Question
Java Foundations Write a class ArrayStack that implements the StackADT interface using arrays, and also defines a toString method to print out the contents of
Java Foundations
Write a class ArrayStack that implements the StackADT interface using arrays, and also defines a toString method to print out the contents of the stack.
Hint: it is helpful to write a main() method in the ArrayStack class that does a quick test to be sure it works correctly. Do some very brief testing such as creating an object, pushing a few items, printing the stack, popping the items, printing them on their way out, etc.
2. Write a class called ArrayPalindrome. ArrayPalindrome is to have the following in stance variables and methods:
(a) Three ArrayStack instance variables.
(b) The constructor will take no parameters and will create the three stacks of type ArrayStack.
(c) A public method called isPalindrome that takes a string parameter and returns a boolean. The incoming string will be checked to see if it's a palindrome. You will need to examine the characters of the string one at a time and push them onto one of the stacks. The charAt(index) method will be helpful here. Recall also the Character char wrapper class. Be sure to look at the String API for other useful methods.
3. Write a main method in ArrayPalindrome that tests several strings, both palindromes and non-palindromes. Be sure to test any boundary conditions. This is what we will evaluate to test the completeness of your lab. Do not ask for input from the user, instead select a set of test strings. Print out the string, and then "is a palindrome" or "is not a Palindrome". Test at least 5 strings.
Hint: you may want to write a clear method that removes all elements from the three stacks so you can reuse the same ArrayPalindrome object.
StackADT.java
/** * Defines the interface to a stack collection. * * @author Java Foundations * @version 4.0 */ public interface StackADT
/** * Removes and returns the top element from this stack. * * @return the element removed from the stack * @throws EmptyCollectionException if the stack is empty */ public T pop();
/** * Returns the top element of this stack without removing it from the stack. * * @return the element on top of the stack. It is not removed from the stack * @throws EmptyCollectionException if the stack is empty */ public T peek();
/** * Returns true if this stack contains no elements. * * @return true if the stack is empty, false if the stack is not empty */ public boolean isEmpty();
/** * Returns the number of elements in this stack. * * @return the number of elements in the stack */ public int size();
}
EmptyCollectionException.java
/** * Represents the situation in which a collection is empty. * * @author Java Foundations * @version 4.0 */ public class EmptyCollectionException extends RuntimeException { /** * Sets up this exception with an appropriate message. * @param collection the name of the collection */ public EmptyCollectionException(String collection) { super("The " + collection + " is empty."); } } ArrayStack.java
import java.util.Arrays;
public class ArrayStack
ArrayPallindrone.java
public class ArrayPallindrome { private ArrayStack
public static void main(String[] args) { ArrayPalindrome pal = new ArrayPalindrome(); if(pal.isPalindrome("bob")) { System.out.println("bob is a palindrome"); } else { System.out.println("bob is not a palidrone"); } // TODO Auto-generated method stub
} /** * Test the input string * @param input string to be tested for palindromity * @return true if input is palindrome */ public boolean isPalindrome(String input)
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
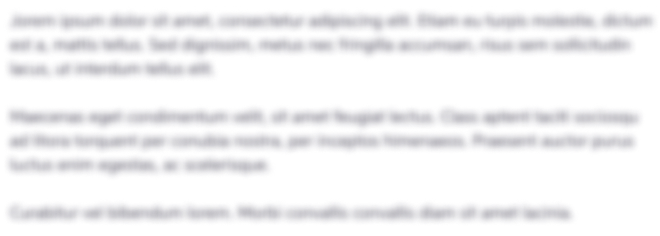
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started