Answered step by step
Verified Expert Solution
Question
1 Approved Answer
( java ) Demo - Strategy Pattern PaymentStategy Interface package StrategyPatternDemoOne; / / Strategy interface interface PaymentStrategy { void pay ( double amount ) ;
javaDemo Strategy Pattern
PaymentStategy Interface
package StrategyPatternDemoOne;
Strategy interface
interface PaymentStrategy
void paydouble amount;
package StrategyPatternDemoOne;
class PayPalStrategy implements PaymentStrategy
private String email;
private String password;
public PayPalStrategyString email, String password
this.email email;
this.password password;
public void paydouble amount
System.out.printlnPaying amount with PayPal.";
Perform the payment logic using PayPal credentials
package StrategyPatternDemoOne;
Concrete strategies
class CreditCardStrategy implements PaymentStrategy
private String cardNumber;
private String expiryDate;
private String cvv;
public CreditCardStrategyString cardNumber, String expiryDate, String cvv
this.cardNumber cardNumber;
this.expiryDate expiryDate;
this.cvv cvv;
public void paydouble amount
System.out.printlnPaying amount with credit card.";
Perform the payment logic using credit card details
package StrategyPatternDemoOne;
Client code
class ShoppingCart
private PaymentStrategy paymentStrategy;
public void setPaymentStrategyPaymentStrategy paymentStrategy
this.paymentStrategy paymentStrategy;
public void checkoutdouble amount
Perform the checkout process
paymentStrategy.payamount;
Driver:
package StrategyPatternDemoOne;
public class Driver
Usage
public static void mainString args
ShoppingCart cart new ShoppingCart;
Set the payment strategy dynamically
cart.setPaymentStrategynew CreditCardStrategy;
cart.checkout;
Change the payment strategy at runtime
cart.setPaymentStrategynew PayPalStrategyexample@example.com", "password";
cart.checkout;
Open imagepng
In the example above, the PaymentStrategy interface defines a contract for payment strategies, and CreditCardStrategy and PayPalStrategy are concrete implementations of the payment algorithm. The ShoppingCart class contains a reference to a PaymentStrategy object, which can be set dynamically. The client code can change the payment strategy by calling the setPaymentStrategy method.
By applying the Strategy pattern, the client code can switch between different payment strategies without modifying the core logic of the ShoppingCart class. This flexibility is especially useful when you have multiple algorithms or behaviors that need to be dynamically selected or replaced based on varying requirements.

Step by Step Solution
There are 3 Steps involved in it
Step: 1
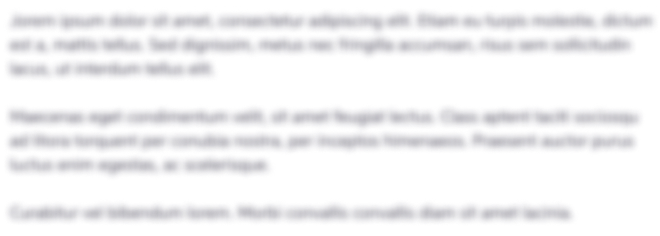
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started