java.io.*; import java.net.*; /** * This program is a very simple Web
Java: Briefly explain the code in each area with /*comment*/ above it
import java.io.*;
import java.net.*;
/**
* This program is a very simple Web server. When it receives a HTTP request,
* it just sends the request back to the client. This default port this
* server listens to is 1500.
*
* Usage:
*
* java HttpRequestMirror
*
**/
public class HttpRequestMirror {
public static void main(String args[]) throws IOException {
// Get the port to listen on
int port = 1500;
if (args.length == 1)
port = Integer.parseInt(args[0]);
try {
/* Comment Here */
ServerSocket ss = new ServerSocket(port);
System.out.println("Server bound at port " + ss.getLocalPort());
while (true) {
Socket client = ss.accept();
/* Comment Here */
BufferedReader in =
new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintWriter out =
new PrintWriter(new OutputStreamWriter(client.getOutputStream()));
/* Comment Here */
out.println("HTTP/1.1 200 ");
out.println("Content-Type: text/plain");
out.println(); // send the empty line
out.flush();
/* Comment Here */
String line;
while((line = in.readLine()) != null) {
if (line.length() == 0) break;
out.println(line);
}
/* Comment Here */
out.close();
in.close();
client.close();
}
}
catch (Exception e) {
System.err.println(e);
System.err.println("Usage: java HttpRequestMirror ");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
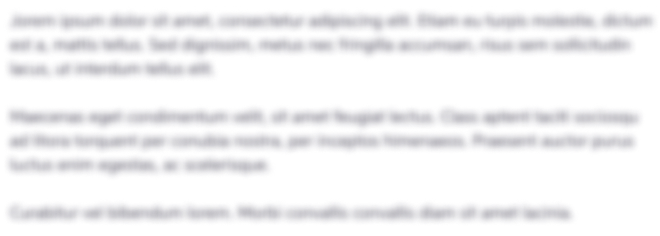
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started