Question
java code for rental car: reqs: -For the server, you will need to use a method that uses the SwingUtilities invokeLater method to update the
java code for rental car:
reqs:
-For the server, you will need to use a method that uses the SwingUtilities invokeLater method to update the scrollable text area. This is needed because Swing components are not thread safe, and the server will be multithreaded by the time you are finished with this project. The following code is an example of a method that takes a String argument and safely updates a GUI component with the string:
private void displayMessage(String message)
{
SwingUtilities.invokeLater(
new Runnable()
{
public void run()
{
outputTextArea.append(message);
}
}
);
}
-The client and server must use TCP as the basis for their communication. A TCP connection should be established between the client and server whenever a client is started. On the server side, once a connection has been established to a client, the server should call a separate method to handle the client communication. This will make adding multithreading easier! This method should take the client socket as an input parameter. This method should setup the data streams using local variables only! The client socket variable MUST NOT BE A MEMBER VARIABLE! Using member variables here would create difficulties for multithreading! The server side should only close the connection when the client has indicated that it has completed its work. Initially, the client handler will be a normal method in the server class. Later on, this will be a special method that enables the server to start a separate thread to handle each client.
-The InventoryManager must maintain a list of vehicle information which includes rental information
Phase 1: Design the message exchange between the client and the server. For each of the operations described in this document, specify the details of what values the client sends to the server and what possible values the server sends back to the client. Make sure you consider any error returns from the server. Design your Message class based on this specification. Turn in a short written description of the message exchange and your Message class source code. Your written description should be no more than a. This is due by the end of Week 3.
Phase 2: Implement the TCP and Object stream network communication between the client and server. At this point, you should be able to send message objects between the client and the server and vice versa. Have your client send a real add, get, rent, and return message. The server should display the contents of the received message, and return message indicating the requested operation failed. The client should display the returned message content appropriately. Turn in screen shots of the client and server which illustrate the message exchange. Also turn in your client and server source code. This is also due by the end of Week 3.
Phase 3: Complete implementation of the client and the single threaded server. This means implementing the Inventory Manager class and adding code to the server to make use of the Inventory Manager methods. Pseudo code for the Inventory Manager has been provided. Phase 3 will be complete when all functionality to support a single client is working. Take screen shots of successfully adding a vehicle, getting vehicle information, renting a vehicle, and returning a vehicle. These will be turned in at the end of Week 4.
Phase 4: Modify the server to make it multithreaded. This includes changing the Inventory Manager to add synchronization. Take screen shots of the server with multiple clients trying to rent the same car at the same time. Turn in all your client and server source code. This will be turned in at the end of Week 4.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
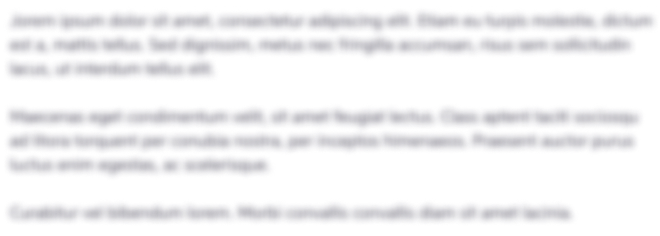
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started