Question
JAVA: Connect 4 server/client program. For the code below I am having trouble figuring out how to make a move in the connect 4 game.
JAVA: Connect 4 server/client program. For the code below I am having trouble figuring out how to make a move in the connect 4 game. Server appears, player can join, but the game board does nothing. Appreciate any help. Thanks!
*********************connect4 client**************
import java.awt.*; import java.awt.event.*; import javax.swing.*; import javax.swing.border.LineBorder; import java.io.*; import java.net.*;
public class connectfourclient extends JApplet implements Runnable, connectfourconstraints {
private boolean my_Turn = false; private char my_token = ' '; private char other_token = ' '; private Cell[][] cells = new Cell[6][7]; private JLabel jibl_Title = new JLabel(); private JLabel jibl_Status = new JLabel(); private int row_Select; private int column_Select; private DataInputStream from_server; private DataOutputStream to_server; private boolean Continuing_PLaying = true; private boolean wait = true; private boolean is_StandAlone = false; private String host = "localhost";
@Override public void init() { JPanel pn = new JPanel(); pn.setLayout(new GridLayout(6, 7, 0, 0)); for (int i = 0; i < 6; i++) { for (int j = 0; j < 7; j++) { pn.add(cells[i][j] = new Cell(i, j, cells)); } } pn.setBorder(new LineBorder(Color.black, 1)); jibl_Title.setHorizontalAlignment(JLabel.CENTER); jibl_Title.setFont(new Font("SansSerif", Font.BOLD, 16)); jibl_Title.setBorder(new LineBorder(Color.black, 1)); jibl_Status.setBorder(new LineBorder(Color.black, 1)); add(jibl_Title, BorderLayout.NORTH); add(pn, BorderLayout.CENTER); add(jibl_Status, BorderLayout.SOUTH); COnnect_To_Server(); }
private void COnnect_To_Server() { try { Socket sckt; if (is_StandAlone) { sckt = new Socket(host, 8000); } else { sckt = new Socket(getCodeBase().getHost(), 8000); } from_server = new DataInputStream(sckt.getInputStream()); to_server = new DataOutputStream(sckt.getOutputStream()); } catch (Exception ex) { System.err.println(ex); } Thread thread = new Thread(this); thread.start(); }
@Override public void run() { try { int plyer = from_server.readInt(); if (plyer == player_2) { my_token = 'r'; other_token = 'b'; jibl_Title.setText("Player 1 with color red"); jibl_Status.setText("Waiting for plyer 2 to join"); from_server.readInt(); jibl_Status.setText("Player 2 has joined. I start first"); my_Turn = true; } else if (plyer == player_2) { my_token = 'b'; other_token = 'r'; jibl_Title.setText("Player 2 with color blue"); jibl_Status.setText("Waiting for plyer 1 to move"); } while (Continuing_PLaying) { if (plyer == player_1) { wait_4_Player_Action(); sendMove(); receiveInfoFromServer(); } else if (plyer == player_2) { receiveInfoFromServer(); wait_4_Player_Action(); sendMove(); } } } catch (Exception ex) { } }
private void wait_4_Player_Action() throws InterruptedException { while (wait) { Thread.sleep(100); } wait = true; }
private void sendMove() throws IOException { to_server.writeInt(row_Select); to_server.writeInt(column_Select); }
private void receiveInfoFromServer() throws IOException { int stats = from_server.readInt(); if (stats == player_1_WON) { Continuing_PLaying = false; if (my_token == 'r') { jibl_Status.setText("I won! (red)"); } else if (my_token == 'b') { jibl_Status.setText("Player 1 (red) has won!"); receiveMove(); } } else if (stats == player_2_WON) { Continuing_PLaying = false; if (my_token == 'b') { jibl_Status.setText("I won! (blue)"); } else if (my_token == 'r') { jibl_Status.setText("Player 2 (blue) has won!"); receiveMove(); } } else if (stats == DRAW) { Continuing_PLaying = false; jibl_Status.setText("Game is over, no winner!"); if (my_token == 'b') { receiveMove(); } } else { receiveMove(); jibl_Status.setText("My turn"); my_Turn = true; } }
private void receiveMove() throws IOException { int rows = from_server.readInt(); int columns = from_server.readInt(); cells[rows][columns].setToken(other_token); }
public class Cell extends JPanel {
private int rows; private int columns; private Cell[][] cells; private char tkn = ' ';
public Cell(int rows, int columns, Cell[][] cells) { this.rows = rows; this.cells = cells; this.columns = columns; setBorder(new LineBorder(Color.black, 1)); addMouseListener(new ClickListener()); }
public char getToken() { return tkn; }
public void setToken(char c) { tkn = c; repaint(); }
@Override protected void paintComponent(Graphics g) { super.paintComponent(g);
if (tkn == 'X') { g.drawLine(10, 10, getWidth() - 10, getHeight() - 10); g.drawLine(getWidth() - 10, 10, 10, getHeight() - 10); } else if (tkn == 'O') { g.drawOval(10, 10, getWidth() - 20, getHeight() - 20); } }
private class ClickListener extends MouseAdapter {
@Override public void mouseClicked(MouseEvent e) { int r = -1; for (int x = 5; x >= 0; x--) { if (cells[x][columns].getToken() == ' ') { r = x; break; } } if ((r != -1) && my_Turn) { cells[r][columns].setToken(my_token); my_Turn = false; row_Select = r; column_Select = columns; jibl_Status.setText("Waiting for the other plyer to move"); wait = false; } } } }
public static void main(String[] args) { JFrame frame = new JFrame("Connect Four Client"); connectfourclient applet = new connectfourclient(); applet.is_StandAlone = true; if (args.length == 1) { applet.host = args[0]; } frame.getContentPane().add(applet, BorderLayout.CENTER); applet.init(); applet.start(); frame.setSize(640, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
********************Connect 4 server*****************
import java.io.*; import java.net.*; import javax.swing.*; import java.awt.*; import java.util.Date; import java.awt.event.*;
public class connectfourserver extends JFrame implements connectfourconstraints {
JButton jbtConnect; JTextField iport; JTextArea jtaLog; JLabel e;
public static void main(String[] args) { connectfourserver frame = new connectfourserver(); }
public connectfourserver() {
JTextArea jtaLog = new JTextArea();
// Create a scroll pane to hold text area JScrollPane scrollPane = new JScrollPane(jtaLog);
// Add the scroll pane to the frame add(scrollPane, BorderLayout.CENTER);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(300, 300); setTitle("Connect4 Server"); setVisible(true);
try { // Create a server socket ServerSocket serverSocket = new ServerSocket(8000); jtaLog.append(new Date() + ": Server started at socket 8000 ");
// Number a session int sessionNo = 1;
// Ready to create a session for every two players while (true) { jtaLog.append(new Date() + ": Wait for players to join session " + sessionNo + ' ');
// Connect to player 1 Socket player1 = serverSocket.accept();
jtaLog.append(new Date() + ": Player 1 joined session " + sessionNo + ' '); jtaLog.append("Player 1's IP address" + player1.getInetAddress().getHostAddress() + ' ');
// Notify that the player is Player 1 new DataOutputStream( player1.getOutputStream()).writeInt(player_1);
// Connect to player 2 Socket player2 = serverSocket.accept();
jtaLog.append(new Date() + ": Player 2 joined session " + sessionNo + ' '); jtaLog.append("Player 2's IP address" + player2.getInetAddress().getHostAddress() + ' ');
// Notify that the player is Player 2 new DataOutputStream( player2.getOutputStream()).writeInt(player_2);
// Display this session and increment session number jtaLog.append(new Date() + ": Start a thread for session " + sessionNo++ + ' ');
// Create a new thread for this session of two players HandleASession task = new HandleASession(player1, player2);
// Start the new thread new Thread(task).start(); } } catch (IOException ex) { System.err.println(ex); } } }
class HandleASession implements Runnable, connectfourconstraints {
private Socket playr1; private Socket player2; private char[][] cells = new char[6][7]; private DataInputStream fromPlayer1; private DataOutputStream toPlayer1; private DataInputStream fromPlayer2; private DataOutputStream toPlayer2; private boolean Continuing_PLaying = true;
public HandleASession(Socket playr1, Socket player2) { this.playr1 = playr1; this.player2 = player2; for (int i = 0; i < 6; i++) { for (int j = 0; j < 7; j++) { cells[i][j] = ' '; } } }
@Override public void run() { try { DataInputStream fromPlayer1 = new DataInputStream( playr1.getInputStream()); DataOutputStream to_Player1 = new DataOutputStream( playr1.getOutputStream()); DataInputStream from_player2 = new DataInputStream( player2.getInputStream()); DataOutputStream to_player2 = new DataOutputStream( player2.getOutputStream()); to_Player1.writeInt(1); while (true) { int rows = fromPlayer1.readInt(); int columns = fromPlayer1.readInt(); char tkn = 'r'; cells[rows][columns] = 'r'; if (isWon(rows, columns, tkn)) { to_Player1.writeInt(player_1_WON); to_player2.writeInt(player_1_WON); sendMove(to_player2, rows, columns); break; } else if (isFull()) { to_Player1.writeInt(DRAW); to_player2.writeInt(DRAW); sendMove(to_player2, rows, columns); break; } else { to_player2.writeInt(CONTINUE); sendMove(to_player2, rows, columns); } rows = from_player2.readInt(); columns = from_player2.readInt(); cells[rows][columns] = 'b'; if (isWon(rows, columns, tkn)) { to_Player1.writeInt(player_2_WON); to_player2.writeInt(player_2_WON); sendMove(to_Player1, rows, columns); break; } else { to_Player1.writeInt(CONTINUE); sendMove(to_Player1, rows, columns); } } } catch (IOException ex) { System.err.println(ex); } }
private void sendMove(DataOutputStream out, int rows, int columns) throws IOException { out.writeInt(rows); out.writeInt(columns); }
private boolean isFull() { for (int i = 0; i < 6; i++) { for (int j = 0; j < 7; j++) { if (cells[i][j] == ' ') { return false; } } } return true; }
private boolean isWon(int rows, int columns, char tkn) { for (int a = 0; a < 5; a++) { for (int b = 0; b < 6; b++) { System.out.print(cells[a][b]); } System.out.println(); } for (int a = 0; a < 6; a++) { for (int b = 0; b < 3; b++) { if (cells[a][b] == tkn && cells[a][b + 1] == tkn && cells[a][b + 2] == tkn && cells[a][b + 3] == tkn) { return true; } } } for (int a = 0; a < 3; a++) { for (int b = 0; b < 7; b++) { if (cells[a][b] == tkn && cells[a + 1][b] == tkn && cells[a + 2][b] == tkn && cells[a + 3][b] == tkn) { return true; } } } for (int a = 0; a < 3; a++) { for (int b = 0; b < 6; b++) { if (cells[a][b] == tkn && cells[a + 1][b + 1] == tkn && cells[a + 2][b + 2] == tkn && cells[a + 3][b + 3] == tkn) { return true; } } } for (int a = 0; a < 3; a++) { for (int b = 3; b < 7; b++) { if (cells[a][b] == tkn && cells[a + 1][b - 1] == tkn && cells[a + 2][b - 2] == tkn && cells[a + 3][b - 3] == tkn) { return true; } } } return false; } }
*************connect4 interface*************
public interface connectfourconstraints {
public static int player_1 = 1; public static int player_2 = 2; public static int player_1_WON = 1; public static int player_2_WON = 2; public static int DRAW = 3; public static int CONTINUE = 4; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
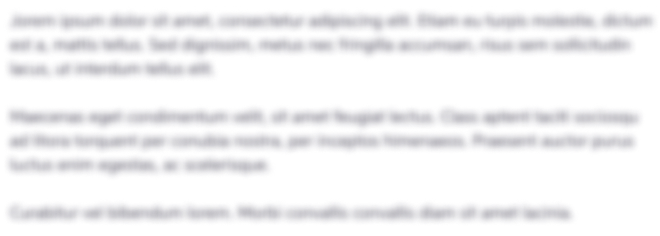
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started