Question
java code level: beginner write a method sumList() public static Integer sumList(ArrayList list) This method calculates the sum of all Integer values in a given
java code
level: beginner
write a method
sumList()
public static Integer sumList(ArrayListlist)
This method calculates the sum of all Integer values in a given ArrayList.
For example, if ArrayList
- Return null if the list is empty or null.
- Think about the base case.
- When should the method terminate/end?
- Under what condition should your method stop making recursive calls and return your expected value?
- Think about the recursive case.
- Make recursive calls to the method; make sure to call sumList in the method body.
- How would the input have to change in the recursive call? What should your parameter argument be?
- Keep in mind, you do not need to keep the original ArrayList content.
- Make recursive calls to the method; make sure to call sumList in the method body.
- Again, iterative solution will not be accepted; do NOT use loop to solve this problem.
strToList()
public static ArrayListstrToList(String word)
This method transforms a String into an ArrayList
For example, strToWord("word") will return an ArrayList
strToWord("hello world") will return an
ArrayList
- Return an empty list if the string is empty.
- Return null if the string is null
- Think about base case.
- When should the method terminate/end?
- Under what condition should your method stop making recursive calls and return your expected value?
- Think about the recursive case
- Make recursive calls to the method; make sure to call to strToList
- How would the input have to change in the recursive call?
- Keep in mind, you do not need to keep the original ArrayList content.
- An iterative solution will not be accepted.
- Make recursive calls to the method; make sure to call to strToList
findAverage()
public static double findAverage(ArrayListlist)
This method returns the average value in the given ArrayList.
For example, if ArrayList
- Return null if the list is empty or null.
- Think about base case.
- When should the method terminate/end?
- Under what condition should your method stop making recursive calls and return your expected value?
- Think about the recursive case
- Make recursive calls to the method; make sure to make a function call to findAverage
- How would the input have to change in the recursive call?
- You do not need to keep the original content of the list.
- An iterative solution will not be accepted; do NOT use a for loop.
- Hint: You should use the idea you used in sumList because finding the average requires calculating the sum.
- However, you cannot use sumList in this method directly. You are only allowed to make function calls to findAverage itself.
- You cannot call the method sumList in findAverage
- What operation do you have to do on the sum to find the average?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
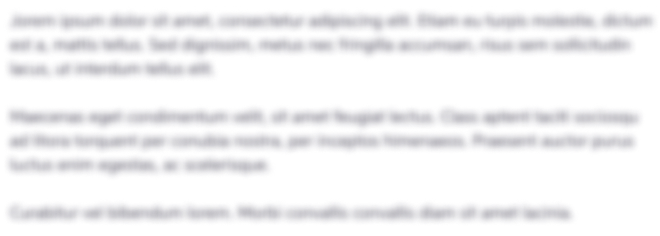
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started