Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java code please /* * Lab 07a - Exploring the String class * * * (c) 2019 Terri Davis */ public class Lab07a { public
Java code please
/* * Lab 07a - Exploring the String class * * * (c) 2019 Terri Davis */ public class Lab07a { public static void main( String[] args ) { String magicWord = "Priori Incantatem"; String anotherMagicWord = "Priori Incantatem"; String hamlet = "To be, or not to be--that is the question: " + "Whether 'tis nobler in the mind to suffer The slings and arrows of " + "outrageous fortune Or to take arms against a sea of troubles " + "And by opposing end them."; /********************************************************************************************************* * Complete the following statements/code segements to produce the required output *********************************************************************************************************/ /********************************************************************************************************* * STATEMENT ONE -- Verify String references by comparing hashcodes for each String. * * The hashcode of an object is a correlate for its storage address. If two references * refer to the EXACT SAME OBJECT, their hashcodes will be identical. Generally, if * the hashcodes of two objects are *different* those objects are discrete and separate objects. * * 1. Complete the condition for the 'if' statement. * 2. Add (to each printf statement) the necessary items to replace the two decimal value holders in * each statement. *********************************************************************************************************/ if( ....... ) System.out.printf( "%n'magicWord' and 'anotherMagicWord' refer to the SAME OBJECT!%nThere are two " + "references, but ONLY ONE String object.%n" + "The hashcode of 'magicWord': %d. The hashcode of 'anotherMagicWord': %d%n", ......., ....... ); else System.out.printf( "%n'magicWord' and 'anotherMagicWord' are two completely SEPARATE OBJECTS.%n" + "The hashcode of 'magicWord': %d. The hashcode of 'anotherMagicWord': %d%n", ......., ....... ); /********************************************************************************************************* * STATEMENT TWO -- count how many characters are in the String 'hamlet'. * * Add the necessary code to replace the decimal placeholder in the printf statement below. *********************************************************************************************************/ System.out.printf( "%nString 'hamlet' is %d characters long%n", ....... ); /********************************************************************************************************* * STATEMENT THREE -- does the String 'magicWord' contain the characters (in sequence) "cantar"? * * Add the appropriate condition for the if statement. The condition should check the String 'magicWord' * for the characters 'canta'. *********************************************************************************************************/ if ( ....... ) System.out.printf( "%nThe char sequence 'cantar' DOES exist in the String 'magicWord'.%n" ); else System.out.printf( "%nThe char sequence 'cantar' DOES NOT exist in the String 'magicWord'.%n" ); /********************************************************************************************************* * STATEMENT FOUR -- write a loop to count the occurrences of the char/letter 'o' in the String 'hamlet' * * 1. Add the condition for the while loop on line 85. The loop should continue until the entire String * 'hamlet' has been examined. * 2. Complete the assignment statement on line 87. Use a method of String class that will examine a * String object for a specific character, beginning at a specific point in the String objec, * and return an integer representing the index into the String at which the character was located. * 3. Add the condition for the if statement on line 88. What value is returned from the method in * step 2 if the character is NOT found in the String? *********************************************************************************************************/ char target ='o'; // the character we will search for int length = hamlet.length( ); // length of String object; for use in loop control int count = 0; // counter -- number of occurrences found int startNxtSrch = 0; // where to start next search 'cycle'; an INDEX into the String int foundAt = 0; // index @ which 'e' was found in this search 'cycle' while( ....... ) { foundAt = .......; if ( ....... ) { startNxtSrch = foundAt +1; count += 1; } // end positive branch else startNxtSrch = length; } // end loop System.out.printf( "%nThere are %d occurrences of '%c' in hamlet.%n", count, target ); } // end main } // end Lab07a
/////////////////////////////////////////////////////////////
/* * Lab 07b - Working with Wrapper Classes * * * (c) 2019 Terri Davis */ public class Lab07b { public static void main( String[] args ) { /* * MAKE NO CHANGES TO THE DECLARED VARIABLES. * DO NOT ADD VARIABLE DECLARATIONS */ String integerA = "6053"; String integerB = "2085"; String integerC = "5023"; String doubleA = "1365.52"; String doubleB = "152.89"; String doubleC = "98541.235"; /* * Complete the following statements to produce the required results. * * Use methods of wrapper classes Integer and Double to complete the work. * */ // Output the sum of integerA, integerB, and integerC. System.out.printf( "The sum of integerA, integerB, and integerC is: %d%n", .......... ); // Output the results of integerC - integerA. System.out.printf( "The difference of integerC less integerA is: %d%n", .......... ); // Output the results of integerA / integerB. NOTE: the output MUST include the DECIMAL PORTION of the result. // You may need to refer to work done in IS2033 as needed.... System.out.printf( "The result of dividing integerA by integerB is: %g%n%n", .......... ); // Output the sum of doubleA, doubleB, and doubleC. System.out.printf( "The sum of doubleA,doubleB, and doubleC is: %f%n", .......... ); // Output the results of doubleB - doubleC. System.out.printf( "The difference of doubleB less doubleC is: %f%n", .......... ); // Output the results of doubleB / doubleA. NOTE: the output MUST include the DECIMAL PORTION of the result. System.out.printf( "The result of dividing doubleB by doubleA is: %f%n", .......... ); } // end main } // end Lab07bDescription This lab will be a little different. You will submit two completed code modules, Lab07a and Lab07b. The first module, Lab07a, requires you to use method of the String class to complete four statements/code segments. Specific instructions are included in the provided shell code and below. Lab07b will require you to use methods of at least two wrapper classes to complete the code. Again, specific instructions are included in the code shell and below Provided to you You have been provided with the following Lab 07 Instructions The following code shells: o Labe7a.java o Labe7b.java . Expected Results Requirements Lab07a.java DO NOT change the provided variable declarations. You may, if you wish, add declarations, but you may not change those already existing in the shell Statement One Add the condition for the if" statement on line 33. The condition should return "true" when the two String objects, magicword and anotherMagicword can be assumed to be a single object, with a single storage location. It should return "False" if those String objects are separate objects, with discrete storage locations. Complete the two printf statements by adding the necessary values to replace the two decimal place holders in each statement 1. 2. Statement Two Complete the printf statement by replacing the dots with a value containing the count of characters in the String hamlet Statement Three Complete the if condition using a String method that will search the String magicWord for the given character sequence and return a boolean value indicating whether that word exists in the String or not Statement Four This portion is somewhat longer and will require some analysis in addition to a little research. Add the condition for the while loop on line 85. The loop is to continue to process until the entire String hamlet has been searched for the target character Complete the assignment statement on line 87. The value assigned to foundAt should be the index value at which the target character was located for the current pass through the loop. 1. 2. 3. Add the condition for the if statement on line 88. The condition should return "true" if an instance of the target character has been found for the current pass through the loop. If the end of the String has been reached, or there are no more instances of the target character, the condition should return "false" NOTE: You will need to be cautious here - an infinite loop will result if the loop condition, assignment, and if condition do not work together properly. There are hints embedded in the provided code Lab07b.java Complete the printf statements to provide the required output DO NOTchange any provided variable declarations. DO NOT ADD any variable declarations to this code DO NOT CHANGE the format String values for the printf statements. Use wrapper class methods to complete the printf statements. In one case, you will need to cast a result into the proper type in order for the printf statement to process correctly Submission Requirements Submit Lab07a.java and Labe7b.java zipped into a single (and single-level) compressed folder. Do not submit.class files; do not submit .javar files Expected Results Lab07a.java magichord' and 'anotherMagicWord' refer to the SAME OBJECT! There are two references, but ONLY ONE String object. The hashcode of 'magicWord' 990997307. The hashcode of 'anotherMagicWord': 990997307 String 'hamlet' is 197 characters long The char sequence 'cantar' DOES NOT exist in the String "magicWord". There are 17 occurrences of "o' in hamlet NOTE: These values will be different in your output. Lab07b.java The sum of integerA, integerB, and integerC is: 13161 The difference of integerC less integerA is: -1030 The result of dividing integerA by integerB is: 2.90312 The sum of doubleA, doubleB, and doubleC is: 100059.645000 The difference of doubleB less doubleC is: 98388.345000 The result of dividing doubleB by doubleA is: 0.111965
Step by Step Solution
There are 3 Steps involved in it
Step: 1
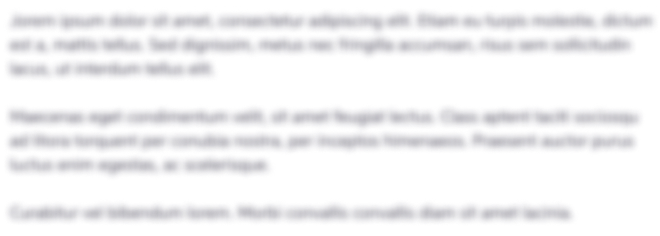
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started