Question
Java code: import java.util.Iterator; public class LinkedList { public void printReverse(){ printReverse(head); System.out.println(); } private void printReverse(Node n){ if(n != null){ printReverse(n.link); System.out.print(n.element+ );
Java code:
import java.util.Iterator;
public class LinkedList
public void printReverse(){
printReverse(head);
System.out.println();
}
private void printReverse(Node n){
if(n != null){
printReverse(n.link);
System.out.print(n.element+ " ");
}
}
public static void main(String[] args) {
String[] dwarfs = {"Happy", "Dopey", "Grumpy", "Sneezy", "Sleepy", "Bashful", "Doc"};
LinkedList
for(String d : dwarfs) {
list.add(d);
}
list.print();
}
private class HappyLittleIterator implements Iterator
public boolean hasNext() {
return false;
}
public E next() {
return null;
}
}
private class Node {
private E element; // Reference to element stored in this node
private Node link; // Reference to next node in list (or null if no next node)
public Node(E element) {
this.element = element;
}
}
private Node head; // Reference to first node in list (null if list empty)
private Node tail; // Reference to last node in list (null if list empty)
private int size; // Number of elements in this list
/**
* Default constructor - creates an empty list.
*/
public LinkedList() {
head = tail = null;
size = 0;
}
public int size() {
return size;
}
public E get(int index) {
if (index = size)
throw new IndexOutOfBoundsException(index+"");
Node walker=head;
for(int i=0; i return walker.element; } public void add(E element) { add(size, element); } public void add(int index, E element) { if (index size) throw new IndexOutOfBoundsException(index+""); Node n = new Node(element); if(size == 0) { head = tail = n; } else if (index == 0) { n.link = head; head = n; } else if (index == size) { tail.link = n; tail = n; } else { Node walker = head; for(int i=0; i n.link = walker.link; walker.link = n; } size++; } public E remove(int index) { if (index = size) throw new IndexOutOfBoundsException(index+""); E doomed = null; if (index == 0) { doomed = head.element; head = head.link; if (head == null) tail = null; } else { Node walker=head; for(int i=0; i doomed = walker.link.element; walker.link = walker.link.link; if (walker.link == null) tail = walker; } size--; return doomed; } public void print() { for(Node walker=head; walker!=null; walker=walker.link) { System.out.print(walker.element+" "); } System.out.println(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
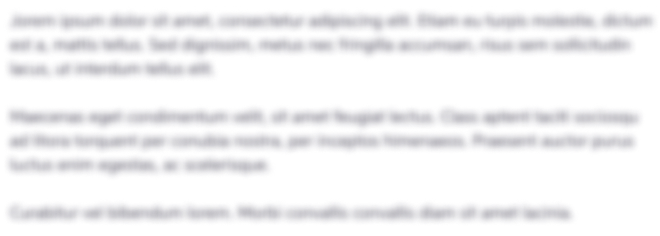
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started