Question
Java code about Enrollment System Please help. So this is the code: import java.util.Scanner; class User { String username; String password; String role; public User(String
Java code about Enrollment System
Please help.
So this is the code:
import java.util.Scanner;
class User {
String username;
String password;
String role;
public User(String username, String password, String role) {
this.username = username;
this.password = password;
this.role = role;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public String getRole() {
return role;
}
}
class Course {
String courseCode;
String courseTitle;
int units;
public Course(String courseCode, String courseTitle, int units) {
this.courseCode = courseCode;
this.courseTitle = courseTitle;
this.units = units;
}
public String getCourseCode() {
return courseCode;
}
public String getCourseTitle() {
return courseTitle;
}
public int getUnits() {
return units;
}
}
class EnrollmentSystem {
User[] users = new User[10];
int userCount = 0;
Course[] courses = new Course[10];
int courseCount = 0;
Scanner sc = new Scanner(System.in);
public void registerAccount() {
System.out.print("Enter username: ");
String username = sc.nextLine();
System.out.print("Enter password: ");
String password = sc.nextLine();
System.out.print("Enter role (student/admin): ");
String role = sc.nextLine();
users[userCount++] = new User(username, password, role);
System.out.println("Account registered successfully!");
}
public boolean login(String username, String password) {
for (int i = 0; i
if (users[i].getUsername().equals(username) && users[i].getPassword().equals(password)) {
return true;
}
}
return false;
}
public void addCourse() {
System.out.print("Enter course code: ");
String courseCode = sc.nextLine();
System.out.print("Enter course title: ");
String courseTitle = sc.nextLine();
System.out.print("Enter units: ");
int units = sc.nextInt();
courses[courseCount++] = new Course(courseCode, courseTitle, units);
System.out.println("Course added successfully!");
}
public void viewCourses() {
System.out.println("List of Courses: ");
for (int i = 0; i
System.out.println(courses[i].getCourseCode() + " - " + courses[i].getCourseTitle() + " (" + courses[i].getUnits() + " units)");
}
}
public void enroll() {
System.out.print("Enter course code: ");
String courseCode = sc.nextLine();
int courseIndex = -1;
for (int i = 0; i
if (courses[i].getCourseCode().equals(courseCode)) {
courseIndex = i;
break;
}
}
if (courseIndex == -1) {
System.out.println("Course not found.");
return;
}
System.out.println("You have enrolled in: " + courses[courseIndex].getCourseTitle());
}
}
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
EnrollmentSystem es = new EnrollmentSystem();
while (true) {
System.out.println(" Welcome to the Enrollment System");
System.out.println("[1] Register Account");
System.out.println("[2] Login");
System.out.println("[3] Exit");
System.out.print("Enter choice: ");
int choice = sc.nextInt();
if (choice == 1) {
es.registerAccount();
} else if (choice == 2) {
System.out.print("Enter username: ");
String username = sc.next();
System.out.print("Enter password: ");
String password = sc.next();
boolean isValid = es.login(username, password);
if (!isValid) {
System.out.println("Invalid username or password.");
} else {
User user = null;
for (int i = 0; i
if (es.users[i].getUsername().equals(username) && es.users[i].getPassword().equals(password)) {
user = es.users[i];
break;
}
}
if (user.getRole().equals("admin")) {
while (true) {
System.out.println(" Welcome, Admin");
System.out.println("[1] Add Course");
System.out.println("[2] View Courses");
System.out.println("[3] Logout");
System.out.print("Enter choice: ");
int adminChoice = sc.nextInt();
if (adminChoice == 1) {
es.addCourse();
} else if (adminChoice == 2) {
es.viewCourses();
} else if (adminChoice == 3) {
break;
}
}
} else if (user.getRole().equals("student")) {
while (true) {
System.out.println(" Welcome, Student");
System.out.println("[1] Enroll");
System.out.println("[2] View Courses");
System.out.println("[3] Logout");
System.out.print("Enter choice: ");
int studentChoice = sc.nextInt();
if (studentChoice == 1) {
es.enroll();
} else if (studentChoice == 2) {
es.viewCourses();
} else if (studentChoice == 3)
{
break;
}
}
}
}
} else if (choice == 3) {
System.out.println("Goodbye!");
break;
}
}
}
}
Problem 1: When I add a 2nd course as an admin, the "Enter course code:" and the "Enter course title:" began to join as one line and that goes on and on. Please, kindly edit and fix it.
Problem 2: When I'm about to enroll in a course as a student, the "Enter course code:" and the "You have enrolled in:" joined as one line and I'm not yet inputting something that I enrolled in a certain course which "csit2 programming2" appeared. Please, kindly edit and fix it.
Thank you so much in advance.
\3 Exit Enter choice: 1 Enter username: student Enter password: student Enter role (student/admin): student Account registered successfully! Welcome to the Enrollment System [1] Register Account [2] Login [3] Exit Enter choice: 2 Enter username: admin Enter password: admin Welcome, Admin [1] Add Course [2] View Courses [3] Logout Enter choice: 1 Enter course code: IT1 Enter course title: Programming 2 Enter units: 3 Course added successfully! Welcome, Admin [1] Add Course [2] View Courses [3] Logout Enter choice: 1 Enter course code: Enter course title: Welcome to the Enrollment System [1] Register Account [2] Login [3] Exit Enter choice: 2 Enter username: student Enter password: student Welcome, Student [1] Enroll [2] View Courses [3] Logout Enter choice: 2 List of Courses: csit1 - programming1 (3 units) - csit2 programming2 (3 units) - csit3 programming3 (3 units) Welcome, Student [1] Enroll [2] View Courses [3] Logout Enter choice: 1 Enter course code: You have enrolled in: csit2 programming2 Enter choiceStep by Step Solution
There are 3 Steps involved in it
Step: 1
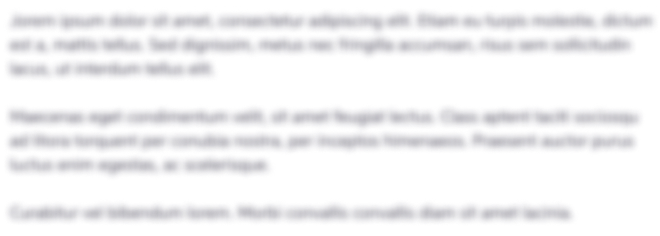
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started