Question
// Java class twoDimPrac{ int grade; public twoDimPrac(int i, int j)//notice the constructor takes in the row and column index values { this.grade=i*j; } public
// Java
class twoDimPrac{
int grade;
public twoDimPrac(int i, int j)//notice the constructor takes in the row and column index values
{
this.grade=i*j;
}
public String toString(){
String ret="";
ret=ret+this.grade;
return ret;
}
public static void main(String [] args){
//use a random number generator to generate the number of rows and columns
//the random number generator will generate values between 1 and 20
int range=(20-1)+1;
int min=1;
int rows=(int)(Math.random()*(range)+min);
//declare the 2 dim array
twoDimPrac [][] myArray;
//Task: intantiate the 2 dimensional array
//Task: using a nested for-loop print out all the objects in the array
for (int i = 0; i < myArray.length; i++) {
for (int j = 0; j < myArray[i].length; j++) {
}
}
}
}
---------------------------------------------------------------------------------------
class threeDimPrac{
int grade;
public threeDimPrac(int i, int j, int k)//notice the constructor takes in the dim1, dim 2 and dim 3 index values
{
this.grade=i*j*k;
}
public String toString(){
String ret="";
ret=ret+this.grade;
return ret;
}
public static void main(String [] args){
//use a random number generator to generate the values of dim1, dim2 and dim3
//the random number generator will generate values between 1 and 20
int range=(20-1)+1;
int min=1;
int dim1=(int)(Math.random()*(range)+min);
//declare the 3 dim array
threeDimPrac [][][] myArray;
//Task: intantiate the 3 dim array
//Task: using a nested for-loop print out all the objects in the array
}
}
---------------------------------------------------------------------------------------
public class Persons
{
String firstName;
String lastName;
int age;
static int id;
int currentid;
public Persons(){
this.firstName="John";
this.lastName="Doe";
this.age = 21;
Persons.id++;
this.currentid=id;
}
public Persons(String fName, String lName, int age){
this.firstName=fName;
this.lastName=lName;
this.age=age;
Persons.id++;
this.currentid=id;
}
//accessor methods
public String getFirstName(){
return this.firstName;
}
public String getLastName(){
return this.lastName;
}
public int getAge(){
return this.age;
}
public int getId(){
return this.currentid;
}
public String toString()
{
return "Last name: " + this.getLastName() + " First name: " + this.getFirstName() + " Age: " + this.getAge();
}
}
---------------------------------------------------------------------------------------
import java.util.Scanner;
import java.io.File;
import java.io.IOException;
public class PersonsClient
{
/*Task 1 write a static function printSameAge() that will take the array of Persons objects and the age to search for as parameters*/
public static void main(String[] args)
{
int arrSize = 0;
int lines = 0;
String str ="";
Persons p1;
Persons []people;
try {
File myFile=new File("persons.txt");
Scanner scan=new Scanner(myFile);
while(scan.hasNextLine()){
str=scan.nextLine();
++arrSize;
}
scan.close();
people = new Persons[arrSize];
scan=new Scanner(myFile);
while(scan.hasNextLine()){
str=scan.nextLine();
String []tok=str.split(",");
p1 = new Persons(tok[1], tok[0], Integer.parseInt(tok[2]));
people[lines++] = p1;
}
scan.close();
/*Write the code to prompt the user to provide an age to search for*/
}
catch(IOException ioe){
System.out.println("The file can not be read");
}
}
}
---------------------------------------------------------------------------------------
public class VehicleA
{
private String vehicle_name;
private double velocity;
private double acceleration;
private double distance;
private int time;
private static int vehicleID;
private int currentID;
private double init_v;
private double init_d;
private VehicleType vt;
public VehicleA()
{
vehicle_name=" ";
velocity=0.0;
acceleration=0.0;
time=0;
distance=0.0;
currentID=0;
init_v=0.0;
init_d=0.0;
}
public VehicleA(String name, double a, int t, double initv, double initd)
{
vehicleID++;
currentID=vehicleID;
vehicle_name=name;
acceleration=a;
time=t;
init_v=initv;
init_d=initd;
}
public String getVehicleName()
{
return vehicle_name;
}
public double getVelocity()
{
return velocity;
}
public double getAcceleration()
{
return acceleration;
}
public double getDistance()
{
return distance;
}
public int getTime()
{
return time;
}
public int getVehicleID()
{
return vehicleID;
}
public int getCurrentID()
{
return currentID;
}
public double getInitV()
{
return init_v;
}
public double getInitD()
{
return init_d;
}
public void setInitV(double inv)
{
init_v=inv;
}
public void setInitD(double ind)
{
init_d=ind;
}
public void setTime(int t)
{
time=t;
}
public void setAcceleration(double acc)
{
acceleration=acc;
}
public void setDistance(double dist)
{
distance=dist;
}
public String toString()
{
String str="The vehicle ID is:"+" "+currentID+" "+"The name of the vehicle is:"+" "+vehicle_name+" "+
"The initial velocity is"+" "+init_v+" "+"The initial distance is"+" "+init_d+" "+"The final velocity is:"+" "+velocity+"m/sec"+" "+"The final distance is:"+" "+distance+"m";
return str;
}
public void calculateV()
{
this.velocity=acceleration*time+init_v;
}
public void calculateD()
{
double timed=(double)time;
double t=Math.pow(timed, 2.0);
this.distance=0.5*acceleration*t+init_v*time+init_d;
}
public String licensePlate()
{
String substr=vehicle_name.substring(3);
String strid=String.valueOf(currentID);
String substr1=substr+strid;
return substr1;
}
public void setVehicleType(VehicleType vehty)
{
this.vt=vehty;
}
public VehicleType getVehicleType()
{
return vt;
}
public boolean equals(Object o)
{
// if o is not an Auto object, return false
if ( ! ( o instanceof VehicleA ) )
return false;
else
{
// type cast o to an Auto object
VehicleA objVeh = ( VehicleA) o;
if (this.init_v==objVeh.init_v&&this.init_d==objVeh.init_d&&this.vt.equals(objVeh.vt)&&this.time==objVeh.time)
return true;
else
return false;
}
}
public void setCalcutedTime(double dist, double acc,double iv)
{
int t=0;
double d=dist;
double a=acc;
double inve=iv;
t=(int)(((Math.pow(inve,2)+Math.sqrt(Math.pow(inve,2)+2*a*d-2*a*init_d))/a));
time=t;
}
}
---------------------------------------------------------------------------------------
public enum VehicleType {FOUR_DOOR_SEDAN,TWO_DOOR_SEDAN, TRUCK, SUV, SPORT, VAN, MINI_VAN };
---------------------------------------------------------------------------------------
import java.util.*;
public class UseVectors
{
public static void main(String[] args)
{
Vector v=new Vector();
VehicleA car1=new VehicleA();
VehicleA car2=new VehicleA();
v.addElement(car1);
v.addElement(car2);
System.out.println("The first object out of the vector:"+" "+v.get(0).toString());
Object o1=v.elementAt(0);
VehicleA veh1=(VehicleA)o1;
veh1.setAcceleration(2);
veh1.setInitD(5.0);
veh1.setInitV(2.0);
veh1.setTime(1);
veh1.calculateV();
veh1.calculateD();
System.out.println("The first object at index 0 out of the vector after we set its initial distance and initial velocity"+" "+v.get(0).toString());
VehicleA v1=(VehicleA)v.get(1);
v1.setAcceleration(3.0);
v1.setTime(2);
v1.calculateV();
v1.calculateD();
System.out.println("The second object at index 1 out of the vector after we set its initial distance and initial velocity"+" "+v.get(1).toString());
//Now lat us exchage positions of teh two objects within teh vector
v.set(0,v1);
v.set(1,veh1);
// Add a new object in the vector index 2
v.addElement(new VehicleA());
//iterate throughteh vector
for (int j=0; j
{
System.out.println("Object at index"+" "+j+"is"+" "+v.get(j).toString());
}
//task1: Add an object v2 of VehicleA whose initial velocity is 2, initial distance is 6 acceleration is 3 and time is 2.
//task2: Add an object v3 of VehicleA whose initial velocity is 3, initial distance is 7 acceleration is 4 and time is 3.
//task3: For object v3, calculate the velocity and the distance.
//task4: Enter the new objects v2 and v3 in the vector.
//task5: Iterate through the vector and display the values of the attributes of each of the 5 objects in the vector.
//task6: Remove the object at index 2 of the vector.
//task7: Display the size of the vector now (v.size())
//task8: Clear the contents of the vector.
//task9: Display the size of the vector again.
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
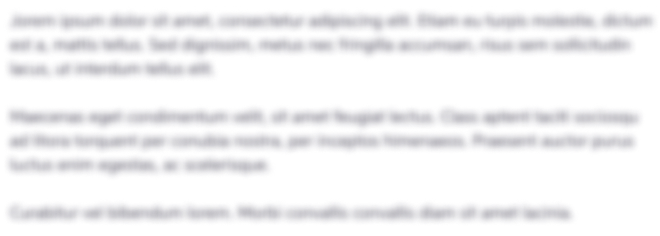
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started